Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial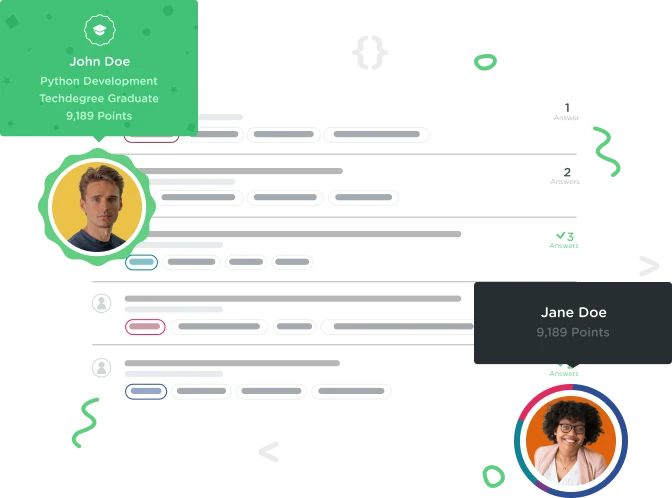
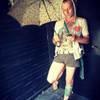
Jonathan Walker
4,240 Pointsboards.py
In the init for the subclass TicTacToe, I can't figure out why it won't set the width and height to 3. What am I doing wrong here?
Thank you
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
class TicTacToe(Board):
def __init__(self, width, height):
super().__init__(width, height)
self.width = 3
self.height = 3
5 Answers
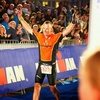
Steve Hunter
57,712 PointsHi Jonathan,
You don't want the user to be able to send a width or height to the TTT board. If you do, you certainly don't want to send them to super().__init__
.
I'd just set the values in the call to super()
and have no parameters accepted for the TTT board:
class TicTacToe(Board):
def __init__(self):
super().__init__(3, 3)
Make sense?
Steve.
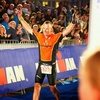
Steve Hunter
57,712 PointsHi Jonathan,
I agree with your statement the init in a subclass has to have at least all the same values as it's parent class, plus whatever new arguments are going into the subclass. The subclass must pass the super's requirements inside the super().__init__
call. But that doesn't mean these values need to be passed to the subclass init
method. The subclass can just send values as I have done - these don't have to be hard-coded as above - they can be calculated from other aspects of the class. But as long as super
gets its required parameters pass in, it'll be happy. For situations like this, where the Board size within the subclass is best not exposed to the user's error-risk, this way of approaching it is way safer!
I don't recall that particular part of this course re the Sneaky
call to super
- can you point me to it (they're also on the Linux partition of this machine; I've booted into Windows at the moment!)? You are right that those calls to super()
will end with the Object
class to handle as generic classes like Sneaky
and Agile
have Object
as their superclass.
Point me to the right part of the course and I'll have a look for you.
Steve.
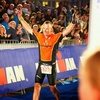
Steve Hunter
57,712 PointsThinking about this, if the class receives *args
and **kwargs
that it's going to disregard, passing to the overriding superclass, Object
makes sense as those *args
and **kwargs
may be of relevance to Object
. It means that those things can be safely disregarded if neither the subclass nor Object
know what to do with them.
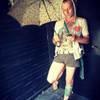
Jonathan Walker
4,240 PointsYou rock Steve! Lots of clarity :)
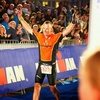
Steve Hunter
57,712 PointsNo problem!
Incidentally, I noticed you've only done Python; why is that? It's a great language but I'm always interested by students' choice of language and their reasons, so I though I'd ask.
I started with Android then moved into Java and only very recentlly moved into the beginner Python stuff. I've been hooked with Rails and done a lot of iOS stuff too. No reason for any of that, I just wandered along those routes.
Steve.
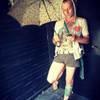
Jonathan Walker
4,240 Pointsthanks for the quick response! I've watched/read a few different videos/articles covering init and super().init, and I haven't seen anyone do what you just did in the above code. So far from what I've taken in, the init in a subclass has to have at least all the same values as it's parent class, plus whatever new arguments are going into the subclass, and then the super().init goes looking for the O.G. variables you want the parent class to take care of. Seeing you write that above is a little bit mind blowing. "I CAN DO THAT?!!?" This makes creating subclasses seem way easier and more flexible. I suppose I can blame myself for not experimenting more and figuring it out on my own, but it just seemed like the way I learned was the RULE.
I have another question about super() if you have time to answer it.
import random
class Sneaky:
sneaky = True
def __init__(self, sneaky=True, *args, **kwargs):
super().__init__(*args, **kwargs)
self.sneaky = sneaky
def hide(self, light_level):
return self.sneaky and light_level < 10
class Agile:
agile = True
def __init__(self, agile=True, *args, **kwargs):
super().__init__(*args, **kwargs)
self.agile = agile
def evade(self):
This is the code under agile.py that Kenneth wrote in the object oriented workspace. Why did he put super()init in these classes? They're not subclasses of anything right? (except of course the python object class that all objects are under) From my understanding of super(), it goes to the parent class to look for whatever arguments listed within it. If you don't have time to answer that I understand, and I'll keep looking on my own.
Thanks again!
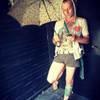
Jonathan Walker
4,240 Pointshttps://teamtreehouse.com/library/multiple-superclasses I'm not sure if it will come up for you, but under this video if I launch workspaces, and launch the workspace Kenneth set up for this part of the class, it will contain the file attributes.py, which is what I posted up there (sneaky, etc) Thank you again sooo much for responding with all this detail, I really appreciate it.
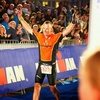
Steve Hunter
57,712 PointsYes, as in my comment above. The call to super
is just to pass on parameters that the subclass ignored to the superclass in case they're relevant there.
Object
can handle lots of things so it's possible to send some keywords into the subclass init to impact the instance created. Generally, certainly for this course, I doubt Kenneth will do that. But the options are available. I guess understanding what Object
can do will illustrate what **kwargs
can be meaningfully sent across. I suspect that's overly complicating things at this stage, though.
Steve.