Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial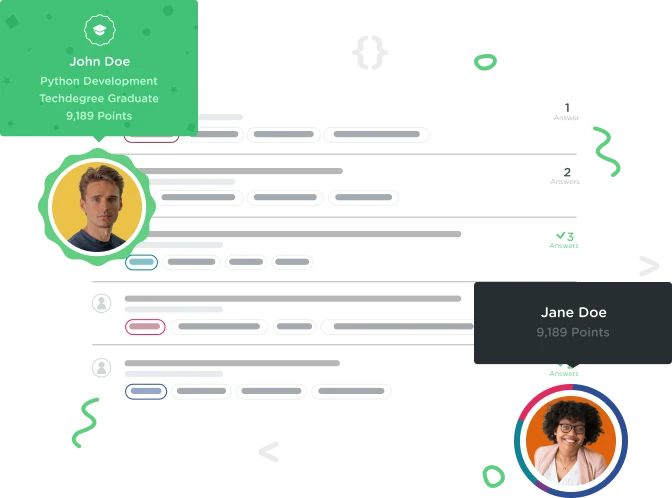
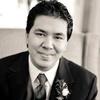
Thomas Helms
16,816 Points<bound method> output
So I've built my combat.py, and did from combat import Combat in my monster.py. But when I go to create hoggle = Goblin() and then do hoggle.attack, I get <bound method Combat.attack of <monster.Goblin object at 0x7fd572733fd0>> instead of the expected output. Where'd I go wrong?
combat.py
import random
class Combat:
dodge_limit = 6
attack_limit = 6
def dodge(self):
roll = random.randint(1,self.dodge_limit)
return roll > 4 # Nice! This returns only if the result is greater than 4
def attack(self):
roll = random.randint(1,self.attack_limit)
return roll > 4
monster.py
import random
from combat import Combat
COLORS = ['yellow','red','blue','green','orange','black']
class Monster(Combat): # object is only used if you need to use the code in Python 2 and 3
min_hit_points = 1
max_hit_points = 1
min_experience = 1
max_experience = 1
weapon = 'sword'
sound = 'roar'
def __init__(self,**kwargs):
self.hit_points = random.randint(self.min_hit_points,self.max_hit_points)
self.experience = random.randint(self.min_experience,self.max_experience)
self.color = random.choice(COLORS)
for key, value in kwargs.items():
setattr(self, key, value) # what we want to set the attr on, the attr we want to set, the value of the attr
def __str__(self):
return '{} {}, HP: {}, XP: {}'.format(self.color.title(),
self.__class__.__name__,
self.hit_points,
self.experience)
def battlecry(self):
return self.sound.upper()
class Goblin(Monster): # this is saying that Goblin is a subclass of Monster
#pass (tell python to keep going like nothing's happened)
max_hit_points = 3
max_experience = 2
sound = 'squeak'
class Troll(Monster):
min_hit_points = 3
max_hit_points = 5
min_experience = 2
max_experience = 6
sound = 'growl'
class Dragon(Monster):
min_hit_points = 5
max_hit_points = 10
min_experience = 6
max_experience = 10
sound = 'roar'
2 Answers
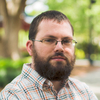
Kenneth Love
Treehouse Guest Teacherattack
is a method so you have to call it, like attack()
.
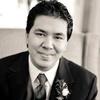
Thomas Helms
16,816 PointsReally? Really? Is it that simple? (checks then facepalms)
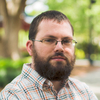
Kenneth Love
Treehouse Guest Teacherit's always the smallest things that bring us down