Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial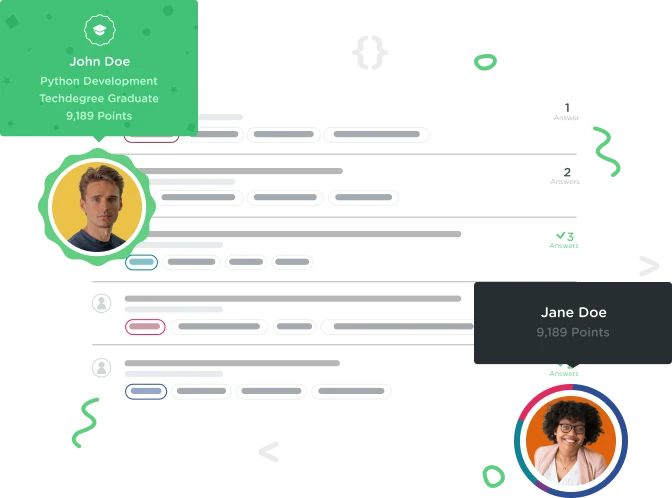

Benjamin Raskin
4,075 PointsBuilding a multiple choice game
Hi guys, I'm trying to build a multiple choice app. Below is what I have so far:
Models:
class Answer < ActiveRecord::Base
belongs_to :question
end
class Question < ActiveRecord::Base
has_many :answers
end
Controller:
class QuizzesController < ApplicationController
def show
@question = Question.find(params[:id])
@answer = Answer.find(params[:id])
end
end
Schema:
ActiveRecord::Schema.define(version: 20150418024450) do
create_table "answers", force: :cascade do |t|
t.string "wrong_answer_1"
t.string "wrong_answer_2"
t.string "wrong_answer_3"
t.string "correct_answer"
t.datetime "created_at", null: false
t.datetime "updated_at", null: false
t.integer "question_id"
end
add_index "answers", ["question_id"], name: "index_answers_on_question_id"
create_table "questions", force: :cascade do |t|
t.string "question"
t.datetime "created_at", null: false
t.datetime "updated_at", null: false
end
end
View:
<h1>Quizzes</h1>
<%= @question.question %>
<% @question.answers.each do |answer| %>
<li>
<%= radio_button_tag("answer[question.id]", answer.id) %>
<%= label("answer_".concat(answer.id.to_s).to_sym, answer.wrong_answer_1) %>
</li>
<% end %>
I have a couple questions. First of all, how do I add all of my answers (wrong_answer_1, wrong_answer_2, etc.) in here:
<%= label("answer_".concat(answer.id.to_s).to_sym, answer.wrong_answer_1) %>
How would i create a button that when clicked would flash either success or failure depending on if the correct answer was selected?
Thanks for the help!
Andrew Stelmach
12,583 PointsAndrew Stelmach
12,583 PointsSorry I can't spend much time on this, but I think you need to build a form_tag:
...using multiple radio_button_tags for the answers. Then use a submit_tag for the button. The form_tag will point to a custom method that you have written in your QuizzesController, called 'check_answer'. Your routes.rb file will have
post 'check_answer' => 'quizzes#check_answer'
I'm sorry I can't do much more right now - way too late. Hopefully that was of some help. The tricky part is the 'stuff in here' part (and the controller method I guess, but less so).
Disclaimer: I might be completely wrong.