Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial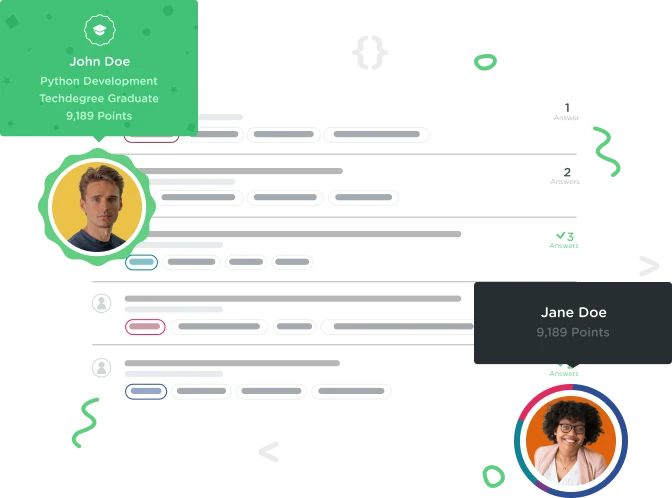

Tafadzwa Timothy Gakaka
10,978 PointsBuild a social network with flask
Add a new model named Relationship. It should have a ForeignKeyField, related to User. The field should be named from_user with a related_name of "relationships".
i have been stuck here for days can someone kindly assist me with the full code .
import datetime
from flask.ext.bcrypt import generate_password_hash
from flask.ext.login import UserMixin
from peewee import *
DATABASE = SqliteDatabase(':memory:')
class User(UserMixin, Model):
email = CharField(unique=True)
password = CharField(max_length=100)
join_date = DateTimeField(default=datetime.datetime.now)
bio = CharField(default='')
class Meta:
database = DATABASE
class Relationship(Model):
from_user = ForeignKeyField(User, related_name='relationships')
to_user = ForeignKeyField(User, related_name='related_to')
created_at = DateTimeField(default=datetime.datetime.now)
class Meta:
database = DATABASE
indexes = (
(('from_user','to_user'), True),
)
@classmethod
def new(cls, email, password):
cls.create(
email=email,
password=generate_password_hash(password)
)
class LunchOrder(Model):
order = TextField()
date = DateField()
user = ForeignKeyField(User, related_name="orders")
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, LunchOrder], safe=True)
DATABASE.close()
2 Answers
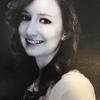
Megan Amendola
Treehouse TeacherThe relationship model should go outside the User class. Right now it is in the middle making the classmethod now relate to the relationship instead of the user class.
import datetime
from flask.ext.bcrypt import generate_password_hash
from flask.ext.login import UserMixin
from peewee import *
DATABASE = SqliteDatabase(':memory:')
class User(UserMixin, Model):
email = CharField(unique=True)
password = CharField(max_length=100)
join_date = DateTimeField(default=datetime.datetime.now)
bio = CharField(default='')
class Meta:
database = DATABASE
@classmethod
def new(cls, email, password):
cls.create(
email=email,
password=generate_password_hash(password)
)
class LunchOrder(Model):
order = TextField()
date = DateField()
user = ForeignKeyField(User, related_name="orders")
class Relationship(Model):
from_user = ForeignKeyField(User, related_name="relationships")
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, LunchOrder], safe=True)
DATABASE.close()

Tafadzwa Timothy Gakaka
10,978 Pointsthank you so much