Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial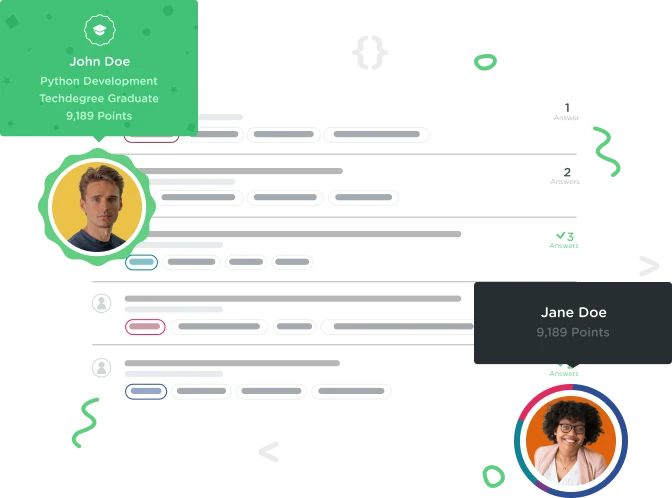

Viktor Johansson
3,523 PointsBreaking out of two loops
Everything is finished but I have to add code for the user to be able to quit from any of the inputs. However, I can't break out of the loop as it would just break the innermost loop and continue looping inputs from the outer loop. Possibly I could make a function for this and use a return statement, but as it hasn't been introduced in the course yet, I'm trying to avoid it to figure out another solution. Here's my code:
using System;
namespace Arithmetic
{
class MainClass
{
public static void Main (string[] args)
{
var number1 = 0.0;
var number2 = 0.0;
var result = 0.0;
var operation = "";
// keep everything running until user inputs quit
while (true) {
// Keep prompting until user inputs a valid number
while (true) {
Console.Write ("Please enter your first number or \"quit\": ");
var str = Console.ReadLine ();
if (str == "quit")
// do something
try {
number1 = double.Parse (str);
break;
} catch (FormatException) {
Console.WriteLine ("Invalid number try again!");
continue;
}
}
// Keep prompting until user inputs a valid operation
while (true) {
Console.Write ("What arithmetic operation would you like to do? +-/*^ : ");
operation = Console.ReadLine ();
if ((operation == "+") || (operation == "-") || (operation == "^") ||
(operation == "/") || (operation == "*")) {
break;
} else if (operation == "quit")
// do something
else
continue;
}
// Keep prompting until user inputs a valid number
while (true) {
Console.Write ("Please enter your second number: ");
var str = Console.ReadLine();
if (str == "quit")
// do something
try {
number2 = double.Parse (str);
break;
} catch (FormatException) {
Console.WriteLine ("Invalid number try again!");
continue;
}
}
// do arithmetic calculations
if (operation == "+")
result = number1 + number2;
else if (operation == "-")
result = number1 - number2;
else if (operation == "/")
result = number1 / number2;
else if (operation == "*")
result = number1 * number2;
else if (operation == "^")
result = Math.Pow (number1, number2);
Console.WriteLine ("Your result is: " + result);
}
}
}
}
1 Answer

Viktor Johansson
3,523 PointsI decided to go with System.Environment.Exit.. Does anyone know if that is good or bad practise ?
lonedo
24,500 Pointslonedo
24,500 Points