Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial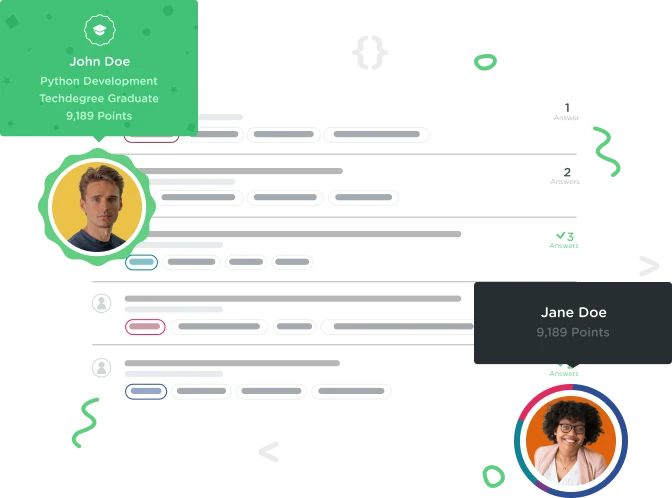
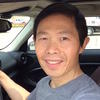
David Lin
35,864 PointsBug in Conditional Logic in Video
There's a bug in the video.
The conditional in the original if statement should be OR (||), and not AND (&&).
The original statement is:
if firstChoice == nil && secondChoice == nil {
if firstChoice == nil {
firstChoice = (title, page)
} else {
secondChoice = (title, page)
}
}
However, if both choices are nil, then only the first sub-condition will always be exercised, while the second sub-condition will never be, which is clearly not the intention.
The teacher even says, "if both the choices are nil, then we can check if the first choice is nil"... which doesn't make logical sense: of course it's nil, since both are.
The corrected logic is:
if firstChoice == nil || secondChoice == nil {
if firstChoice == nil {
firstChoice = (title, page)
} else {
secondChoice = (title, page)
}
}
This corrected version makes more sense, because then either the firstChoice or secondChoice can be nil, and both sub-conditions can be potentially exercised.
I understand that the above was not the final implementation the teacher used in the method, but I just wanted to point out the logical error.
2 Answers

Sean Fillmore
12,458 Points// This line checks to see if each of these variables are nil, otherwise at lease one has a value and returns page
if firstChoice == nil && secondChoice == nil {
//this is a nested if statement used to assign a value to one of the empty variables
if firstChoice == nil {
firstChoice = (title, page)
} else { // this else statement is nested inside of the initial if statement and is used to assign the tuple to secondChoice if firstChoice has a value
secondChoice = (title, page)
}
}
I hope this makes sense
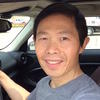
David Lin
35,864 PointsSean,
My point is that if BOTH firstChoice AND secondChoice are nil, that is, the top condition as written is satisfied, then inside the condition block, only the first sub-condition (checking that firstChoice is nil) will ALWAYS be satisfied, and the "else" clause will NEVER be executed.
Rather, the sub-conditions become relevant only if the top condition was if EITHER firstChoice OR secondChoice were nil. Then it makes sense to check if firstChoice was nil or not in the sub-conditions, since the top condition (if using OR) does not guarantee firstChoice is nil.
Dave

Sean Fillmore
12,458 PointsAfter reading your explanation and re-watching the video I see what you are saying. How could the else clause be possible if we already checked and both are nil.
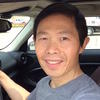
David Lin
35,864 PointsExactly! :-)
Abdulwahab Alansari
15,151 PointsAbdulwahab Alansari
15,151 PointsI was wondering about the same thing, at least I know I am not the only one :)