Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial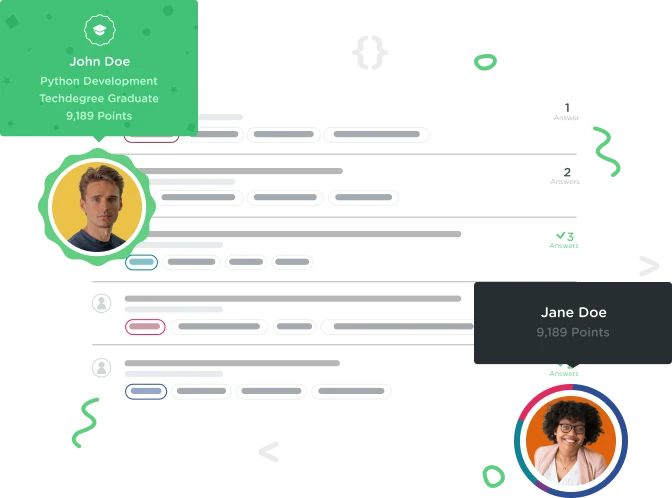
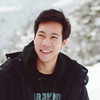
Howie Yeo
Courses Plus Student 3,639 PointsBoolean value always False + countdown feature not working
Hi i need some help to debug, currently, (1) the boolean value does not turn to TRUE even though i input the exact value to the questions. (2) The countdown feature is not working, it is always saying "5 questions left"... Please help to review and solve my problem :)
// Assume all answer are wrong
var question1 = false;
var question2 = false;
var question3 = false;
var question4 = false;
var question5 = false;
// To add countdown feature for remaining question
var countdown = 5;
var questionsLeft = ' [' + countdown + ' questions left]';
// To ask player the question and collect input
var guess = prompt('What is color of apple?' + questionsLeft);
if ( guess.toUpperCase() === 'RED') {
questions1 = true;
}
countdown -= 1;
var guessTwo = prompt('What is color of sky?' + questionsLeft);
if ( guessTwo.toUpperCase() === 'BLUE') {
questions2 = true;
}
countdown -= 1;
var guessThree = prompt('What is color of grass?' + questionsLeft);
if ( guessThree.toUpperCase() === 'GREEN') {
questions3 = true;
}
countdown -= 1;
var guessFour = prompt('What is color of soil?' + questionsLeft);
if ( guessFour.toUpperCase() === 'BROWN') {
questions4 = true;
}
countdown -= 1;
var guessFive = prompt('What is color of cloud?' + questionsLeft);
if ( guessFive.toUpperCase() === 'WHITE') {
questions = true;
}
//Send alert to end player input.
alert('Thanks for playing the quiz, press OK to view your results.');
/*
Based on the true value of each question to determine:
1. The score
2. print the result
*/
var score = 0;
if (question1) {
score += 1;
document.write('<p>Question 1 was correct!</p>');
} else {
document.write('<p>Question 1 was incorrect! It should be Red</p>');
}
if (question2) {
score += 1;
document.write('<p>Question 2 was correct!</p>');
} else {
document.write('<p>Question 2 was incorrect! It should be Blue</p>');
}
if (question3) {
score += 1;
document.write('<p>Question 3 was correct!</p>');
} else {
document.write('<p>Question 3 was incorrect! It should be Green</p>');
}
if (question4) {
score += 1;
document.write('<p>Question 4 was correct!</p>');
} else {
document.write('<p>Question 4 was incorrect! It should be Brown</p>');
}
if (question5) {
score += 1;
document.write('<p>Question 5 was correct!</p>');
} else {
document.write('<p>Question 5 was incorrect! It should be Red</p>');
}
//output rank
if ( score === 5 ) {
document.write("<p><strong>You earned a gold crown!</strong></p>");
} else if ( score >= 3 ) {
document.write("<p><strong>You earned a silver crown!</strong></p>");
} else if ( score >= 1 ) {
document.write("<p><strong>You earned a bronze crown!</strong></p>");
} else {
document.write("<p>Sorry, nothing for you</p>");
}
1 Answer
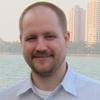
Ryan Field
Courses Plus Student 21,242 PointsHi, Howie. Looks like you've got a simple copy+paste error. You've got variables like question1
, but below you have them as questions1
with an s.
As for why you countdown statement isn't working, it's because you created the variable questionsLeft
once, inside which you use the countdown
variable. When you update a variable, it doesn't update inside another variable that was previously created. That is to say, the moment you construct your questionsLeft
string, it no longer uses the countdown
variable, and is simply hard-coded as [5 questions left]
. To do what you're wanting to do, you need to update questionsLeft
before you use it each time, something like this:
countdown -= 1;
questionsLeft = ' [' + countdown + ' questions left]';
var guessTwo = prompt('What is color of sky?' + questionsLeft);
if ( guessTwo.toUpperCase() === 'BLUE') {
questions2 = true;
}
There are cleaner ways to do this, such as using a function, but you get the main idea.