Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial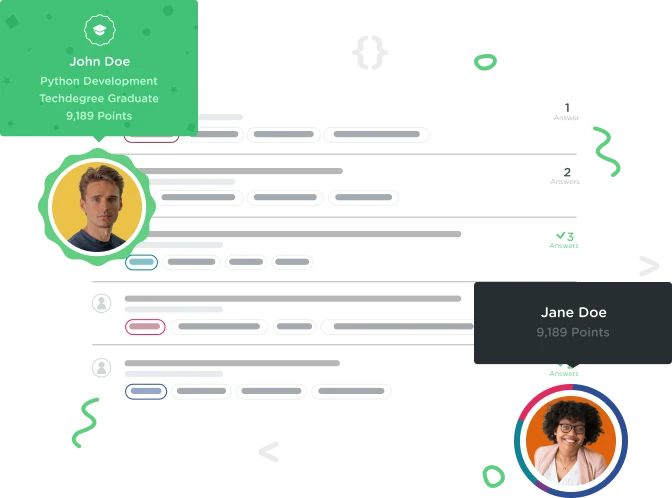
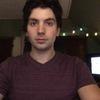
Jimmy Otis
3,228 PointsBonus: "Change your player to a dictionary with a key that holds onto where the player has been."
Been trying to work this out in my code. Help on how I might go about this?
import random
import os
def clear():
if os.name == 'nt':
os.system('cls')
else:
os.system('clear')
def map_size():
while True:
try:
map_width = int(input("How wide would you like your game map to be? "))
map_length = int(input("How long would you like your game map to be? "))
except ValueError:
clear()
print("Please enter a valid number!")
else:
CELLS = list((x, y) for y in range(map_length) for x in range(map_width))
return CELLS
def get_values():
CELLS = map_size()
player, monster, door = random.sample(CELLS, 3)
longest = max(CELLS)[0]
y_longest = max(CELLS)[1]
return player, monster, door, CELLS, longest, y_longest
def draw_map(player, monster, door, CELLS, longest):
a, b = player
c, d = monster
e, f = door
print(" _" * (longest + 1))
for cells in CELLS:
x, y = cells
if player == cells and x < longest:
if player == monster:
print("|m", end='')
elif player == door:
print("|d", end='')
else:
print("|x", end='')
elif player == cells:
if player == monster:
print("|m|")
elif player == door:
print("|d|")
else:
print('|x|')
elif x < longest:
print("|_", end='')
else:
print("|_|", "\n", end='')
move_list = {"LEFT": (-1, 0), "RIGHT": (1, 0), "UP": (0, -1), "DOWN": (0, 1)}
def game():
clear()
print("\n Welcome to the dungeon game!")
input("Press any button to continue \n")
print("Let's set up our dungeon size!")
f = get_values()
clear()
player, monster, door, CELLS, longest, y_longest = f
def player_position(move, player):
x, y = player
return (x + move_list[move][0], y + move_list[move][1])
def avaialable_moves(player):
x, y = player
all = ['LEFT', 'RIGHT', 'UP', 'DOWN']
if x == 0:
all.remove('LEFT')
if x == longest:
all.remove('RIGHT')
if y == 0:
all.remove('UP')
if y == y_longest:
all.remove('DOWN')
return all
game_loop = True
while game_loop is True:
valid_moves = avaialable_moves(player)
print("You have entered the dungeon!")
draw_map(player, monster, door, CELLS, longest)
print("You are currently in cell {}".format(player))
print("You can move {}".format(', '.join(valid_moves)))
move = input(" \n").upper()
clear()
if move in valid_moves:
player = player_position(move, player)
elif move == 'QUIT':
game_loop = False
print("See you next time")
break
else:
print("\n Ouch! Don't run into wall!")
if player == monster:
clear()
draw_map(player, monster, door, CELLS, longest)
print("AH! The monster has gotten you!")
replay = input("Game over. Press any key to play again or QUIT to exit").upper()
clear()
if replay != 'QUIT':
game()
else:
game_loop = False
print("See you next time")
break
if player == door:
clear()
draw_map(player, monster, door, CELLS, longest)
print("You escaped!")
replay = input("Congratulations. Press any key to play again or QUIT to exit").upper()
clear()
if replay != 'QUIT':
game()
else:
game_loop = False
print("See you next time")
break
game()