Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial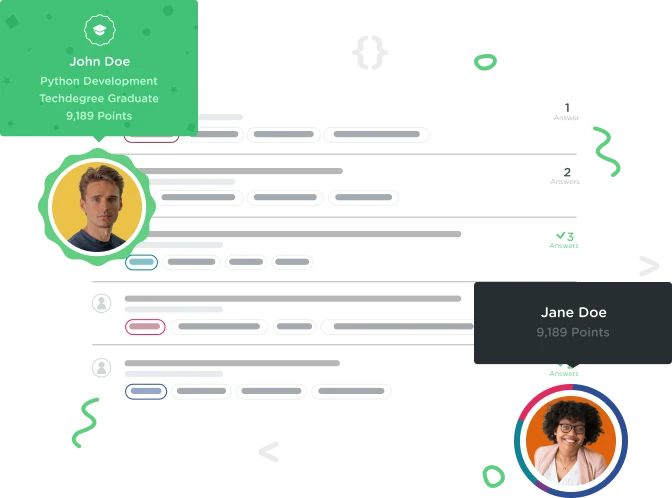

Manuel Aviles
4,995 PointsBreaking down a For Loop
I have recently started the JavaScript course and it is a bit difficult for me. I do not seem to understand For Loops that well. If someone could kindly break down a For Loop it would be much appreciated! It could also help some other people that need help too :)
2 Answers

Alexander La Bianca
15,959 PointsHey! So a For Loop is great for cases when you want to loop through a list of 'known' size. A list of usernames for example. Let's say that list of usernames is stored in an array:
var usernames = ['user1','user2','user3','user4','user5']
Now let's say you want to alert each username in the array to the browser...for whatever reason. You could do something like:
alert(usernames[0]) //alerts user1
alert(usernames[1]) //alerts user2
alert(usernames[2]) //alerts user3
alert(usernames[3]) //alerts user4
alert(usernames[4]) //alerts user5
Doing it like that is a pain though...Imagine you had a list of 100 users.. That is when the For Loop is very useful
for(var i = 0; i<5;i++) {
alert(usernames[i];
}
//BREAKDOWN OF THE FOR LOOP
//1. var i = 0 --> this is telling the for loop to start the counter at 0 and store the counter in a variable called i
// It could be anything. It could be var counter = 2. In that case your counter starts at 2 and stores the //counter in a variable called counter
//2. i<5 --> This is telling the for loop when to stop counting. In this case at 4 as it is the last value that is smaller than 5
//You can even do something like this i<usernames.length (usernames.length is the length of the array which is
//5)
//3. i++ --> This is telling the for loop to increase the counter by 1 every time the for loops body is completed
//4. alert(usernames[i]) --> This is the body of the for loop. Here you write your logic that you want to execute each time //the for loop iterates. So in this case we want to alert the username at each index. So the first time the loop goes //through, usernames[i] will be usernames[0]. The next iteration will be usernames[1] etc.

Rhys Kearns
4,976 Pointsfor(var i = 0; i < 10; i++) {
console.log("Each time it loops the number increases! Look: " + i)
}
So here what happens is you declare a variable named i, this is no difference than me writing
var number;
Then aslong as i is LESS than 10 it will keep running. Then each time it runs it will increment i by 1 - thats what i++ does. Each time is does all these 3 steps and i is STILL less than 10 it will log out what is in the for loop
Manuel Aviles
4,995 PointsManuel Aviles
4,995 PointsI appreciate the help! Thanks alot, it makes more sense now
Nick Trabue
Courses Plus Student 12,666 PointsNick Trabue
Courses Plus Student 12,666 PointsYou actually don't have to know the size if you're looping through an array.
array.length will count the number of items in an array. This is good if the size of your array could grow or shrink.
var usernames = ['user1','user2','user3','user4','user5']