Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial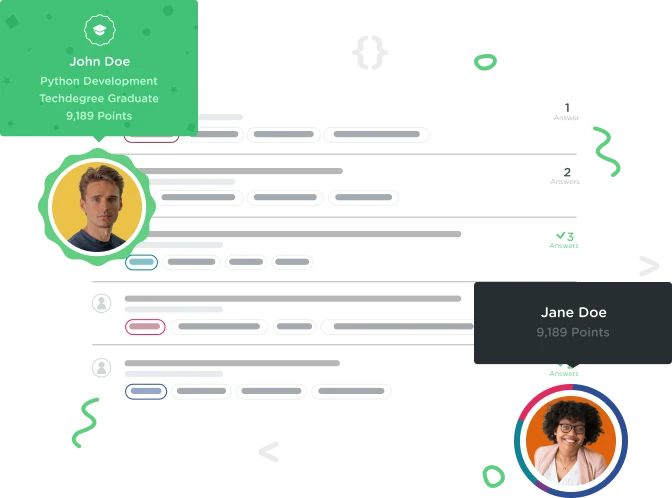
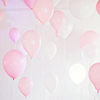
Dylan Cairns
11,191 Pointsblog reader challenge declaring intent
I can't figure out how to do this challenge: The member variable 'mUrls' is filled with a few URLs. In the 'onListItemClick()' method, declare and start an Intent that causes the URL tapped on to be viewed in the browser. Hint: The String URL needs to be set as the data for the Intent as a Uri object.
Here's what I have:
import android.os.Bundle; import android.widget.ArrayAdapter; import android.view.View; import android.view.ListView; import android.content.Intent; import android.net.Uri; public class CustomListActivity extends ListActivity {
protected String[] mUrls = { "http://www.teamtreehouse.com", "http://developer.android.com", "http://www.github.com" };
protected JSONObject mBlogData;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_custom_list);
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, mUrls);
setListAdapter(adapter);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l,v,position,id);
JSONObject jsonPosts = mBlogData.getJSONArray("posts");
JSONObject jsonPost = jsonPosts.getJSONObject(position);
Intent intent = new Intent(Intent.ACTION_VIEW);
intent.setData(Uri.parse(mUrls));
}
}
2 Answers
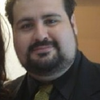
Carlos Delgado B
11,330 PointsHi there, you can't use the mUrls array for the Uri.parse method, instead of that you need to first declare a String variable to get the String value of "mUrls" for each position, for that you can do the following; "String URL = mUrls[position];" after that you need to use the newly declared variable and then you need to start the activity using a sentence, I dont see the need to write everything, I think you are stuck just because of that missing variable.
Like Dillon said, you dont need any JSON type object for this challenge
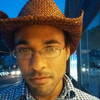
Dillon Shaw
6,400 PointsIf I remember, mUrls is a string array, so you don't need to do anything with JSON in this challenge. So instead of using the position parameter int he getJSONObject function, you would use it as the position in the mUrls array.