Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial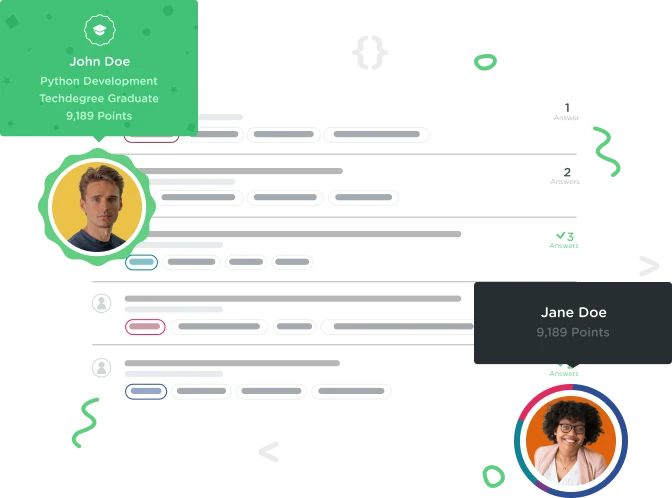

Lauren Boyes
2,516 PointsBringing in information from a PHP array without using a foreach() loop.
Hi there,
Need a bit of help. I'm writing my own bit of code at the moment. I am trying to pull just one bit of data from an array, held in a function.php file. I am assuming as it is just one bit of information I should not be using a foreach() loop but I am unsure what to use instead.
Here is some of my code from the index.php file (I want to change the text in the <p> tag to be read in from the array in the functions.php file:
<?php include("functions.php"); ?>
<body>
<div id= "question"><p>IN GERMAN - WHAT DOES THE WORD "SAMMELN" MEAN?</p></div>
</body>
And code from my functions.php file:
<?php
function get_question($question_id, $question) {
$output = "";
$output = $output . "<p>" . $question["question"] . "</p>";
return $output;
}
$questions = array();
$questions[1]= array(
"question" => 'IN GERMAN - WHAT DOES THE WORD "SAMMELN" MEAN?',
"hint-one" => "JOHN LIEBT ES, BRIEFMARKEN SAMMELN",
"hint-two" => "TO ACCUMULATE AS A HOBBY OR FOR STUDY",
"answer-one" => "TARGET",
"answer-two" => "COLLECT",
"answer-three" => "SAMPLE",
"correct" => "answer-two"
)
?>
I know that this is probably a very easy thing to accomplish but I think I am set in my C++ ways and can't quite get it right.
Thanks in advance!
2 Answers

Lee Chavers
22,506 PointsOkay, this code is working for me
// your html page
<?php
include("functions.php");
$question = get_question($questions[0]);
?>
<body>
<div id="question"><p><?php echo $question; ?></p></div>
</body>
and this would be your functions.php file
<?php
function get_question($question) {
$output = "";
$output = $output . "<p>" . $question["question"] . "</p>";
return $output;
}
$questions = array();
$questions[]= array(
"question" => 'IN GERMAN - WHAT DOES THE WORD "SAMMELN" MEAN?',
"hint-one" => "JOHN LIEBT ES, BRIEFMARKEN SAMMELN",
"hint-two" => "TO ACCUMULATE AS A HOBBY OR FOR STUDY",
"answer-one" => "TARGET",
"answer-two" => "COLLECT",
"answer-three" => "SAMPLE",
"correct" => "answer-two"
);
?>
I fixed a couple issues you had with your code. On your html/php page you would call your function with the specific part of the array that you want ($question = get_question($questions[0]);). After that we can call our $question variable which uses your function to just get the question from your array.
On your functions.php I removed the hard-coded $questions[1] and replaced it with $questions[]. PHP will automatically add keys to your array as you add more in the future. Another thing that I changed is what variables you're passing to the function. Since we are passing which value from the array we need you only need this to get your question get_question($question);
Let me know if you have any questions on how my code works. If you like I can also give you a forward-looking example of when you have multiple questions and how you could use a foreach() statement to go through all your questions and still reference your function as you have it set up.

Lee Chavers
22,506 PointsYou should be able to access your information via this correction in your code
$output = $output . "<p>" . $question[0]["question"] . "</p>";

Lee Chavers
22,506 PointsArrays in php start with 0. So, I'm not sure in your example if you're just trying to grab information from the first instance of your array or the second. If it's the later you would just change the 0 in my example above to 1.

Lauren Boyes
2,516 PointsOk, that makes much more sense for the function. Thank you.
I'm more unsure about what to do in the file that calls the function.
- I am unsure if the parameters I am using are correct or even necessary
- If I should be declaring these parameters before I call the function
- How to call the function without using a foreach loop
I have been sitting here for hours trying many different combinations and having no luck. :/
Lauren Boyes
2,516 PointsLauren Boyes
2,516 PointsYou know that moment where after you've been staring at something far too long and then someone comes along and tells you the most obvious of things you've done wrong and it finally clicks?
I feel incredibly stupid but oh so very happy at the same time.
You have completely made my night and saved my sanity.
Thank you!
Lee Chavers
22,506 PointsLee Chavers
22,506 PointsI understand completely. I'm glad that I could help point you in the right direction.