Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial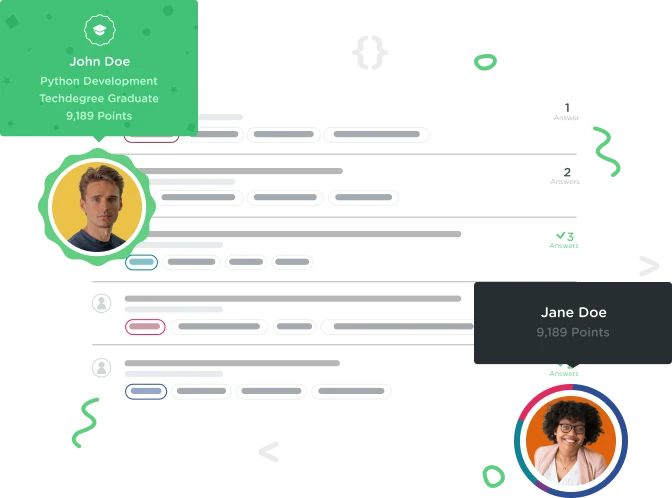
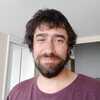
Rodrigo Muñoz
Courses Plus Student 20,171 PointsBuild a Simple PHP Application > Listing Inventory Items > Introducing Arrays > Task: 6/7
Help with this code challenge:
The fourth echo statement, the one inside the foreach loop, should only display one letter at a time. Change that fourth echo statement so that it does not display the static piece of text AB; instead, make it display the value for the array element under consideration inside the foreach loop.
<?php $letters = array("D","G","L");
echo "My favorite "; echo count($letters); echo " letters are these: "; foreach($letters as $letter){ echo $letter . "\n"; } echo "AB"; echo "."; ?>
This is what I did:
<?php $letters = array("D","G","L","AB");
echo "My favorite "; echo count($letters); echo " letters are these: "; foreach($letters as $letter){ echo $letter . "\n"; } echo "."; ?>
It returns that I have to many letters. Should be displaying one letter inside the foreach loop.
13 Answers

Randy Hoyt
Treehouse Guest TeacherI apologize that my error message is not clear.
- You should be displaying one letter at a time inside the foreach loop. That would be three letters total.
- The hard return is counting as a "letter", so right now you are displaying two letters at a time in the foreach loop for a total of six.
I wasn't expecting you to put in hard returns, so my error message is not clear. :~) I'll fix some of the checks and messages in the challenge to account for hard returns.
But if you remove the hard return, changing this ...
foreach($letters as $letter){
echo $letter . "\n";
}
... to this ...
foreach($letters as $letter){
echo $letter;
}
... then I believe it will pass.
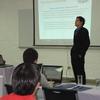
Carlos Sura
6,680 PointsRandy Hoyt I am new here, I can say you really are a great teacher, I really love to watch your videos, but this challange was a little bit confusing, I had to read the post to pass it, even that the fact that I was doing what you asked on the instructions, if you can clear this for others guys would be great!
Thanks.
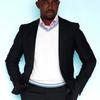
Isaiah Etuk
2,597 Points<?php
$letters = array("D", "G", "L");
echo "My favorite "; echo count($letters); echo " letters are these: "; foreach($letters as $letter){ echo $letter[0]; echo $letter[1]; echo $letter[3];
} echo ".";
?>

Randy Hoyt
Treehouse Guest TeacherI think the issue is that you have a hard return inside the foreach loop. Instead of this ...
foreach($letters as $letter){
echo $letter . "\n";
}
... change it to this ...
foreach($letters as $letter){
echo $letter;
}
The two letters that were there before were all on one line ...
AB
... and these new ones need to be on one line also ...
DGL
Does that help?
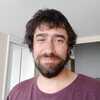
Rodrigo Muñoz
Courses Plus Student 20,171 PointsIt says that there are too many letters and should only be displaying one letter inside the foreach loop. The thing that I don't understand is "how to display only one letter if I am using a foreach loop?" .
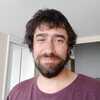
Rodrigo Muñoz
Courses Plus Student 20,171 PointsIt was strange, I just deleted the "AB" and excecute the code and it passed. I don't know if I was right but I just wanted to keep going with the excercises. @Randy thanks for the help.

Randy Hoyt
Treehouse Guest TeacherCool. Glad you got it working! I thought you had already removed the AB altogether, but I see now that you had moved it to the array. I'm glad to hear that the hard returns weren't counted as letters! You should have only had three letters ...
DGL
... but it sounds like you had five.
DGLAB
Let me know if you have more questions! :~)

Ryan Krienitz
4,403 PointsIs this wrong? It still errors out even if you have: <?php
$letters = array("D","G","L"); echo "My favorite "; echo count($letters); echo " letters are these:"; foreach($letters as $letter){ echo $letter;
} echo ""; echo ".";
?>

Randy Hoyt
Treehouse Guest TeacherHmmm ... it looks like you are missing a space after the colon. Your output looks like this ...
My favorite 3 letters are these:DGL.
... when it should look like this ...
My favorite 3 letters are these: DGL.
The error message isn't very helpful there ... sorry about that! :~) I'll need to update the error message to account for this scenario.
Does that help?

Eduardo La Hoz
3,881 PointsThis exercise is a bit confusing. From reading the above, I just deleted the echo "AB"; entirely and it passed. But I have no idea if that was what was intended. Maybe making the user add AB as the third index in the array would be a more understandable exercise.

Randy Hoyt
Treehouse Guest TeacherHey @Eduardo,
Thanks for the feedback. I made some changes to the instructions for the code challenge that hopefully clarify it. Would you mind taking a look and letting me know if it's clearer now?
The code block below displays a list of two letters from the Latin alphabet using echo statements. But these two letters are NOT my favorite letters! In this code challenge, we’ll change this block of code to display my real favorite letters. We’ll also modify it to use an array so that it’s easier to add a new letter in the future.
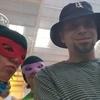
Robbie Thomas
31,093 PointsIt helped!
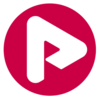
Tech Solutions
6,077 PointsHi Randy,
I think this still needs fixing, it's a great tutorial otherwise but this particular challenge is confusing, I had already tried
foreach($letters as $letter){
echo $letter;
}
before checking this forum, it's pretty disconcerting to enter what you're certain is correct code and have it fail, the solution as others on here have said is to use this code but not add "AB" to the array, it is not clear what your favourite letters are so I assumed (as I'm sure others did too) that AB was one of them. Surely you could add some conditional to your validation for this task to allow the addition of AB to the array while also allowing it to pass without it?