Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial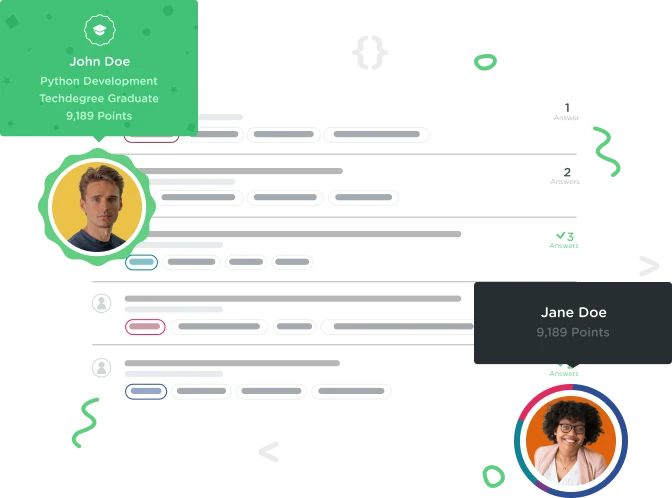
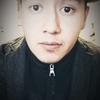
Nelson J
7,411 PointsBuild a Quiz Challenge, Part 1 Solution FEEDBACK/Help.
I think I did pretty good with the quiz except that there is one problem. When questions are wrong, the numbering doesn't count properly. In the else (count + 1) won't work, it always print 1 before the wrong question. I've been at it for like 4 hours and I want to move on.
var questions = [
["How many states are in the USA?", "50"],
["How many countries are in the world?", "195"],
["What is 2 + 2?", "4"]
];
var count = 0;
var userAnswer;
var numOfCorrectAnswers;
var rightQuestions = "";
var wrongQuestions = "";
for(var i = 0; i < questions.length; i++){
userAnswer = prompt(questions[i][0]);
if(userAnswer.includes(questions[i][1])){
rightQuestions += "<br>" + (count + 1) + " " + questions[i][0];
count++;
} else {
wrongQuestions += "<br>" + (count + 1) + " " + questions[i][0];
}
}
numOfCorrectAnswers = print("You got " + count + " question(s) right.");
function print(numOfCorrectAnswers) {
document.write(numOfCorrectAnswers + "<br><br>");
document.write("<strong>You got these questions correct:</strong> <br><br>");
document.write(rightQuestions + "<br><br>");
document.write("<strong>You got these questions wrong:</strong> <br><br>");
document.write(wrongQuestions);
}
2 Answers
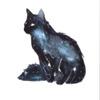
Antony .
2,824 PointsI would suggest adding another variable, call it whatever, as long as it distinguishes the right questions from the wrong. For e.g.,
var count = 0; //you can change the variable name to goodCount to be more descriptive if you like.
var badCount = 0;
then you can accumulate badCount on your else statement, and also make sure to change the variable inside your parentheses.
} else {
wrongQuestions += "<br>" + (badCount + 1) + " " + questions[i][0];
badCount++;
}
I feel like there's a more efficient way of doing this so I'm going to leave this as an alternative for you.

Siddharth Pande
9,046 Pointsfunction print(message) {
document.write(message);
}
var questArray = [
["Which gas helps in burning?","OXYGEN"],
["Who is the incumbent Prime Minister of India?","NARENDRA MODI"],
["Which state is the Capital of India?","DELHI"]
];
//All the main code is in this function:
function quiz (array) { //array is a parameter where argument will be passed.
var finalHtml = "<p>" //To produce the Html code
/*To make the
wrong answer Html Code*/
var wrongStr = "<h4>You got these questions wrong: </h4><ol>"
var correctAnswer = 0; // Counter for number of correct guesses
// To Make the correct Answer Html Code
var correctStr = "<h4> You got these questions correct: </h4><ol>";
//Engine(loop) where questions are asked and answers are
//compared by iterating through the loop and using a decision making if statement.
for(i=0; i < array.length; i += 1) {
var guess = prompt(array[i][0]);
if (guess.toUpperCase() === array[i][1]) {
correctAnswer += 1;
correctStr += "<li>" + array[i][0] + "</li>"
} else {
wrongStr += "<li>" + array[i][0] +"</li>"
}
}
//completing the Html code
finalHtml += "You got " + correctAnswer +
" question(s) right. </p> </br>" +
correctStr + "</ol> </br>" + wrongStr + "</ol>";
//Printing the final Html code on the document
print(finalHtml);
}
quiz(questArray); //Run the Program
Nelson J
7,411 PointsNelson J
7,411 PointsThank you that's what I was missing. I am sure there is a more efficient way but I would like to move on and play around with code, I feel like the more I practice the better I learn, like practice with newer problems. I saw the solutions after doing this and the author is clearly a lot more experienced than me that his solution is very concise. Thanks again.