Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial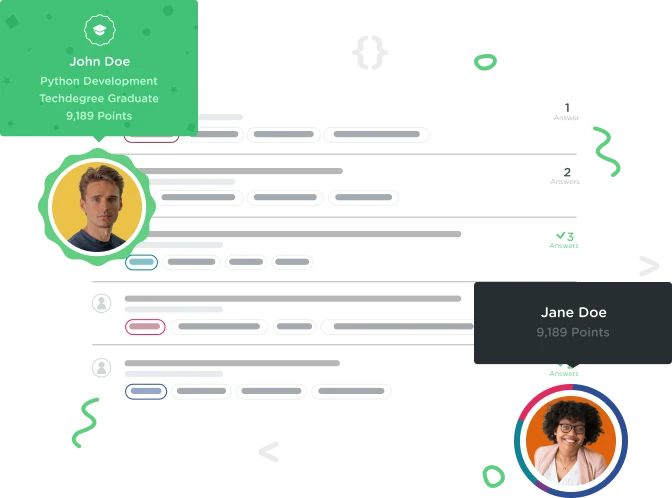

Vincent Jardel
6,436 PointsBonus : Facebook Login with email address using Parse.com and adding Users.
Hello, I've finished tracks for logging, adding friends. I would like to make a bonus : using Facebook connection and the possibility to add friends with full name of facebook. In the backend I don't have any problem, it saved everything I want. But in "FriendsViewController", when I'm connecting with FB account, or when i'm signing a new account, the view stay on the previous friends' user list. I think it's a problem with "logout" with FB account because when I stop running app and I reboot the app, it works ! In the editViewController, when I tap on a user, that add the user in parse.com, but the FriendsViewController don't refresh, so I don't know where is the real problem. And like i've said, if I stop app and reboot, that works !
Can you help me (if I'm understandable, because I'm French) :)
Here is my code :
// SignupViewController.m
#import "SignupViewController.h"
#import <Parse/Parse.h>
@interface SignupViewController ()
@end
@implementation SignupViewController
- (void)viewDidLoad
{
[super viewDidLoad];
// Do any additional setup after loading the view.
}
- (IBAction)signup:(id)sender {
NSString *password = [self.passwordField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
NSString *email = [self.emailField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
NSString *email2 = email;
NSString *name = [self.nameField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
if ([password length] == 0 || [email length] == 0)
{
UIAlertView *alertViewUsername = [[UIAlertView alloc] initWithTitle:@"Oops !" message:@"One field is empty" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil];
[alertViewUsername show];
}
else {
PFUser *newUser = [PFUser user];
newUser.password = password;
newUser.username = email;
newUser.email = email2;
newUser[@"name"] = name;
[newUser signUpInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {
if(error) {
UIAlertView *alertViewSignUp = [[UIAlertView alloc] initWithTitle:@"Sorry!" message:[error.userInfo objectForKey:@"error"] delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil];
[alertViewSignUp show];
}
else{
[self.navigationController popToRootViewControllerAnimated:YES];
}
}];
}
}
@end
// LoginViewController.m
#import "LoginViewController.h"
#import <Parse/Parse.h>
@interface LoginViewController ()
@end
@implementation LoginViewController
- (void)viewDidLoad
{
[super viewDidLoad];
self.navigationItem.hidesBackButton=YES;
}
- (IBAction)login:(id)sender {
NSString *user = [self.emailField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
NSString *password = [self.passwordField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
if ([user length] == 0 || [password length] == 0)
{
UIAlertView *alertViewUsername = [[UIAlertView alloc] initWithTitle:@"Oops !" message:@"One field is empty" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil];
[alertViewUsername show];
}
else{
[PFUser logInWithUsernameInBackground:user
password:password block:^(PFUser *user, NSError *error) {
if(error) {
UIAlertView *alertViewSignUp = [[UIAlertView alloc] initWithTitle:@"Sorry!" message:[error.userInfo objectForKey:@"error"] delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil];
[alertViewSignUp show];
}
else {
[self.navigationController popToRootViewControllerAnimated:YES];
}
}];
}
}
- (IBAction)loginButtonTouchHandler:(id)sender
{
// Set permissions required from the facebook user account
NSArray *permissionsArray = @[@"public_profile", @"email", @"user_friends"];
// Login PFUser using facebook
[PFFacebookUtils logInWithPermissions:permissionsArray block:^(PFUser *user, NSError *error) {
if (user) {
[FBRequestConnection startForMeWithCompletionHandler:^(FBRequestConnection *connection, id result, NSError *error) {
if (!error) {
[self.navigationController popToRootViewControllerAnimated:YES];
// Store the current user's Facebook ID on the user
[[PFUser currentUser] setObject:[result objectForKey:@"email"]
forKey:@"email"];
[[PFUser currentUser] setObject:[result objectForKey:@"name"]
forKey:@"name"];
[[PFUser currentUser] saveInBackground];
}
}];
}
}];
}
@end
// FriendsViewController.m
#import "FriendsViewController.h"
#import "EditFriendsViewController.h"
@interface FriendsViewController ()
@end
@implementation FriendsViewController
- (void)viewDidLoad
{
[super viewDidLoad];
self.friendsRelation = [[PFUser currentUser] objectForKey:@"friendsRelation"];
}
- (void)viewWillAppear:(BOOL)animated {
[super viewWillAppear:animated];
PFQuery *query = [self.friendsRelation query];
[query orderByAscending:@"username"];
[query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if (error) {
NSLog(@"Error %@ %@", error, [error userInfo]);
}
else {
self.friends = objects;
[self.tableView reloadData];
}
}];
}
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
if ([segue.identifier isEqualToString:@"showEditFriends"]) {
EditFriendsViewController *viewController = (EditFriendsViewController *)segue.destinationViewController;
viewController.friends = [NSMutableArray arrayWithArray:self.friends];
}
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
return [self.friends count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
PFUser *user = [self.friends objectAtIndex:indexPath.row];
cell.textLabel.text = user.username;
return cell;
}
@end```
// EditFriendsViewController.m
#import "EditFriendsViewController.h"
@interface EditFriendsViewController ()
@end
@implementation EditFriendsViewController
- (void)viewDidLoad
{
[super viewDidLoad];
PFQuery *query = [PFUser query];
[query orderByAscending:@"username"];
[query findObjectsInBackgroundWithBlock:^(NSArray *objects, NSError *error) {
if (error) {
NSLog(@"Error: %@ %@", error, [error userInfo]);
}
else {
self.allUsers = objects;
[self.tableView reloadData];
}
}];
self.currentUser = [PFUser currentUser];
}
#pragma mark - Table view data source
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Return the number of sections.
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
// Return the number of rows in the section.
return [self.allUsers count];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *CellIdentifier = @"Cell";
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
PFUser *user = [self.allUsers objectAtIndex:indexPath.row];
cell.textLabel.text = user.email;
if ([self isFriend:user]) {
cell.accessoryType = UITableViewCellAccessoryCheckmark;
}
else {
cell.accessoryType = UITableViewCellAccessoryNone;
}
return cell;
}
#pragma mark - Table view delegate
- (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath
{
[self.tableView deselectRowAtIndexPath:indexPath animated:NO];
UITableViewCell *cell = [tableView cellForRowAtIndexPath:indexPath];
PFRelation *friendsRelation = [self.currentUser relationforKey:@"friendsRelation"];
PFUser *user = [self.allUsers objectAtIndex:indexPath.row];
if ([self isFriend:user]) {
cell.accessoryType = UITableViewCellAccessoryNone;
for(PFUser *friend in self.friends) {
if ([friend.objectId isEqualToString:user.objectId]) {
[self.friends removeObject:friend];
break;
}
}
[friendsRelation removeObject:user];
}
else {
cell.accessoryType = UITableViewCellAccessoryCheckmark;
[self.friends addObject:user];
[friendsRelation addObject:user];
}
[self.currentUser saveInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {
if (error) {
NSLog(@"Error: %@ %@", error, [error userInfo]);
}
}];
}
#pragma mark - Helper methods
- (BOOL)isFriend:(PFUser *)user {
for(PFUser *friend in self.friends) {
if ([friend.objectId isEqualToString:user.objectId]) {
return YES;
}
}
return NO;
}
@end```

Guillaume Maka
Courses Plus Student 10,224 Points«But when the new user is signing up, it appear the previous friends' user, I need just this help ! :)»
Could you explain in more details !
PS: You can send me an email (french/english) 134508@supinfo.com, if it's more easier for you !
Vincent Jardel
6,436 PointsVincent Jardel
6,436 PointsThe error begin when I create a user for the first time, or change user. If i'm connecting for the twice on the user, it's ok. But if I change user, that appear the friends' user list of the previous user.
Thanks for helping !
I've found the mistake : add self.friendsRelation = [[PFUser currentUser] objectForKey:@"friendsRelation"]; in viewWillAppear method, before PFQuery ;)
But when the new user is signing up, it appear the previous friends' user, I need just this help ! :)