Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial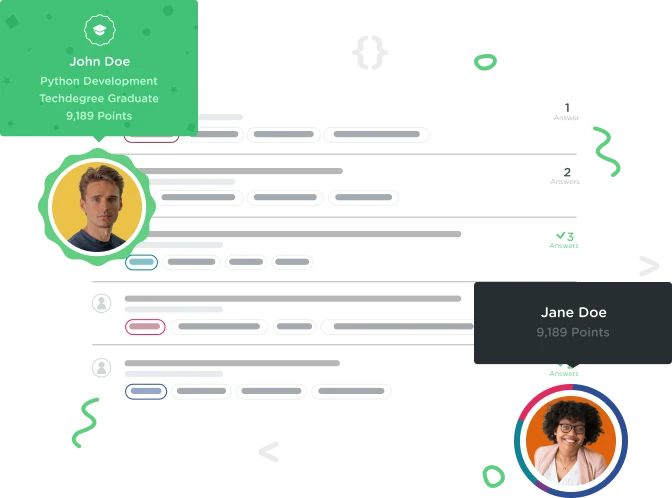

Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsBuild a Quiz Challenge, Part 1 Solution
Here is my solution to the above problem:
const quiz = [
[ "How many states are there in USA?" , 50],
[ "How many legs does an insect have?" , 6],
[ "How many continents are there?" , 7],
[ "How many centuries does Tendulkar have" , 100]
]
function print(message) {
document.write(message);
}
function quizGame( array ){
var correctAnswerNumber = 0;
var rightAnswer = "<ol>";
var wrongAnswer = "<ol>";
for( var i = 0 ; i < array.length ; i++){
var answer = prompt(array[i][0]);
answer = parseInt(answer);
if( answer == array[i][1]){
correctAnswerNumber += 1;
rightAnswer += '<li>' + array[i][0] + '</li>';
}
else{
wrongAnswer += '<li>' + array[i][0] + '</li>';
}
}
rightAnswer += '</ol>';
wrongAnswer += '</ol>';
print("You got " + correctAnswerNumber + " questions correct.\n");
print("<p>You got these questions correc:\n</p>");
print( rightAnswer );
print("<p>You got these questions wrong:</p>");
print( wrongAnswer );
}
quizGame(quiz);
Please suggestion any improvement I can make to optimize my code . Please review :) Thanks
1 Answer
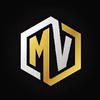
maikelvreugdenhil
1,762 PointsHi Aakash,
I noticed you used ES5 markup in your file because of the "const" you used. But you didn't apply it in other areas of your code.
You can improve your ES5 markup code by exchanging your var's that will have their values changing with let's wherever this is possible.(hint let is block scope if you happen to change it in your for loop make sure you check out your loop when accessing the array's ). For the other variables that should not change their value use const.
You can also rewrite your functions to arrow syntax. I can already see one that can be on 1 line. Also the function that can be on one line is using an outdated method for writing to the documnt. (*hint think about being specific to what area you want to print your message out to. There is a different document.method look for Document Object Properties and Methods)
Also try making objects out of your quiz array. There is a lesson in a course here on Treehouse on how you can have an const array of objects and still be able to push a new object to it. This will make your Quiz more flexible for let's say you want to be able to add more quiz objects with a question and a response to your quiz array of objects :).
Finally i'd say try an use template literals to make some of that string concatenation in your code easier to read.
If none of this makes sense, try and follow the ES2015 workshops here on Treehouse.
Cheers from one new js programmer to another! :)