Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial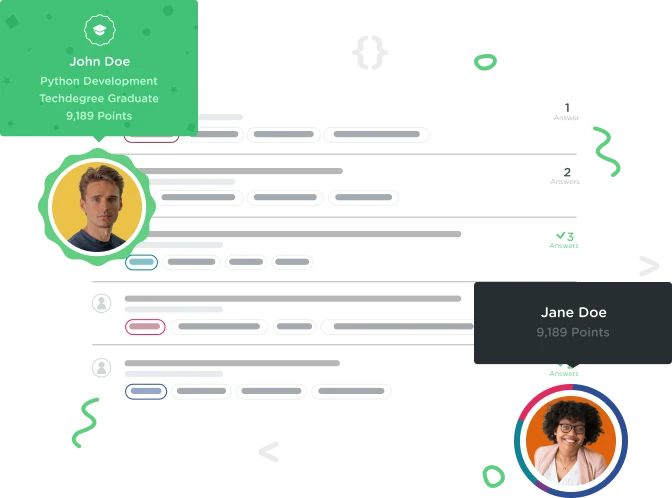

Thomas Nilsen
14,957 PointsBuild a Simple iPhone App stage 4, challenge 2 / 3
Here's the challenge:
Switch to the implementation file and implement a 'quotes' method which will allocate and initialize the '_quotes' instance variable only if it is 'nil'. Initialize the array with any three strings.
My code:
- (NSString *) quotes{ if(_quotes == nil){ _quotes = [[NSArray alloc] initWithObjects:@"Hey", "Thomas", @"Nilsen"]; } return _quotes; }
Where is my mistake?
4 Answers

John W
21,558 PointsYou have three bugs there:
You need a terminating nil when initializing an array (or any other kind of collections) using this method, otherwise initWithObjects will not know when to stop reading.
Each object should be an NSString instead of Cstring, i.e. each string should start with the @ directive.
Consider what your method is returning. Is your method declared to return the same class?
Considering the number of problems in your solution, it is probably a good idea that you re-watch some of the videos to make sure you understand the structure of Objective-C before you move forward to more advanced videos. Additional resources and tutorials are also abundant everything online that can help you understand the basics in alternative ways that may serve you better as well.
The new project Objective-C basics (https://teamtreehouse.com/library/objectivec-basics) here at treehouse will likely be helpful for you as well.

John W
21,558 PointsHere's the video that gives you almost exactly the code you need, starting at 2:30. Though you are encouraged to watch it from the beginning to understand how to set up a @property.
One last hint: you want something like this before implementing randomQuote. Just fill in whatever you learn from the video.
- (NSArray *)quotes{
...
}

Thomas Nilsen
14,957 PointsThank you! I got confused about the setters and getters because in previous videos they said @property handles all that for us. So when I. in challenge one declared a @property - variable, I didn't think we'd have setters and getters anymore.
Again, thank you!

Brooks Wood
Courses Plus Student 2,147 PointsFound answer after scouting around in the other forums for this issue.
Thomas Nilsen
14,957 PointsThomas Nilsen
14,957 PointsI fixed it
import "Quote.h"
@implementation Quote
int random = arc4random_uniform(self.quotes.count);
return [self.quotes objectAtIndex:random]; } @end
Still doesn't work... Any suggestions?
The error I get: "You need to allocate and initialize the 'quotes' array property!"