Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial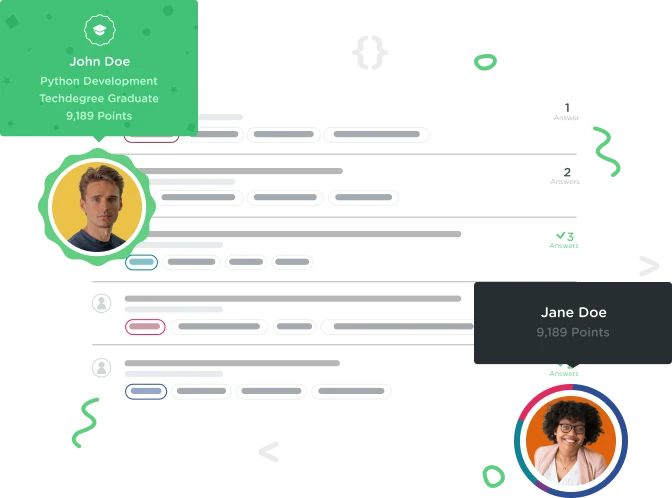

Leonardo Escalante
3,190 PointsBoolean value in a class
I really appreciate your help in this topic, my code doesn' t work:
class TodoList attr_reader :name, :todo_items
def initialize(name) @name = name @todo_items = [] end
def add_item(name) todo_items.push(TodoItem.new(name)) end
def empty? index = 0 found = false todo_items.each do |todo_item| if todo_item.name == name found = true end if found break else index += 1 end end if found todo_items.delete_at(index) return true else return false end end if found todo_items.delete_at(index) return true else return false end
end
end
Please tell me what I'm doing bad.
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def empty?
index = 0
found = false
todo_items.each do |todo_item|
if todo_item.name == name
found = true
end
if found
break
else
index += 1
end
end
if found
todo_items.delete_at(index)
return true
else
return false
end
end
if found
todo_items.delete_at(index)
return true
else
return false
end
end
end
1 Answer
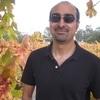
Kourosh Raeen
23,733 PointsThe only thing the empty? method needs to do is to check whether @todo_items array is empty or not:
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def empty?
if @todo_items.empty?
return true
else
return false
end
end
end