Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial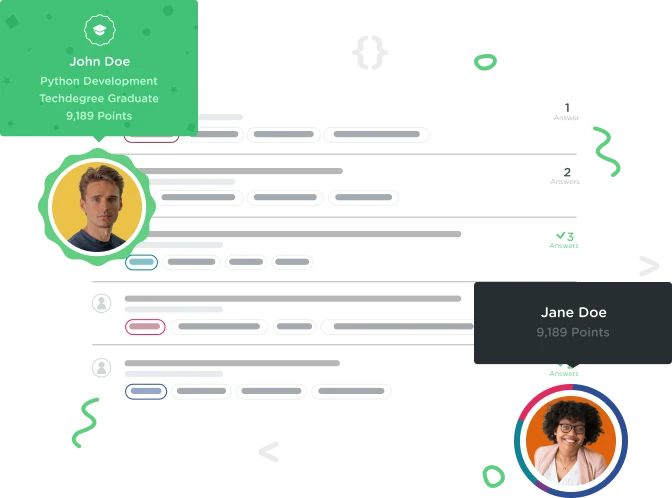

james white
78,399 PointsBummer! Can't find the formset in the HTML. Did you remove it?
I can't figure out what this Bummer message is trying to tell me...?
I didn't delete any part of the code that was originally presented in the challenge.
I only added some lines to check for form validity:
def bulk_create_products(request):
formset = forms.DigitalFormset()
if form.is_valid():
form.save()
return HttpResponseRedirect(reverse('products:create'))
return render(request, 'products/bulk_create.html', {'formset': formset})
The other slight variation I tired (and got the same Bummer!):
def bulk_create_products(request):
formset = forms.DigitalFormset()
if form.is_valid():
form.save()
return HttpResponseRedirect(reverse('products:bulk_create'))
return render(request, 'products/bulk_create.html', {'formset': formset})
By the way this is currently the only forum post/thread dealing with
"Django Forms - Inlines and Media" challenges:
3 Answers

james white
78,399 PointsAfter several hours of trying different variations I finally came up with this code that passed the challenge:
from django.core.urlresolvers import reverse
from django.http import HttpResponseRedirect
from django.shortcuts import render
from . import forms
def product_form(request):
form = forms.DigitalProductForm()
if request.method == 'POST':
form = forms.DigitalProductForm(request.POST)
if form.is_valid():
form.save()
return HttpResponseRedirect(reverse('products:create'))
return render(request, 'products/product_form.html', {'form': form})
def bulk_create_products(request):
formset = forms.DigitalFormset()
if request.method == 'POST':
formset = forms.DigitalFormset(request.POST)
if formset.is_valid():
formset.save()
return HttpResponseRedirect(reverse('products:bulk_create'))
return render(request, 'products/bulk_create.html', {'formset': formset})
Note: to Ken...in regard to:
"I know that in the past we have differed in our approach to answering questions,
so if this still causes more confusion.."
You are right Ken. Your approach to answering questions I find distinctly un-helpful.
You answer post failed to point me in the right direction and gave me no insights whatsoever into the proper approach for coding inline formsets.
Your incomplete code snippet only confused things further.
At least Chris took the time to explain (walk me through) things in this thread:
https://teamtreehouse.com/community/inline-formsets-the-outer-edge-of-arcane-django-trivia
My main point (that the original Bummer! about "did you remove it?" is inaccurate) still holds.
The Bummer! error should have just been shortened to:
Bummer! Can't find the formset in the HTML.
... because I never "removed" anything from the original code --only added to it.
Having the Bummer! message add something to "Can't find the formset in the HTML",
like "missing request method", would have been more informative (for debugging purposes) as well.
If only I was asked to QA some of these courses --they would come out vastly differently (including the way the challenge Bummer! answer responses work).
Then again doing QA for one of Instructor Love's courses would probably mean I would have to interact with him or his code more closely than I do now and the thought of that only makes me shudder.
But oh well...got my few measly [semi-meaningless] points, moving on..

Ken Alger
Treehouse TeacherMr. White;
You are missing the check to see, and do something, if the request method is a POST request. Something like:
if request.method == 'POST':
and then set the formset
variable to forms.DigitalFormset(request.POST)
. Then you can do your check to see if formset
is valid or not and reverse the route to bulk_create
.
Hope that points you in the right direction. I know that in the past we have differed in our approach to answering questions, so if this still causes more confusion, post back and I'll try again.
Happy coding,
Ken

james white
78,399 PointsSorry Chris, but despite your thumbs up Ken's answer isn't working for me.
But I'm am getting a different Bummer (so I guess I'm making progress):
Bummer! Didn't find any errors with bad data. Did you check the validity of the formset?
..with this code:
from django.core.urlresolvers import reverse
from django.http import HttpResponseRedirect
from django.shortcuts import render
from . import forms
def product_form(request):
form = forms.DigitalProductForm()
if request.method == 'POST':
form = forms.DigitalProductForm(request.POST)
if form.is_valid():
form.save()
return HttpResponseRedirect(reverse('products:create'))
return render(request, 'products/product_form.html', {'form': form})
def bulk_create_products(request):
formset = forms.DigitalFormset()
if request.method == 'POST':
form = forms.DigitalProductForm(request.POST)
if form.is_valid():
form.save()
return HttpResponseRedirect(reverse('products:create'))
return render(request, 'products/bulk_create.html', {'formset': formset})
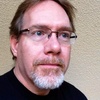
Chris Freeman
Treehouse Moderator 68,441 PointsHi James, Sorry this challenge was frustrating. My thumbs up was to indicate to Ken that I upvoted his answer. (it is an experiment I'm trying to let those posters know I upvoted them). His answer wasn't a complete solution, but it was intended guide in the correct direction, and he did ask for a post back if you his help wasn't sufficient. Which I take, it wasn't.
Good for you for keeping at it and finding the solution.
My moderator experience has been that each of us moderators and Kenneth has our own styles of answering questions on the forum. I personally try to only chime in as the original responder, or if I see something that needs clarification or correction. Since Ken A. was on this one, I had moved on to other questions. There isn't any communication directly between moderators, other than what we see in each others post, to coordinate our efforts. I primarily focus on the Python forum so I don't know if the behavior is different in how other moderators interact outside of Python.
As the 4th highest overall points leader, you certainly have more insight the flow of interactions on the forum pages. If you have suggestions on how the moderator system (or my performance as a moderator) can be improved I encourage you to provide feed back to help@teamtreehouse.com.
-- Chris

Stephen Hutchinson
8,426 PointsYou aren't validating the formset in the above...just the form