Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial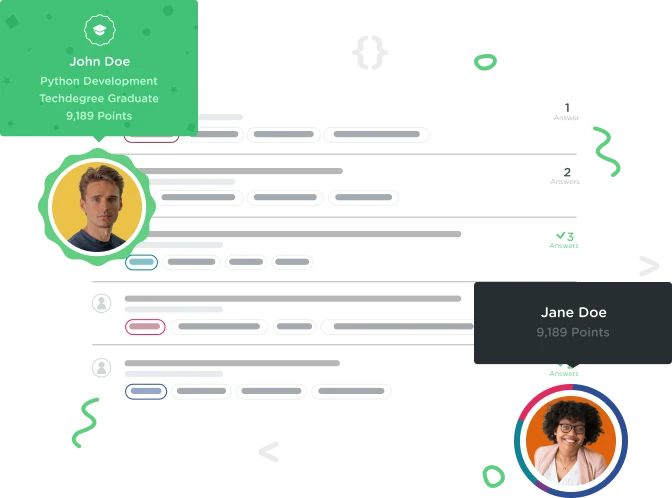

Moe Machef
Courses Plus Student 5,514 PointsBrowser Output "Cannot read property '0' of undefined"
I Keep Getting "Cannot read property '0' of undefined", I Doubled Checked My Code
app.js
var express = require('express');
var path = require('path');
var favicon = require('serve-favicon');
var logger = require('morgan');
var cookieParser = require('cookie-parser');
var bodyParser = require('body-parser');
var mongoose = require('mongoose');
var passport = require('passport');
var GitHubStrategy = require('passport-github').Strategy;
var session = require('express-session');
var MongoStore = require('connect-mongo')(session);
var User = require("./models/user");
//Configure Github Strategy
passport.use(new GitHubStrategy({
clientID : process.env.GITHUB_CLIENT_ID,
clientSecret : process.env.GITHUB_CLIENT_SECRET,
callbackURL : "http://localhost:3000/auth/github/return"
}, function(accessToken, refreshToken, profile, done){
if(profile.emails[0]){
User.findOneAndUpdate({
email : profile.emails[0].value
}, {
name : profile.displayName || profile.username,
email : profile.emails[0].value,
photo : profile.photos[0].value
}, {
upsert : true
},
done);
} else {
var noEmailError = new Error("Your email privacy settings prevent you from signing into bookworm!");
done(noEmailError, null);
}
}));
passport.serializeUser(function(user, done){
done(null, user._id);
});
passport.deserializeUser(function(userId, done){
User.findById(userId, done);
});
var routes = require('./routes/index');
var auth = require('./routes/auth');
var app = express();
// view engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'pug');
// uncomment after placing your favicon in /public
//app.use(favicon(path.join(__dirname, 'public', 'favicon.ico')));
app.use(logger('dev'));
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({ extended: false }));
app.use(cookieParser());
app.use(express.static(path.join(__dirname, 'public')));
// mongodb connection
mongoose.connect("mongodb://localhost:27017/bookworm-oauth");
var db = mongoose.connection;
//Session config for Passport and MongoDB
var sessionOptions = {
secret : 'this is a super secret dadada',
resave : true,
saveUninitialized : true,
store : new MongoStore({
mongooseConnection : db
})
};
app.use(session(sessionOptions));
//Initialize Passport
app.use(passport.initialize());
//Restore Session
app.use(passport.session());
// mongo error
db.on('error', console.error.bind(console, 'connection error:'));
app.use('/', routes);
app.use('/auth', auth);
// catch 404 and forward to error handler
app.use(function(req, res, next) {
var err = new Error('Not Found');
err.status = 404;
next(err);
});
// error handlers
// development error handler
// will print stacktrace
if (app.get('env') === 'development') {
app.use(function(err, req, res, next) {
res.status(err.status || 500);
res.render('error', {
message: err.message,
error: err
});
});
}
// production error handler
// no stacktraces leaked to user
app.use(function(err, req, res, next) {
res.status(err.status || 500);
res.render('error', {
message: err.message,
error: {}
});
});
module.exports = app;
auth.js
var express = require('express');
var router = express.Router();
var passport = require('passport');
//GET /auth/login/github
router.get('/login/github', passport.authenticate('github'));
//GET /auth/github/return
router.get('/github/return', passport.authenticate('github', {failureRedirect: '/'}),
function(req, res){
//Success Auth, redirect to profile page
res.redirect('profile');
});
//GET /auth/logout
router.get('/logout', function(req, res){
req.logout();
req.redirect('/');
});
module.exports = router;

Vitaly Khe
7,160 PointsI have exactly same issue. btw, even don't have an idea where to find an issue. First, i added console.log of user that is being passed to profile in req. It shows nothing...
Maybe github returns 500 - so no data binded to user? Here the node log. Pls check last string.
Visit http://localhost:3000 in your web browser.
(node:447) DeprecationWarning: collection.ensureIndex is deprecated. Use createIndexes instead.
GET /login 304 700.967 ms - -
GET /stylesheets/style.css 304 4.340 ms - -
GET /images/bg.jpg 304 0.533 ms - -
GET /auth/login/github 302 286.503 ms - 0
GET /stylesheets/style.css 304 0.565 ms - -
GET /images/bg.jpg 304 0.577 ms - -
GET /auth/github/return?code=d696e7d639c312bcbf42 500 1330.391 ms - 1906
1 Answer

Vitaly Khe
7,160 Pointsthe issue caused by GitHub settings. User's email on github should have been set as public.
Fix here: https://github.com/settings/profile
Zack Lee
Courses Plus Student 17,662 PointsZack Lee
Courses Plus Student 17,662 Pointswhat line is the error being thrown? there were a couple instances where you used profile.emails[0] and profile.photos[0], but I could not find where these values were instantiated.