Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial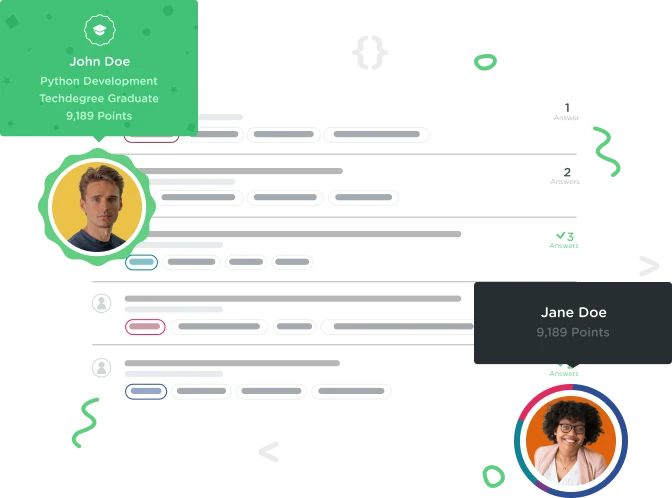
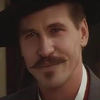
huckleberry
14,636 PointsBuild an Object Challenge, Part 1: Pre Solution-Vid.
Hello all you sassy little scripters out there,
Here's my attempt at the lesson based on ol' Dave McFarland 's instructions as given in the part1 video.
//Build an object challenge
/*+++
1. create a script that creates a bunch of student records
2. then prints those records to a webpage
Steps:
1. create a data structure to hold information about a group of students
an array named students
this will hold a list of objects
each object will represent a student
each object will contain the following properties.
name
track
achievements - number value
points - number value
at least 5 student objects
*/
I decided to take the opportunity to use the JSON thingy he taught us by accessing the teamtreehouse/uername.json for various users I'd then grab the data and count the badges and check the points that way. Yeah, could've just gone to their profile pages but how ya gonna learn that way?
Anyway... my code is below... and scroll to the end for my codepen of it.
function print(message){
document.write(message);
return "As you requested, sir."
};
var students = [
{
name: "Huckleberry",
track: "Starting a Business",
achievements: 90,
points: 7502
},
{
name: "Marcus Parsons",
track: "PHP Development",
achievements: 97,
points: 9544
},
{
name: "Nicholas Olsen",
track: "Front End Development",
achievements: 158,
points: 11289
},
{
name: "William Li",
track: "Web Design",
achievements: 157,
points: 17809
},
{
name: "Colin Key",
track: "Web Design",
achievements: 16,
points: 1023
}
];
var confirm = prompt("Would you like to meet our students?");
makeItSo = confirm.toUpperCase();
if (makeItSo === 'YES'){
for (var i = 0; i < students.length; i++){
var studentList = "<p>Fantastical and Fantabulous Student #" + (i + 1) + " is:<br><ul>";
for (prop in students[i]){
studentList += "<li><span>" + prop + "</span>: " + students[i][prop] + "</li>"
console.log(prop, ":", students[i][prop]);
}
studentList+= "</ul></p>";
print(studentList);
}
} else {
alert("OH YEAH? WELL... WELL YOU'RE NOT MY REAL MOM!");
}
http://codepen.io/huckleberry/pen/zxVGqp
I'm not sure if this is how he wanted it done but it works out nicely. Any comments, suggestions or "lol... foolish Huck" comments are welcome and appreciated.
Cheers,
Huck -
3 Answers
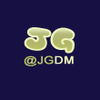
Jonathan Grieve
Treehouse Moderator 91,252 PointsGreat job Hucke. :)
I'm interested in why in your codepen when you close the #output div it wrecks the CSS list style property and shows the bullet points. I don't know why closing the element would make such a difference but the script looks great. :)

Jake Hudson
7,841 PointsYeah... that is a real head scratcher... I guess its a codepen bug? or, some fancy un-advertized feature where you don't have to close your divs...

Iain Simmons
Treehouse Moderator 32,305 PointsThe reason you get the bullet points in the CodePen when you close the div is because you're using document.write
, which just outputs the string/HTML wherever the script is in the HTML, which for CodePen, is always just before the closing body
tag.
Change your print
function to the following and it should work:
function print(message){
document.getElementById('output').innerHTML = message;
return console.log("As you requested, sir.");
}
Otherwise, nice job!
Here's mine:
var html = '';
var students = [
{
name: 'Iain Simmons',
track: 'Full Stack JavaScript',
achievements: 219,
points: 18767
},
{
name: 'Dave McFarland',
track: 'Full Stack JavaScript',
achievements: 197,
points: 18375
},
{
name: 'Kenneth Love',
track: 'Learn Python',
achievements: 38,
points: 8504
},
{
name: 'Andrew Chalkley',
track: 'Full Stack JavaScript',
achievements: 175,
points: 14544
},
{
name: 'Nick Pettit',
track: 'Front End Web Development',
achievements: 79,
points: 6470
}
];
function print(nodeID, message) {
document.getElementById(nodeID).innerHTML = message;
}
function buildDefinitionList(obj) {
var prop;
var dListHTML = '<dl>';
for (prop in obj) {
dListHTML += '<dt>' + prop + '</dt>';
dListHTML += '<dd>' + obj[prop] + '</dd>';
}
dListHTML += '</dl>';
return dListHTML;
}
function buildStudentList(list) {
var i;
var uListHTML = '<ul>';
for (i = 0; i < list.length; i++) {
uListHTML += '<li>' + buildDefinitionList(list[i]) + '</li>';
}
uListHTML += '</ul>';
return uListHTML;
}
html = buildStudentList(students);
print('output', html);
huckleberry
14,636 Pointshuckleberry
14,636 PointsThank you!
And yeeeah I noticed that as well... what gives?? I really hadn't been using codepen and hadn't touched it in like a year and a half... back when I first started even dabbling with styling a hello world html doc. But it hit me that it would probably be best for posting these kinds of projects rather than just "here's ma codez! wutz yall thinkins on it?" .... BLEGH. So I gets over there and I'm completely unfamiliar with its various pecadillos. Like when it started yelling at me to get rid of doctype and the head and all that. I'm like take it easy bro... it's my first day. Right?
So yeah, if anyone wandering through knows why closing that div wrecks it, me and the Grieves would love to know why.
Cheers,
Huck -