Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial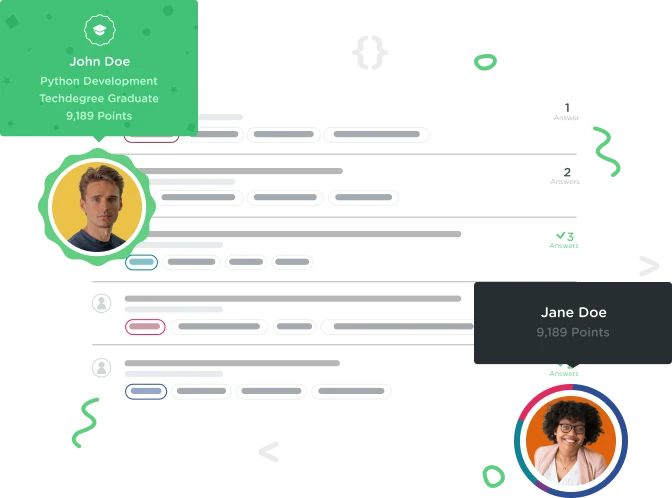
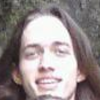
Andrew Gay
20,893 PointsBookings in Ruby on Rails
Alright I have scaffold setup that allows us to add more products on our page but was wondering if someone could help me out with setting up a booking system for the products (AS this is a rental server we cannot rent out 1 item to two people at the same time)
I really just need the idea on how to go about doing it best. (And help with how to setup the database to store and request that info)
We currently use devise for authentication and stripe for payments (Though you probably don't need either of this information perhaps you could show me how to attach a bookings data to the devise model so I can sort through all the users that have booked something to verify if someone can book on that day etc.)
I do not need the math done, I just need help setting up the database and possible how to setup the form
(I honestly could figure it out on my own, but I know for a fact it would not be the correct way to do it in Rails)
Thank you very much for you help! If you need to see the source code please go to my github
If you have suggestions on my code please shoot away (I know a lot of it is junk and rushed, I planned on going back and fixing it but I honestly need to start adhering to writing good code once and not forcing myself to rush and go back and fix)
1 Answer
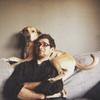
Joshua Shroy
9,943 PointsHey Andrew,
My first impression is that this is an app that would allow a customer to rent out a bike. Would they be renting anything else? Will you be selling other products and/or bikes?
Since Bikes are a focal object within your app. I suggest creating a Bike model. If you intend to sell Bikes too, they could inherit from Product. I recommend the Active Record Acts-As gem. A Product will be "actable". A Bike will act_as a Product. Other products such as tires, chains, handlebars, gloves, could be divided up to different tables (models) depending on your needs - all inheriting from Product. Maybe have one for "Apparel" and another for "Parts".
For renting/booking, I suggest creating a Booking table. I assume the relationship between Bikes and User would be a many-to-many so you could use Booking as your "through" table.
class User < ActiveRecord::Base
has_many :bikes, through: :bookings
has_many :bookings
end
class Booking < ActiveRecord::Base
belongs_to :user
belongs_to :bike
end
class Bike < ActiveRecord::Base
has_many :users, through: :bookings
has_many :bookings
end
I assume you would need a way to account for the date and times a bike would be rented out, take a look at the ice_cube gem. There is a lot of details that will depend on your needs but I hope this give you a good kick-start in the right direction. Let me know if I could clear anything up for you.