Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial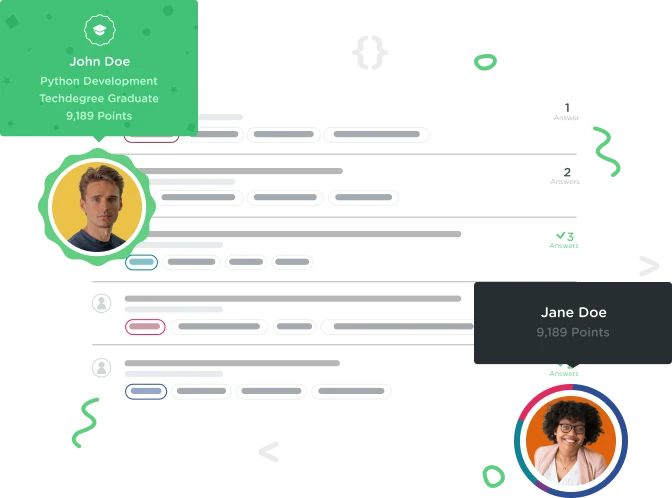
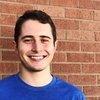
Caleb Jost
6,072 PointsBuild an Object: My Solution
I couldn't believe it when this finally worked. Here's my solution, let me know if anyone has improvements. I still don't 100% understand that print(message) function.
var students = [ { Name: 'Caleb', Track: 'Front-end Development', Achievements: 50, Points: 1000, },
{ Name: 'Cristof', Track: 'FullStack JavaScript', Achievements: 49, Points: 999 },
{ Name: 'Yanet', Track: 'iOs Development', Achievements: 30, Points: 2000 },
{ Name: 'Boberton', Track: 'Front-end Development', Achievements: 78, Points: 3000 },
{ Name: 'Jimbo', Track: 'iOS Development', Achievements: 62, Points: 250 }, ];
function print(message) { var outputDiv = document.getElementById('output'); outputDiv.innerHTML=message; }
var html; for (var i=0; i < students.length; i+=1) { html += '<p>Student Name: ' + students[i].Name + '</p>'; html += '<p>Track: ' + students[i].Track + '</p>'; html += '<p>Achievements: ' + students[i].Achievements + '</p>'; html += '<p>Points: ' + students[i].Points + '</p>'; html += '<br>'+'</br>'; }
print(html);
1 Answer
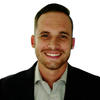
Joshua Petry
6,313 PointsHere's my code:
var printedRecords = "";
var student = [
{name: 'Josh', track: 'Javascript', achievements: 10, points: 4560},
{name: 'Jon', track: 'iOS', achievements: 10, points: 4560},
{name: 'Sam', track: 'Java', achievements: 10, points: 4560},
{name: 'Avery', track: 'PHP', achievements: 10, points: 4560},
{name: 'Chelsea', track: 'Web Design', achievements: 10, points: 4560}
];
for ( i = 0; i < student.length; i++ ) {
printedRecords += '<p>Name: ' + student[i].name + '<br>';
printedRecords += 'Track: ' + student[i].track + '<br>';
printedRecords += 'Achievements: ' + parseInt(student[i].achievements) + '<br>';
printedRecords += 'Points: ' + parseInt(student[i].points) + '</p>';
}
document.write(printedRecords);

Junet Ismail
3,563 PointsHi Josh you could refactor the second part of your code as such using the for in as covered in the course
var title =['Name','Track','Achievements','Points']; var titlecount ;
for ( i = 0; i < student.length; i++ ) { titlecount = 0; for (var identifier in student){
printedRecords += '<p> ' +title[titlecount]+':'+ student[i][identifier] + '</p>'; titlecount+=1; } } } document.write(printedRecords);
Marija Shakleva
2,608 PointsMarija Shakleva
2,608 PointsHere is my code, I know it's better to have it in a function, but this was a first try and it kind of awesomely worked :D
var html = document.getElementById('output');
for(var i = 0; i<students.length; i++){ for(var student in students[i]){ html.innerHTML += '<p>' + student + ': ' + students[i][student] + '</p>'; } }
The print message function is really cool actually, first with var outputDiv = document.getElementById('output') it lets you select an element from the DOM and then with outputDiv.innerHTML=message; it lets you modify it's content/structure/HTML by passing a parameter message which doesn't always have to be a structure with <p> or a <ul>, but anything you want.
I think you can improve your code definitely, so try this instead of the for you use: for(var i = 0; i<students.length; i++){ for(var student in students[i]){ html += '<p>' + student + ': ' + students[i][student] + '</p>'; } } P.S. You don't need commas on last array elements, last objects etc.