Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial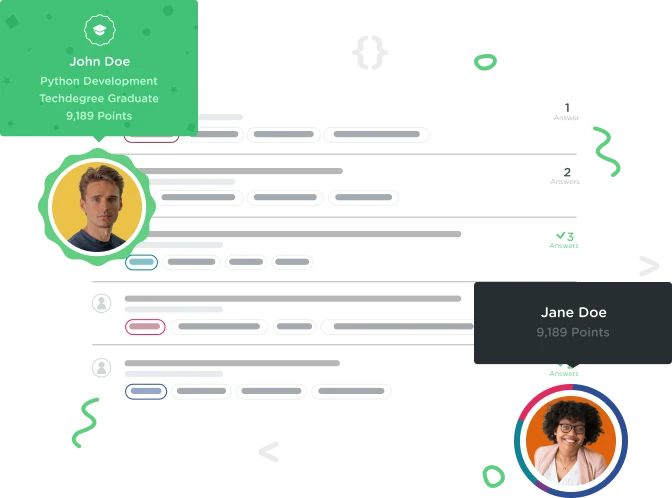
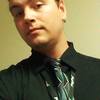
Marshal Currier
6,416 PointsBrowser Compatibility Issues (works in Chrome only)
I am working on building a more intense quiz than what was in the tutorials. I have buttons and everything is working fine in Chrome, but I am having issues with a function being undefined in Firefox and I have no idea why it isnt working in IE. Anyone able to lend a hand?
<script>
var correctAnswers =0;
var randomItem;
var selected;
var selectedQuestion;
var testing = "this is a test of testing";
var questionCheckResult = false;
var displayedQuestion = '';
var divID =0;
var questionBox = 'questionBox0';
var originalArrayLength;
var answersArray =[];
var questionArray=[[
['What do you do at a red light?'],
['Drive fast so you dont get the red light.',0],
['Stop',1],
['Run over the guy in the crosswalk', 0],
['Bump Taylor Swift till you feel better',0]],
[['Can you pass in a schoul zone?'],
['Yes.',0],
['No',1],
['Run them kids over!', 0],
['Remember back to the good ole days.',0]
],
[['How fast do you drive on the freeway?'],
['There\'s a limit?',0],
['65 Miles Per Hour',1],
['42', 0],
['Faster than the guy behind you.',0]
]
]
var callQuestion = function(number){
displayedQuestion = '';
displayedQuestion +='<div id="questionBox'+divID+'" class="questionBox">';
displayedQuestion += '<center>'+questionArray.length+' Questions Left</center>';
if (questionCheckResult){
displayedQuestion +='<div class="questionHeader">'+questionCheckResult+'</div>';
}
displayedQuestion +='<div class="questionHeader">'+questionArray[number][0]+'</div>';
for(var i2 =1;i2<=4;i2+=1){
selectedQuestion = i2;
answersArray.push('<button type="button" class="btn btn-info" value="testing" id="answer" onclick="questionCheck(randomItem, '+selectedQuestion+')">'+questionArray[number][i2][0]+'</button><br>');
}
shuffle(answersArray);
for(var i2 =0;i2<=3;i2+=1){
displayedQuestion += answersArray[i2];
}
answersArray = [];
displayedQuestion +='</div>';
document.write(displayedQuestion);
}
function shuffle(array) {
var currentIndex = array.length, temporaryValue, randomIndex ;
while (0 !== currentIndex) {
randomIndex = Math.floor(Math.random() * currentIndex);
currentIndex -= 1;
temporaryValue = array[currentIndex];
array[currentIndex] = array[randomIndex];
array[randomIndex] = temporaryValue;
}
return array;
}
var randomQuestion = function(){
var randomArrayItem = [Math.floor(Math.random() * questionArray.length)];
return randomArrayItem;
}
var questionCheck = function(array, clickedAnswer){
questionBox = "questionBox'+divID+'";
if(divID > 0){
removeQuestionBox();
}
divID += 1;
callHeaders();
console.log(array, clickedAnswer);
if (questionArray[array][clickedAnswer][1] == 1){
questionArray.splice([array], 1 );
questionCheckResult = '<font color="green">Your Answer Was Correct</font>';
}else{
questionCheckResult = '<font color="red">Your Answer Was Incorrect</font>';
}
if (questionArray.length > 0){
randomItem = randomQuestion();
callQuestion(randomItem);
}else{
document.write('<center>There are no questions left!</center>');
}
}
var callHeaders = function(){
document.write('<link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.4/css/bootstrap.min.css" rel="stylesheet"><style>.questionBox{margin:10px; background: #b6fcd5; border: solid #8dcd91 2px; padding:10px; max-width:340px;min-width:340px; border-radius: 5px; margin-right:auto;margin-left:auto;}button{margin:10px; max-width:300px;min-width:300px;}.questionHeader{text-align: center;font-weight: bold; padding-bottom: 5px;}</style>');
}
var removeQuestionBox = function(){
var elem = document.getElementById('questionBox'+divID);
elem.parentNode.removeChild(elem);
}
originalArrayLength = questionArray.length;
callHeaders();
randomItem = randomQuestion();
document.write(questionArray.length);
document.write(randomItem);
callQuestion(randomItem);
</script>