Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial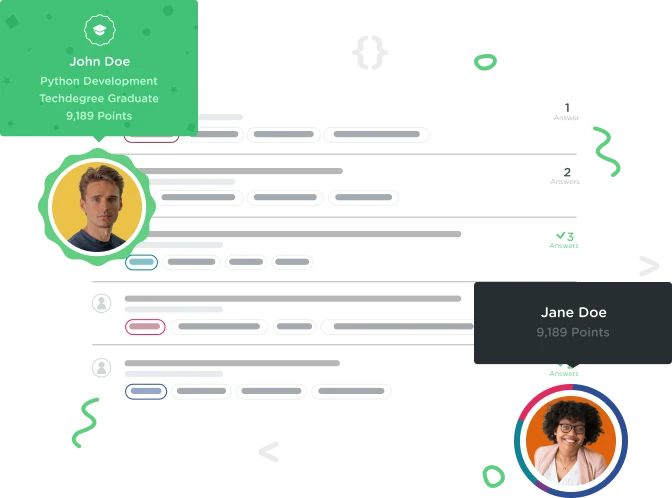

Tatenda Chimonera
4,509 PointsBoard game
I cannot pass this challenge
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
class TicTacToe(Board):
def __init__(self,width=3,height=3)
super().__init__(self,width=3,height=3)
2 Answers

William Li
7,950 PointsThe init method for the TicTacToe class should not have width and height as parameters because width and height have to always be 3.
The parameters for the super().init should not take self as a parameter because it refers to an instance of the parent class not itself.
def init(self,width=3,height=3)
By writing the code above, you are setting the default value of width and height to 3 but it can still be changed by using different parameters. You want to make it so that the width and height are always 3.
Below is how I would solve the challenge
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
class TicTacToe(Board):
def __init__(self):
width=3
height=3
super().__init__(width,height)

Josh Keenan
20,315 PointsYou have made a few little mistakes:
class TicTacToe(Board):
def __init__(self, width, height):
super().__init__(width=3, height=3)
Firstly you missed your colon at the end of the __init__
, you also don't need to pass self in when using super()
. Hope this helps, feel free to ask any questions
Tatenda Chimonera
4,509 PointsTatenda Chimonera
4,509 PointsThanks boss this helped