Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial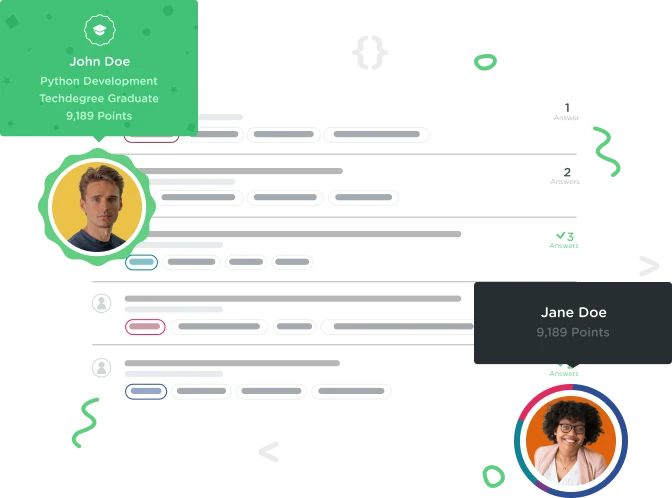

Daniel Cunningham
21,109 PointsBug in Validation Challenge!
The validation challenge asks you to fill in the appropriate code that will throw an error when member fields do not start with a lower case 'm' and the second letter in the field name must be upper-cased to ensure camel-casing. The first test is relatively straight-forward in that you need to parse the first character of the string and check if it is a lower case 'm'. However, depending on how your approach the second test, you could set yourself up for a lot more work! <br> <br> The validation is tested with several field name combinations, one of which is "m_first_name". This test must fail in order to complete the challenge. <br> <br> The common and easiest way to pass the second test is to check if the second character is upper cased using isUpperCase() in an if statement. This WOULD cause 'm_first_name' to fail because the underscore character is not a letter and therefore cannot be present in upper-case... however, if the field name was 'm_First_name' (where the second letter is, in fact, upper-cased), the field name would ALSO fail. In the end, the test passes, but for the wrong reasons. <br> <br> I want to bring this up as a potential bug because a student that attempts to solve using an "!isLowerCase" logic on a conditional statement would find that 'm _ first _ name' passes the test. If you open java-repl and test isUpperCase() and isLowerCase() on '_', you will find that they both fail. This creates a whole new challenge for anyone that uses !isLowerCase() as your if statement will allow "m_first_name" to pass. <br> <br>
For myself, I had to create a whole new function that would find the second letter in a string and THEN perform a test to ensure that the letter is, in fact, upper-cased. This would then allow an input like "m _ First _ name" or even "m_____First _ name" to pass. <br> <br> I think that it might be a very valuable to adjust the code to test whether 'm_First_name' passes. That way, students can make sure their code is passing for the right reasons. Or, as another option, challenge students to find the second letter in a string before having them produce the validation test. This 'extra work' I had to do was actually a very good lesson. Hope that helps!
2 Answers

Craig Dennis
Treehouse TeacherUnderscores aren't allowed to pass the challenge, did you get the test to pass? The second character is _
in your examples.

Jerry Kong
5,672 PointsFor 2nd condition I combined isUpperCase from the Character class with charAt() method, so:
Character.isUppercase(fieldname.charAt(1))
I am wondering how did you do it? I know there are multiple ways to solve this problem, which is really cool.

Daniel Cunningham
21,109 Pointspublic static int findSecondLetter(String fieldName) { <br> int location = 1; <br> boolean isLetter = false; <br> while (!isLetter) { <br> if (Character.isLetter(fieldName.charAt(location))){ <br> isLetter = true; <br> } else { <br> location +=1; <br> isLetter=false; <br> } <br> }<br> return location; <br> } <br> <br>
forgive the terrible formatting.. I can't figure out how to post this as code... But that finds the second letter.

Jerry Kong
5,672 PointsOkay so to add code, just use ``` at start & end (TIL).
Thanks for the write up bud.
public static int findSecondLetter(String fieldName) { int location = 1;
boolean isLetter = false;
while (!isLetter) {
if (Character.isLetter(fieldName.charAt(location))){
isLetter = true;
} else {
location +=1;
isLetter=false;
}
}
return location;
}
Daniel Cunningham
21,109 PointsDaniel Cunningham
21,109 PointsThis code, alone, passes the test:<br>
if ((fieldName.charAt(0) == 'm') && Character.isUpperCase(fieldName.charAt(1))){ return fieldName; }else{ throw new IllegalArgumentException ("This does not work"); }
in the case of fieldName.charAt(1) when fieldName = "m_first_name", it is failing because the test for uppercase and lower case on an underscore is a fail. It's not failing because the 'f' is lower cased. <br> <br>
The way to test it is to change the code to" !Character.isLowerCase(fieldName.charAt(1)))" as this would turn the false into a true and incorrectly pass the "m_first_name" field name.
Daniel Cunningham
21,109 PointsDaniel Cunningham
21,109 PointsHere's my full code to test this...
public class TeacherAssistant {
public static int findSecondLetter(String fieldName) { int location = 1; boolean isLetter = false; while (!isLetter) { if (Character.isLetter(fieldName.charAt(location))){ isLetter = true; } else { location +=1; isLetter=false; } } return location;
}
public static String validatedFieldName(String fieldName) { char letter1 = fieldName.charAt(0); char letter2 = fieldName.charAt(findSecondLetter(fieldName));
}
}
apologies for the awful formatting... again, posting code is a bit of a challenge for me :-(
Daniel Cunningham
21,109 PointsDaniel Cunningham
21,109 PointsSorry Craig, I may have misunderstood your response. I took a bit of a literal interpretation on the test in reading that the test must pass if the second letter is upper cased. The solution isUppercase() does the job in passing the test, however taking an alternative route of !isLowercase() would fail the test because it would turn an underscore character into a "true" in the same way an upper case character would. When your test of "m_first_name" incorrectly passed the test, i thought that your intention was to be able to capture the second real letter rather than fail "all other" characters vs an upper cased letter. Hopefully that makes sense. Thanks for your response!
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherOh...hmmm...sorry no I meant 2nd character. I can update that text. Sorry for the confusion!
Daniel Cunningham
21,109 PointsDaniel Cunningham
21,109 PointsIt's not a problem, it was a great learning moment. I just wanted to bring that nuance up in case that was the intent. Thank you for making the course, it's been great to work on!