Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial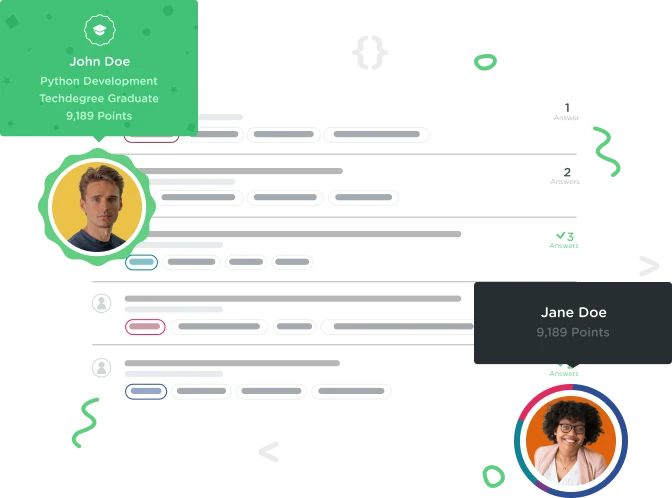
Rodrigo Chousal
16,009 PointsBlog Reader Challenge
Extra Credit Table View Sections
Create sections in your table view to categorize blog posts into Design, Development and Mobile.
Hint: You can create three separate arrays and modify the method numberOfSectionsInTableView
to return the number three. In your cellForRowAtIndexPath
method check indexPath.section
and write an if statement or a switch statement to return text from the appropriate array.
I've created three separate properties for the sections in the header file:
@property (nonatomic, strong) NSArray *mobile;
@property (nonatomic, strong) NSArray *development;
@property (nonatomic, strong) NSArray *design;
I`ve also initialised these arrays in the implementation file:
- (void)viewDidLoad
{
[super viewDidLoad];
self.mobile = [NSArray
arrayWithObjects:@"The Missing Widget in the Android SDK: SmartImageView",
@"Get Started with iOS Development", nil];
self.design = [NSArray
arrayWithObjects:@"An Interview with Shay Howe",
@"Getting a Job in Web Design and Development", nil];
self.development = [NSArray
arrayWithObjects:@"Treehouse Friends: Paul Irish",
@"Treehouse Show Episode 13 - Navicons and Framework Flights", nil];
}
And modified the numberOfSectionsInTableView
method to return the number 3.
I managed to do this as well:
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
if (section == 0) {
return [self.mobile count];
} else if (section == 1) {
return [self.design count];
} else if (section == 2) {
return [self.development count];
}
return 0;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
if (indexPath.section == 0) {
cell.textLabel.text = [self.mobile objectAtIndex:indexPath.row];
} else if (indexPath.section == 1) {
cell.textLabel.text = [self.design objectAtIndex:indexPath.row];
} else if (indexPath.section == 2) {
cell.textLabel.text = [self.development objectAtIndex:indexPath.row];
}
return cell;
}
Does anyone see what's wrong? When I run I see a screen with all of the rows and labels, but no sections...
3 Answers

Martin Granger
14,521 PointsHi Rodrigo,
I too battled with this one for a couple of days before I finally got it to work. I had to add another section (maybe there's another way, but I couldn't find it):
- (NSString *)tableView:(UITableView *)tableView titleForHeaderInSection:(NSInteger)section
{
NSString *title = nil;
if (section == 0) {
title=@"Mobile";
} else if (section == 1) {
title=@"Design";
} else if (section == 2) {
title=@"Development";
}
return title;
}
Hopefully this helps you.
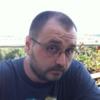
Robert Bojor
Courses Plus Student 29,439 PointsHi Rodrigo,
You will also need to implement a similar if-else statement into numberOfRowsInSection:.
Base on the section index you need to return the number of rows for that section, which is actually the number of items in your arrays.
- (NSInteger)numberOfRowsInSection:(NSInteger)section {
if (section == 0) {
return [mobile count];
} else if (section == 1) {
return [design count];
} else if (section == 2) {
return [development count];
}
return 0;
}
Rodrigo Chousal
16,009 PointsSorry! I forgot to post that part... This is what I wrote in my project:
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
if (section == 0) {
return [self.mobile count];
} else if (section == 1) {
return [self.design count];
} else if (section == 2) {
return [self.development count];
}
return 0;
}
Should be the same right?
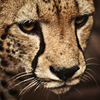
Will Macleod
Courses Plus Student 18,780 Points// TableViewController.m
// BlogReader
//
// Created by Amit Bijlani on 12/6/12.
// Copyright (c) 2012 Amit Bijlani. All rights reserved.
//
#import "TableViewController.h"
@interface TableViewController ()
@end
@implementation TableViewController
- (id)initWithStyle:(UITableViewStyle)style
{
self = [super initWithStyle:style];
if (self) {
// Custom initialization
}
return self;
}
- (void)viewDidLoad
{
[super viewDidLoad];
self.mobile = [NSArray arrayWithObjects:@"The Missing Widget in the Android SDK: SmartImageView",
@"Get started with iOS Development",
nil];
self.design = [NSArray arrayWithObjects:
@"An Interview with Shay Howe",
@"Treehouse Friends: Paul Irish",
nil];
self.development = [NSArray arrayWithObjects:
@"Getting A Job In Web Design and Development",
@"Treehouse Show Episode 13 – LLJS, Navicons and Framework Flights",
nil];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Return the number of sections.
return 3;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
if (section == 0) {
return [self.mobile count];
} else if (section == 1) {
return [self.design count];
} else if (section == 2) {
return [self.development count];
}
return 0;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
if (indexPath.section == 0) {
cell.textLabel.text = [self.mobile objectAtIndex:indexPath.row];
} else if (indexPath.section == 1) {
cell.textLabel.text = [self.design objectAtIndex:indexPath.row];
} else if (indexPath.section == 2) {
cell.textLabel.text = [self.development objectAtIndex:indexPath.row];
}
return cell;
}
- (NSString *)tableView:(UITableView *)tableView titleForHeaderInSection:(NSInteger)section
{
NSString *title = nil;
if (section == 0) {
title = @"Mobile";
} else if (section == 1) {
title = @"Design";
} else if (section == 2) {
title = @"Development";
}
return title;
}
/*
// Override to support conditional editing of the table view.
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath
{
// Return NO if you do not want the specified item to be editable.
return YES;
}
*/
/*
// Override to support editing the table view.
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath
{
if (editingStyle == UITableViewCellEditingStyleDelete) {
// Delete the row from the data source
[tableView deleteRowsAtIndexPaths:@[indexPath] withRowAnimation:UITableViewRowAnimationFade];
}
else if (editingStyle == UITableViewCellEditingStyleInsert) {
// Create a new instance of the appropriate class, insert it into the array, and add a new row to the table view
}
}
*/
/*
// Override to support rearranging the table view.
- (void)tableView:(UITableView *)tableView moveRowAtIndexPath:(NSIndexPath *)fromIndexPath toIndexPath:(NSIndexPath *)toIndexPath
{
}
*/
/*
// Override to support conditional rearranging of the table view.
- (BOOL)tableView:(UITableView *)tableView canMoveRowAtIndexPath:(NSIndexPath *)indexPath
{
// Return NO if you do not want the item to be re-orderable.
return YES;
}
*/
#pragma mark - Table view delegate
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
// Navigation logic may go here. Create and push another view controller.
/*
<#DetailViewController#> *detailViewController = [[<#DetailViewController#> alloc] initWithNibName:@"<#Nib name#>" bundle:nil];
// ...
// Pass the selected object to the new view controller.
[self.navigationController pushViewController:detailViewController animated:YES];
*/
}
@end
Rodrigo Chousal
16,009 PointsRodrigo Chousal
16,009 PointsStone Preston Help me out?