Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial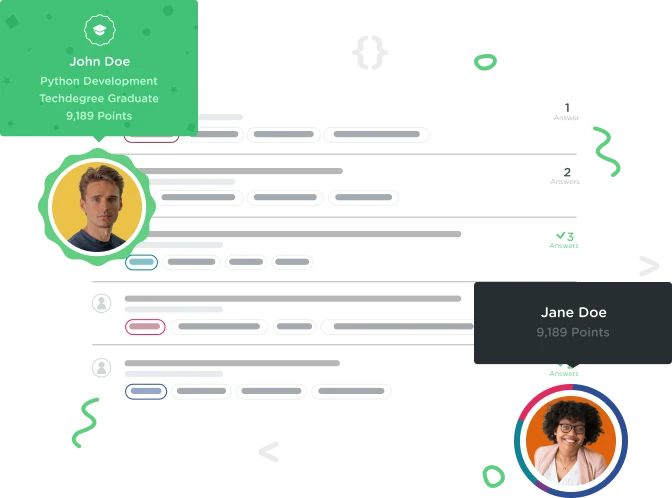
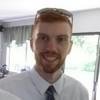
Alex Ross
3,091 PointsBuilding a simple iPhone app robot challenge.
I've been working on this one for a while and can't seem to figure out something which works. After trying my hand at it I searched many other posts people have done and none of their proposed answers worked either. Due to the fact the original code provided already had some bugs I had to remove... maybe this challenge is just bugged?
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(_ direction: String) {
print("Do nothing! Im a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(direction: String) {
let move = ["Up", "Down", "Left", "Right"]
let direction = location
switch move {
case "Up": Point(y + 1)
case "Down": Point(y - 1)
case "Left": Point(x - 1)
case "Right": Point(x + 1)
default: break
}
}
}

Jonathan Law
iOS Development Techdegree Student 6,824 PointsWas just working on this one also. Wish I knew how to copy directly from my code after submitting, but this is what worked for me:
1) You don't need the declarations: let move = ["Up, "Down", etc...] let direction = location
2) Just switch on "move" like you did and then for each case, instead of Point(y + 1), etc. just use case "Up": location.y += 1 case "Down": location.y -= 1 etc.
Hope that helps! Wish I was more experienced in Swift to provide a deeper explanation but it just looks like you tried to do too much and since the new class inherits the "location" variable from the super-class you can just access it directly using dot notation, combine it with the x or y variable and then add or subtract from it in the switch.

Donald Zarraonandia
4,434 PointsWhats up Alex.
This one gave me some trouble as well when I first worked on it.
You have a couple things I would change.
First you do not need to the move array. The function will ask for this string when called.
Second you need to get rid of direction for two reasons. One it is declared in the super class. And two you can only make changes to a var.
Third you will want to use += 1 and -= 1. This syntax will shorten your code.
Fourth you are trying to reference either the x or y point in the var location. To do this you should use dot notation. Which would look something like this: location.y
Lastly you will need a _ in the func move. This is the external name. You will still use direction inside the function. However when called it will look like this ("Up") instead of (direction: "Up").
Here is a copy of my code that passed the challenge:
class Robot: Machine {
override func move(_ direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break
}
}
}
Jase-Omeileo West
2,011 PointsJase-Omeileo West
2,011 PointsYou are switching on an array, you need to switch on the "direction" variable. In each case, your call to the Point() class is expecting an object to be returned so you need to be storing it in something ;). You also don't need the move String constant. location is a Point attribute but you are trying to store a String in it.