Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial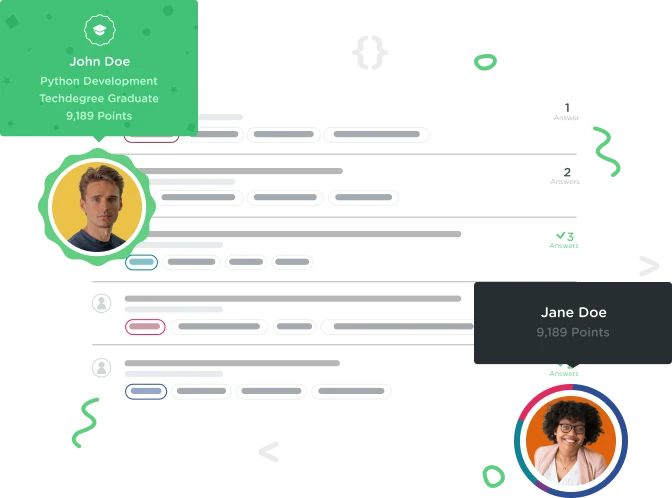
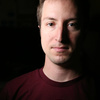
Yohanan Braun-Feder
6,101 Pointsbroken code, no errors reported...
ok, so up until a certain point, the app did what it was supposed to, then started to break - items added with the add button had no visible label and the edit and delete buttons on the new items were not showing. when i navigate using the console navigator to where the buttons are and click the edit button i can finally see the text box with the text i entered, but as soon as I finish editing the label disappears. the console logs no errors and syntax appears to be in order as far as i can tell... obviously i missed a step. anyone care to help?
// Problem: User Interaction doesnt provide desired results.
// Solution: Add interactivity so the user can manage daily tasks.
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; // first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks");
var completedTasksHolder = document.getElementById("completed-tasks");
//New Task Li
var createNewTaskElement = function(taskString) {
// create list item
var listItem = document.createElement("li");
//input (checkbox)
var checkBox = document.createElement("input"); //checkbox
//label
var label = document.createElement("label");
//input (text)
var editInput = document.createElement("input"); //text
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//Each element needs modifying and appending
checkBox.type = "checkbox";
editInput.type = "text";
editButton.textContent = "Edit";
editButton.className = "edit";
deleteButton.textContent = "Delete";
deleteButton.className = "delete";
label.innerText = taskString;
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
// add a new task
var addTask = function () {
console.log("add task...");
//create a new list item with the text from #new-task
var listItem = createNewTaskElement(taskInput.value);
// append li to incompleteTasksHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
taskInput = "";
}
// edit existing task
var editTask = function () {
console.log("edit task...");
var listItem = this.parentNode;
var editInput = listItem.querySelector("input[type=text]");
var label = listItem.querySelector("label");
var containsClass = listItem.classList.contains("editMode");
//if the class of the parent is .editMode
if ( containsClass ) {
//switch from .editMode
//label text become the input's value
label.textContent = editInput.value;
} else {
//switch to .editMode
//input value becomes the label's text
editInput.value = label.textContent;
}
//Toggle .editMode on the li
listItem.classList.toggle("editMode");
}
// delete existing task
var deleteTask = function () {
console.log("delete task...");
var listItem = this.parentNode;
var ul =listItem.parentNode;
//remove the parent li from the ul
ul.removeChild(listItem);
}
//mark task as complete
var taskCompleted = function () {
console.log("task Complete...");
//append the task li to the #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//mark task as incomplete
var taskIncomplete = function () {
console.log("task Incomplete...");
//append the task li to the #incomplete-tasks
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function (taskListItem, checkBoxEventHandler) {
console.log("bind li events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler the checkbox
checkBox.onchange = checkBoxEventHandler;
}
//set the click handler to the addtask function
addButton.onclick = addTask;
//cycle over incompleteTasksHolder ul list items
for ( i = 0; i < incompleteTasksHolder.children.length; i++ ){
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//cycle over completedTasksHolder ul list items
for ( i = 0; i < completedTasksHolder.children.length; i++ ){
//bind events to list item's children (taskInomplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
Chris Johnson
15,048 PointsChris Johnson
15,048 PointsI had a similar issue where I couldn't find out what was wrong with my code. I was using Firefox and it would not work at all. it ended up working in IE and Chrome. When i checked out the teacher notes code it also did not work in Firefox for me but worked in IE and chrome as well.