Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial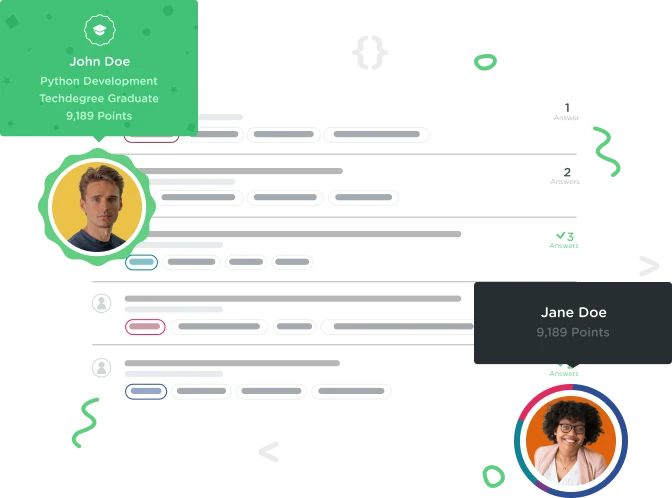
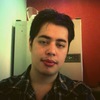
Jonathan Fernandez
8,325 PointsBlog Reader Extra Credit
Extra Credit Instead of dates display the age of a blog post. For example, few hours ago, X days ago, weeks ago, months ago.
Hi Everyone, just trying to do the extra credit for blog reader and thought I would try to start with "days ago" working. I'm trying to implement this code as shown below:
<p>
- (NSString *) dateAgo; {
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"yyyy-MM-dd HH:mm:ss"]; //format type is = 2014-07-03 11:00:35 (e.g.)
NSDate *tempDate = [dateFormatter dateFromString:self.date];
NSTimeInterval day = 60 * 60 * 24; //double
NSDate *timeDifference = [NSDate dateWithTimeInterval:day sinceDate:tempDate]; //How can I convert NSDate to if statement?
if ( ?? ) { //if timeDifference > day ???
[dateFormatter setDateFormat:@"dd"];
} else { [dateFormatter setDateFormat:@"EE MM dd"];
}
return [dateFormatter stringFromDate:tempDate];
}
</p>
Although as you can see I'm kinda stuck and am not sure how to proceed from here. Can anyone point me in the right direction? Any feedback will be greatly appreciated. : )
3 Answers
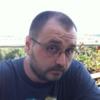
Robert Bojor
Courses Plus Student 29,439 PointsIf you want to check other similar code on "fuzzy time" you can have a look at my implementation here: https://github.com/robertBojor/postedOn
I've made it into a category so it's easier to call all the time.

Stone Preston
42,016 Pointsyou are on the right track. what you need to get is a time interval from the date of the post to now, then compare that to different values (seconds in a day for example)
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"yyyy-MM-dd HH:mm:ss"]; //format type is = 2014-07-03 11:00:35 (e.g.)
NSDate *tempDate = [dateFormatter dateFromString:self.date];
NSDate *currentDate = [NSDate date];
NSTimeInterval timeInterval = [tempDate timeIntervalSinceDate:currentDate];
//compare the interval to the number of seconds in a min
if (timeInterval <= 60) {
cell.dateLabel.text = @"1 minute ago"
} else if (timeInterval <= 3600 && timeInterval > 60) {
cell.dateLabel.text = @"1 hour ago";
}
and just keep increasing the time. notice how after the first one you have to check if its greater than one number, but less than another.
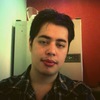
Jonathan Fernandez
8,325 PointsHi Stone,
Thanks so much for the feedback. I have tried implementing the "timeIntervalSinceDate" and it really helps me throw it in to the if statements. The only problem I have now though is that the code only executes on the first if statement.
Also I can't directly throw in the "cell.dateLabel.text = @"1 hour ago";" (Which makes it hard for me to create string data I need) That part is implementing in my man view controller as the code we are working in now is at a class to handle this. I don't want to move all this code all the way to the view controller as I think it will make it very cumbersome. What should I do? (Author and Date are sharing one text Label together as was demonstrated in the video)
<p>
//View Controller.m
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
JWFBlogPost *bloggy = [self.blogPosts objectAtIndex:indexPath.row];
if ( [bloggy.thumbnail isKindOfClass:[NSString class]] ){
NSData *imageData = [NSData dataWithContentsOfURL:bloggy.thumbnailURL];
UIImage *image = [UIImage imageWithData:imageData];
cell.imageView.image = image;
} else {
cell.imageView.image = [UIImage imageNamed:@"treehouse.png"];
}
cell.textLabel.text = bloggy.title;
cell.detailTextLabel.text = [NSString stringWithFormat:@"%@ - %@", bloggy.author, bloggy.dateAgo]; // <-- bloggy.dateAgo is where the text will be shown.
return cell;
}
//Class.m
- (NSString *) dateAgo; {
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"yyyy-MM-dd HH:mm:ss"]; //format type is = 2014-07-03 11:00:35 (e.g.)
NSDate *tempDate = [dateFormatter dateFromString:self.date];
NSLog(@"tempDate: %@", tempDate);
NSDate *today = [NSDate date];
NSTimeInterval timeInterval = [tempDate timeIntervalSinceDate:today];
//Setting up Time Intervals
double min = 60;
double hour = min * 60;
double day = hour * 24;
double week = day * 7;
double month = week * 4;
double year = month * 12;
if ( timeInterval < min) { // (However the code only executes this if statement.. Why?)
[dateFormatter setDateFormat:@"s"]; //Seconds Ago
} else if ( timeInterval < hour && timeInterval >= min) {
[dateFormatter setDateFormat:@"m"]; //Minutes Ago
} else if (timeInterval < day && timeInterval >= hour) {
[dateFormatter setDateFormat:@"k"]; //Hours Ago
} else if (timeInterval < week && timeInterval >= day) {
[dateFormatter setDateFormat:@"d"]; //Days Ago
} else if (timeInterval < month && timeInterval >= week) {
[dateFormatter setDateFormat:@"e"]; //Weeks Ago
} else if (timeInterval < year && timeInterval > month) {
[dateFormatter setDateFormat:@"M"]; //Months Ago
}
return [dateFormatter stringFromDate:tempDate];
}
</p>
Again thanks for taking the time to help someone like me : )
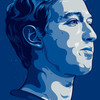
khanh tn
2,012 PointsThis is my result and my dateAgo method. Seem not like you. Source code:
-(NSString *)dateAgo {
double min = 60;
double hour = min * 60;
double day = hour * 24;
double week = day * 7;
double month = week * 4;
double year = month * 12;
NSDateFormatter *dateFormatter = [[NSDateFormatter alloc] init];
[dateFormatter setDateFormat:@"yyyy-MM-dd HH:mm:ss"];
NSDate *blogDate = [dateFormatter dateFromString:self.date];
NSDate *today = [NSDate date];
double timeAgo = fabs([blogDate timeIntervalSinceDate:today]);
NSString *timeAgoText;
if (timeAgo < min) {
timeAgoText = [NSString stringWithFormat:@"%f seconds",timeAgo];
} else if (timeAgo < hour) {
timeAgoText = [NSString stringWithFormat:@"%d minutes",(int)(timeAgo/min)];
} else if (timeAgo < day) {
timeAgoText = [NSString stringWithFormat:@"%d hours",(int)(timeAgo/hour)];
} else if (timeAgo < week) {
timeAgoText = [NSString stringWithFormat:@"%d days",(int)(timeAgo/day)];
} else if (timeAgo < month) {
timeAgoText = [NSString stringWithFormat:@"%d weeks",(int)(timeAgo/week)];
} else if (timeAgo < year) {
timeAgoText = [NSString stringWithFormat:@"%d months",(int)(timeAgo/month)];
} else {
timeAgoText = [NSString stringWithFormat:@"%d years",(int)(timeAgo/year)];
} return timeAgoText; }
// and in controller.m cell.dateLabel.text = [NSString stringWithFormat:@"%@ ago",[blog dateAgo]];
Hope can help you! :)
Jonathan Fernandez
8,325 PointsJonathan Fernandez
8,325 PointsYou.. your code is awesome.. I had made a category using your code and it made it so easy to use on the View Controller.m or Class.m. Although it doesn't feel right using it till I can build what you made from scratch. I'm gonna be studying it until I can masterfully build it like no problem.
Thank you for this reference and having the time to help someone like me. : )