Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial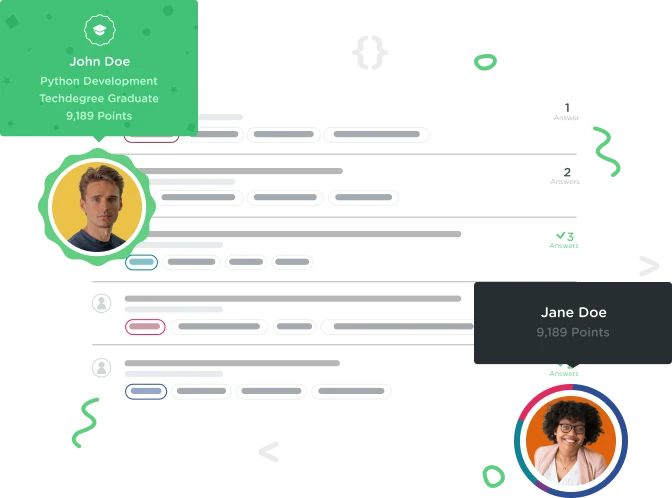

Tafadzwa Timothy Gakaka
10,978 PointsBuild A social network with flask
Add a new model named Relationship. It should have a ForeignKeyField, related to User. The field should be named from_user with a related_name of "relationships".
help
import datetime
from flask.ext.bcrypt import generate_password_hash
from flask.ext.login import UserMixin
from peewee import *
DATABASE = SqliteDatabase(':memory:')
class User(UserMixin, Model):
email = CharField(unique=True)
password = CharField(max_length=100)
join_date = DateTimeField(default=datetime.datetime.now)
bio = CharField(default='')
user = ForeignKeyField(
rel_model=User,
related_name='post'
)
class Relationship(Model):
from_user = ForeignKeyField(User, related_name='relationships')
to_user = ForeignKeyField(User, related_name='related_to')
class Meta:
database = DATABASE
@classmethod
def new(cls, email, password):
cls.create(
email=email,
password=generate_password_hash(password)
)
class LunchOrder(Model):
order = TextField()
date = DateField()
user = ForeignKeyField(User, related_name="orders")
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, LunchOrder], safe=True)
DATABASE.close()
4 Answers
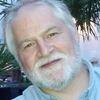
Jeff Muday
Treehouse Moderator 28,722 PointsTafadzwa,
You aren't too far from a solution here. Good work!
If you take the "Relationship" model and separate it from the "User" model, you will pass the first two parts of the Challenge.
There are two other parts of the Challenge afterward, but this will get you going.
best, Jeff
class User(UserMixin, Model):
email = CharField(unique=True)
password = CharField(max_length=100)
join_date = DateTimeField(default=datetime.datetime.now)
bio = CharField(default='')
user = ForeignKeyField(
rel_model=User,
related_name='post'
)
class Meta:
database = DATABASE
@classmethod
def new(cls, email, password):
cls.create(
email=email,
password=generate_password_hash(password)
)
class Relationship(Model):
from_user = ForeignKeyField(User, related_name='relationships')
to_user = ForeignKeyField(User, related_name='related_to')

Tafadzwa Timothy Gakaka
10,978 Pointsit keeps saying user not defined do you mind sending the full code
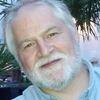
Jeff Muday
Treehouse Moderator 28,722 PointsThis is a really good challenge and takes a bit of effort to get all the parts correct. Especially the last part the syntax is slightly tricky because of a Python tuple requirement of a trailing comma for a one-element tuple.
Charles Leifer's documentation on PeeWee is admirable. I had the pleasure of meeting Charles at a conference a number of years ago-- very intelligent, very humble fellow. I am glad Kenneth Love selected teaching PeeWee over SQLAlchemy, it's flexible and simpler to learn. But when you get out there in the world of developers, you should be aware that SQLAlchemy has a larger community.
http://docs.peewee-orm.com/en/latest/peewee/models.html#indexes-and-constraints
Spoiler alert on code below--
# Challenge part 1 code below
class Relationship(Model):
from_user = ForeignKeyField(User, related_name='relationships')
# Challenge part 2 adds the code below
to_user = ForeignKeyField(User, related_name='related_to')
# Challenge part 3 adds the code below
created_at = DateTimeField(default=datetime.datetime.now)
# Challenge part 4 adds the code below
class Meta:
database = DATABASE
# the hardest part is the indexes as this is slightly less common
# reference documentation:
# http://docs.peewee-orm.com/en/latest/peewee/models.html#indexes-and-constraints
indexes = (
(('from_user','to_user'), True),
)

Tafadzwa Timothy Gakaka
10,978 Pointsimport datetime
from flask.ext.bcrypt import generate_password_hash from flask.ext.login import UserMixin from peewee import *
DATABASE = SqliteDatabase(':memory:')
class User(UserMixin, Model): email = CharField(unique=True) password = CharField(max_length=100) join_date = DateTimeField(default=datetime.datetime.now) bio = CharField(default='')
class Meta:
database = DATABASE
class Relationship(Model): from_user = ForeignKeyField(User, related_name='relationships') to_user = ForeignKeyField(User, related_name='related_to') created_at = DateTimeField(default=datetime.datetime.now)
class Meta:
database = DATABASE
indexes = (
(('from_user','to_user'), True),
)
@classmethod
def new(cls, email, password):
cls.create(
email=email,
password=generate_password_hash(password)
)
class LunchOrder(Model): order = TextField() date = DateField() user = ForeignKeyField(User, related_name="orders")
def initialize(): DATABASE.connect() DATABASE.create_tables([User, LunchOrder], safe=True) DATABASE.close()
i dont knw where im goin wrong this challenge got me stuck