Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial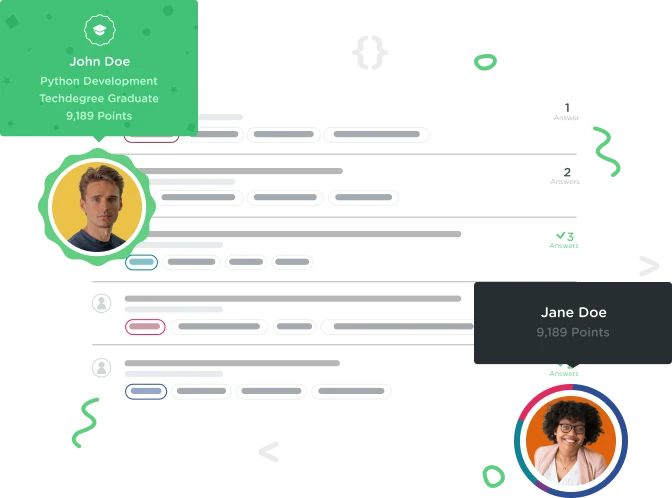

Simon K
217 PointsBlogReader error: "unable to start activity componentinfo"
I haven't completely finished all tutorials for the blogreader (I still need to watch the social sharing). My error comes when I click on a listview item and it tries to open BlogWebViewActivity. I simply get an error inside my emulator once I click on a blog post that says "Unfortunately BlogReader has stopped", strange thing is I don't get the error if I just open the file in the browser.
Here is my LogCat: ```06-18 00:08:43.560: E/AndroidRuntime(875): FATAL EXCEPTION: main 06-18 00:08:43.560: E/AndroidRuntime(875): Process: com.komlos.blogreader, PID: 875 06-18 00:08:43.560: E/AndroidRuntime(875): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.komlos.blogreader/com.komlos.blogreader.BlogWebViewActivity}: java.lang.NullPointerException 06-18 00:08:43.560: E/AndroidRuntime(875): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2195) 06-18 00:08:43.560: E/AndroidRuntime(875): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2245) 06-18 00:08:43.560: E/AndroidRuntime(875): at android.app.ActivityThread.access$800(ActivityThread.java:135) 06-18 00:08:43.560: E/AndroidRuntime(875): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1196) 06-18 00:08:43.560: E/AndroidRuntime(875): at android.os.Handler.dispatchMessage(Handler.java:102) 06-18 00:08:43.560: E/AndroidRuntime(875): at android.os.Looper.loop(Looper.java:136) 06-18 00:08:43.560: E/AndroidRuntime(875): at android.app.ActivityThread.main(ActivityThread.java:5017) 06-18 00:08:43.560: E/AndroidRuntime(875): at java.lang.reflect.Method.invokeNative(Native Method) 06-18 00:08:43.560: E/AndroidRuntime(875): at java.lang.reflect.Method.invoke(Method.java:515) 06-18 00:08:43.560: E/AndroidRuntime(875): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:779) 06-18 00:08:43.560: E/AndroidRuntime(875): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:595) 06-18 00:08:43.560: E/AndroidRuntime(875): at dalvik.system.NativeStart.main(Native Method) 06-18 00:08:43.560: E/AndroidRuntime(875): Caused by: java.lang.NullPointerException 06-18 00:08:43.560: E/AndroidRuntime(875): at com.komlos.blogreader.BlogWebViewActivity.onCreate(BlogWebViewActivity.java:21) 06-18 00:08:43.560: E/AndroidRuntime(875): at android.app.Activity.performCreate(Activity.java:5231) 06-18 00:08:43.560: E/AndroidRuntime(875): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1087) 06-18 00:08:43.560: E/AndroidRuntime(875): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2159) 06-18 00:08:43.560: E/AndroidRuntime(875): ... 11 more
and here is my BlogWebViewActivity.java
```public class BlogWebViewActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_blog_web_view);
Intent intent = getIntent();
Uri blogUri = intent.getData();
WebView webView = (WebView) findViewById(R.id.webView1);
webView.loadUrl(blogUri.toString());
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.blog_web_view, menu);
return true;
}
}
Finally, here is my webView xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="fill"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.komlos.blogreader.BlogWebViewActivity$PlaceholderFragment" >
<WebView
android:id="@+id/webView1"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_alignParentLeft="true"
android:layout_alignParentRight="true" />
</RelativeLayout>
6 Answers

Simon K
217 PointsI found out how to fix the problem. It's actually really easy.
Copy all the content from fragment_activity.xml and paste it into activity_blog.xml then delete fragment_activity.xml and it should be fixed.

João Ferreira
1,596 PointsExacly the same error that i have.. i reviewed the video and Ben creates the new activity without being prompted with the fragment name which is never created in his project, however when i create the new android activity "BlogWebViewActivity" and i guess it happens with you too, a fragment with the name "fragment_blog_web_view.xml" is created and that fragment holds the layout for the activity leaving "activity_blog_web_view.xml" with a framelayout named "container". Can this file structure be related to this demonic error? :|
I've been trying to work arround this for some hours and im not being very successfully, which leaves me a bit frustrated.
I realy hope that someone can help us out :)

ryanloerzel
12,671 PointsIn Android Studio my app is compiling and running without errors, but the only difference I'm seeing is that I'm extending Activity rather that ActionBarActivity. I'm not sure if that will make a difference. Here is my code if you want to take a look:
package com.spacecasestudios.simpleblogreader;
import com.spacecasestudios.simpleblogreader.R;
import android.app.Activity;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.webkit.WebView;
public class BlogWebViewActivity extends Activity {
protected String mUrl;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_blog_web_view);
Intent intent = getIntent();
Uri blogUri = intent.getData();
mUrl = blogUri.toString();
WebView webView = (WebView) findViewById(R.id.webView1);
webView.loadUrl(blogUri.toString());
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.blog_web_view, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int itemId = item.getItemId();
if (itemId == R.id.action_share) {
sharePost();
}
return super.onOptionsItemSelected(item);
}
private void sharePost() {
Intent shareIntent = new Intent(Intent.ACTION_SEND);
shareIntent.setType("text/plain");
shareIntent.putExtra(Intent.EXTRA_TEXT, mUrl);
startActivity(Intent.createChooser(shareIntent, getString(R.string.share_chooser_title)));
}
}

Simon K
217 PointsThat unfortunately didn't fix my error.

João Ferreira
1,596 Pointsdidint work :|

ryanloerzel
12,671 PointsWish I could be more helpful. It looks like your error is coming from line 21 in the onCreate method. Which line is that?
Do you have the following permissions set in your manifest file:
<manifest xmlns:android="http://schemas.android.com/apk/res/android" android:versionCode="1" android:versionName="1.0" package="com.spacecasestudios.simpleblogreader">
<uses-sdk android:minSdkVersion="9" android:targetSdkVersion="19"/>
//--------------------HERE-----------------------
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
//--------------------HERE-----------------------
<application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme">
<activity android:label="@string/app_name" android:name="com.spacecasestudios.simpleblogreader.MainListActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN"/>
<category android:name="android.intent.category.LAUNCHER"/>
</intent-filter>
</activity>
<activity android:label="@string/title_activity_blog_web_view" android:name="com.spacecasestudios.simpleblogreader.BlogWebViewActivity">
</activity>
</application>
</manifest>

Simon K
217 PointsYeah I already have those permissions. In my MainListActivity.java line 21 is just an import. In my BlogWebViewActivity.java line 21 is:
WebView webView = (WebView) findViewById(R.id.webView1);

ryanloerzel
12,671 PointsIt looks like
WebView webView = (WebView) findViewById(R.id.webView1);
is throwing a null pointer exception.
Here's my full activity_blog_web_view.xml
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.spacecasestudios.simpleblogreader.BlogWebViewActivity"
tools:ignore="MergeRootFrame" >
<WebView
android:id="@+id/webView1"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</FrameLayout>
If you want to check out my file structure here's my GitHub link https://github.com/ryanloerzel/SimpleBlogReaderApp
Hopefully someone else will jump in here and help solve this. Good luck!