Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial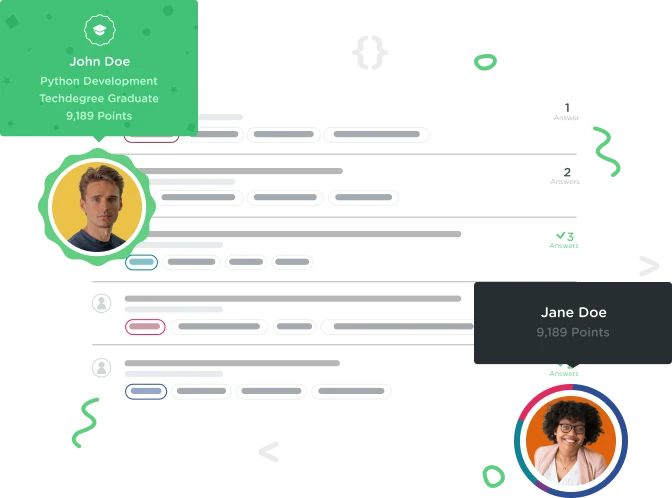

Tanuj Wadhi
869 PointsBug in code, C programming
include <stdio.h>
typedef struct { char * first; char * last; } name;
int main() { name user;
printf("First name:");
scanf("%s",user.first);
printf("Last name:");
scanf("%s",user.last);
printf("%s %s\n", user.first, user.last);
}
My code is stopping after in input my first name, and last name. It breaks with error "Thread 1:EXC_BAD_ACCESS (code=2, address=07xfff5fc0105e)
Could you tell me my error? Thanks in advance. P.S- New to coding.
1 Answer
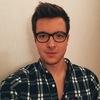
Pierre Thalamy
4,494 PointsHi Tanuj,
EXC_BAD_ACCESS
means that there is some bug in the memory management of your program. It is trying to write at a memory address that has not been initialised.
This is exactly what is happening in your program, the members of the user struct first and last are char pointers, and currently, as they have not been allocated, they both point to NULL (@0x0).
It is not a valid address, and thus the program cannot write your input there, it would return a segmentation fault, which is exactly what is happening.
Here is a reviewed version of your program that should work:
#include <stdio.h>
#include <stdlib.h>
typedef struct {
char * first;
char * last;
} name;
int main() {
name user;
// Allocate a char pointer for both first and last name strings
// It tells your program where to store the store your inputs.
user.first = malloc(sizeof(char *));
user.last = malloc(sizeof(char *));
printf("First name:");
scanf("%s", user.first);
printf("Last name:");
scanf("%s",user.last);
printf("%s %s\n", user.first, user.last);
}
I can explain further if you need it.