Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial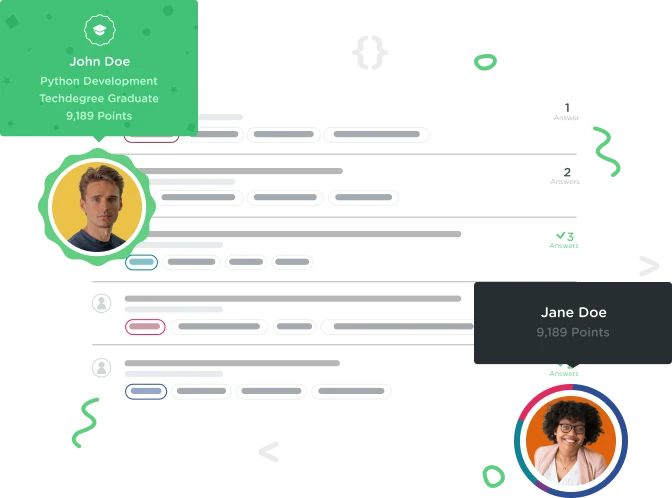
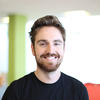
Kristian Woods
23,414 PointsBook title and Description aren't being inserted into database and redirect isn't working, either
I'm following along to the video, but i'm using my own database. So I have to change a few things to fit my needs.
I'm not getting any error messages, telling me that the connection to the database was unsuccessful, though. However, nothing is being inserted into the database. Plus the redirect isn't working
My connection:
<?php
try {
$db = new PDO('mysql:host=127.0.0.1;dbname=music_online', 'root', 'root');
$db->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
} catch ( \Exception $e ) {
echo 'Error connecting to the Database: ' . $e->getMessage();
exit;
}
The function:
<?php
/**
* @return \Symfony\Component\HttpFoundation\Request
*/
function request() {
return \Symfony\Component\HttpFoundation\Request::createFromGlobals();
}
function addMusic($title, $description) {
global $db;
$ownerId = 0;
try {
$query = 'INSERT INTO books (name, description, owner_id) VALUES(:name, :description, :ownerId)';
$stmt = $db->prepare($query);
$stmt = $db->bindParam(':name', $title);
$stmt = $db->bindParam(':description', $description);
$stmt = $db->bindParam(':ownerId', $ownerId);
return $stmt->execute();
} catch (\Exception $e) {
throw $e;
}
}
<?php
require_once __DIR__ . '/../inc/bootstrap.php';
$bookTitle = request()->get('title');
$bookDescription = request()->get('description');
try {
$newBook = addMusic($bookTitle, $bookDescription);
$response = \Symfony\Component\HttpFoundation\Response::create(null, \Symfony\Component\HttpFoundation\Response::HTTP_FOUND, ['Location' => '/books.php']);
$response->send();
exit;
} catch (\Exception $e){
$response = \Symfony\Component\HttpFoundation\Response::create(null, \Symfony\Component\HttpFoundation\Response::HTTP_FOUND, ['Location' => '/add.php'] );
$response->send();
exit;
}
<?php
require_once __DIR__ . '/inc/head.php';
require_once __DIR__ . '/inc/nav.php';
?>
<div class="container">
<div class="well">
<h2>Add a Music Record</h2>
<form class="form-horizontal" method="post" action="procedures/addMusic.php">
<?php include __DIR__ . '/inc/addMusicRecord.php'; ?>
</form>
</div>
</div>
<?php
require_once __DIR__ . '/inc/footer.php';
After I click the button to add the record, I get redirected to the addMusic.php page. However, I'm supposed to get redirected to the books.php page
any help would be great
1 Answer

Simon Coates
28,694 PointsMy guess:
$stmt = $db->bindParam(':description', $description);
might be
$stmt->bindParam(":description", $description);
(this would apply to the other uses of bindParam also).
Is your environment set up to show you error messages? Or do you have a debug log to store these? If your code is running but sending you to the add.php page, then helpful information may be present on the exception object $e.
Miguel Rivera
21,679 PointsMiguel Rivera
21,679 PointsI have the same problem