Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial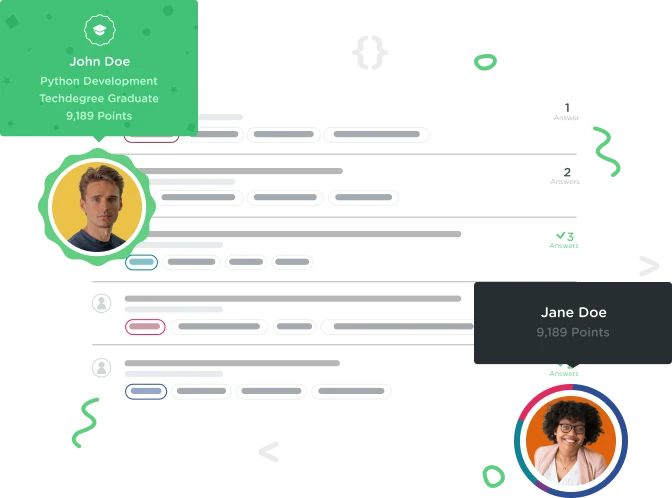
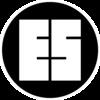
Ephraim Smith
11,930 PointsBuild a REST API with Spring: At a stand still with course: "Error creating bean with name 'restHandlerMapping'"...
I have the following exceptions and have been unable to find any solutions that address them:
- BeanInstantiationException
- IllegalStateException
Up to this point I've had to research errors/warnings/Exceptions/outdated syntax for every section of the course. Is anyone else running into this?
Any help on this would be appreciated!
P.S. Any Treehouse moderators know if this course could be updated or removed? I know research is part of the game, but being a paid service, it shouldn't be required for every section of a course.
Thanks in advance everyone! Have a good night. -E

Michael Switzer
7,490 PointsI just finished that course and I remember running into issues in several places. Where are you at in the course specifically? Nevermind, I figured it out. What is the code you just finished writing before you tried to run the application and got the error?
Also, for these courses, instead of using the versions in gradle that the course used, I updated mine to use the current versions of all the dependencies, but I don't think that should make much of a difference.
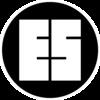
Ephraim Smith
11,930 PointsHey Mike,
The exceptions started right after trying to extend the Spring repo "PagingAndSortingRepository" in my Repository classes.

Michael Switzer
7,490 PointsThat happens at the beginning of the next video. When you ran the build at the end of the "Populating and Relationships" video, did your code work?
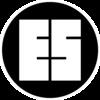
Ephraim Smith
11,930 PointsHey man,
No, that's when it broke.

Michael Switzer
7,490 PointsPost the code you have for your classes, you probably have a syntax error. Make sure you use the Markdown for posting your code so that it shows up formatted in a readable way
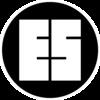
Ephraim Smith
11,930 PointsThanks for taking a look at this Mike. I followed the videos line-for-line. I'm guessing I need to update some syntax somewhere.
"Error starting ApplicationContext".
org.springframework.beans.factory.BeanCreationException: Error creating bean with name 'restHandlerMapping' defined in class path resource [org/springframework/data/rest/webmvc/config/RepositoryRestMvcConfiguration.class]
CAUSED BY: org.springframework.beans.BeanInstantiationException: Failed to instantiate
CAUSED BY: java.lang.IllegalStateException: Missing path mapping.
Course.java
package com.modevx.course;
import com.modevx.core.BaseEntity;
import com.modevx.review.Review;
import javax.persistence.*;
import java.util.ArrayList;
import java.util.List;
@Entity
public class Course extends BaseEntity {
private String title;
private String url;
@OneToMany(mappedBy = "course", cascade = CascadeType.ALL)
private List<Review> reviews;
protected Course() {
super();
reviews = new ArrayList<>();
}
public Course(String title, String url) {
this();
this.title = title;
this.url = url;
}
public List<Review> getReviews() {
return reviews;
}
public void addReview(Review review) {
review.setCourse(this);
reviews.add(review);
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
}
CourseRepository.java
package com.modevx.course;
import org.springframework.data.repository.CrudRepository;
import org.springframework.data.repository.PagingAndSortingRepository;
public interface CourseRepository extends PagingAndSortingRepository<Course, Long> {
}
Review.java
package com.modevx.review;
import com.modevx.core.BaseEntity;
import com.modevx.course.Course;
import javax.persistence.*;
@Entity
public class Review extends BaseEntity {
private int rating;
private String description;
@ManyToOne
private Course course;
protected Review() {
super();
}
public Review(int rating, String description) {
this.rating = rating;
this.description = description;
}
public Course getCourse() {
return course;
}
public void setCourse(Course course) {
this.course = course;
}
public int getRating() {
return rating;
}
public void setRating(int rating) {
this.rating = rating;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
}
ReviewRepository.java
package com.modevx.review;
import org.springframework.data.repository.CrudRepository;
import org.springframework.data.repository.PagingAndSortingRepository;
public interface ReviewRepository extends PagingAndSortingRepository<Review, Long> {
}
Application.java
package com.modevx;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
DataBaseLoader.java
package com.modevx.core;
import com.modevx.course.Course;
import com.modevx.course.CourseRepository;
import com.modevx.review.Review;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.ApplicationArguments;
import org.springframework.boot.ApplicationRunner;
import org.springframework.stereotype.Component;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.IntStream;
@Component
public class DatabaseLoader implements ApplicationRunner {
private final CourseRepository courses;
@Autowired
public DatabaseLoader(CourseRepository courses) {
this.courses = courses;
}
@Override
public void run(ApplicationArguments args) throws Exception {
Course course = new Course("Java Basics", "teamtreehouse.com/library/java-basics");
course.addReview(new Review(3, "This is a new review"));
courses.save(course);
String[] templates = {
"Up and running with %s",
"%s Basics",
"%s for Beginners",
"Under the hood: %s"
};
String[] buzzwords = {
"Spring REST Data",
"Java 9",
"Scala",
"Groovy"
};
List<Course> bunchOfCourses = new ArrayList<>();
IntStream.range(0, 100).forEach(i -> {
String template = templates[i % templates.length];
String buzzword = buzzwords[i % buzzwords.length];
String title = String.format(template, buzzword);
Course c = new Course(title, "http://www.example.com");
c.addReview(new Review(i % 5, String.format("More %s please!", buzzword)));
bunchOfCourses.add(c);
});
courses.saveAll(bunchOfCourses);
}
}
BaseEntity.java
package com.modevx.core;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.MappedSuperclass;
@MappedSuperclass
public abstract class BaseEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private final Long id;
protected BaseEntity() {
id = null;
}
}

Michael Switzer
7,490 PointsI'm not sure what's causing the problem. The only thing I can think of at this point is to set a break point and then run the program in debug mode and just step through it line by line until you hit the place where it chokes. Either that or go back to the point in the video where it worked and then retrace the steps.
Stefan Novak
6,791 PointsStefan Novak
6,791 PointsI'm sorry I can't help, I just wanted to agree with you with you post script, research is fine but to have to update every single section yourself is really frustrating. The course is in major need of an update.