Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial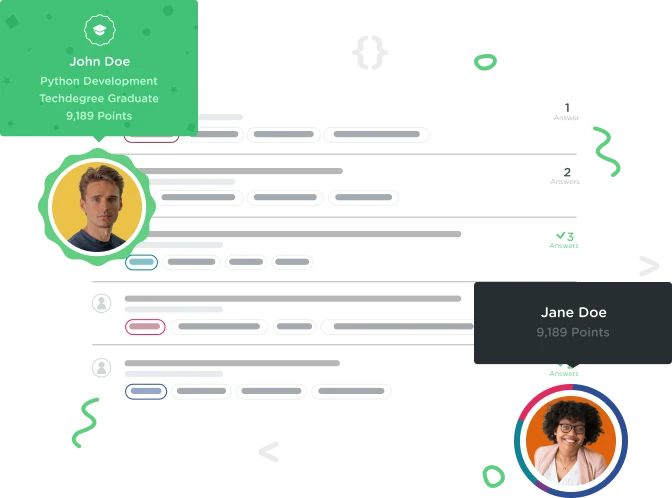

MUZ140515 Herbert Chirwa
2,589 Pointsbummer expected "Meeeeeeeeeeeeeeeeeeeeeeeee" got meeeeeeeeeeeeeeeeeeeeeeee
code won't pass challenge the response l'm getting is confusing me help. thank you
from animal import Animal
class Sheep(Animal):
sound = "meeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee"
def animal_noise(self):
return self.sound.lower()
def Sheep_noise(self):
return self.sound.uppercase()
2 Answers

Andrei Fecioru
15,059 PointsThe last step of that challenge requires you to override the noise()
method in the Animal
base class. You do that by defining a method with the exact same name in the Sheep
class. The code should look like the following:
from animal import Animal
class Sheep(Animal):
sound = "meeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeeee"
# let's re-define the noise method and override the
# implementation from the base class
def noise(self):
# we need to transform the "sound" instance var into it's upper-case version
return self.sound.upper()
Note that in order to transform a string into its uppercased counterpart you need to call the upper()
method on it.
Hope this helps.

Dan Johnson
40,533 PointsIn order to override a method of the base class, it needs to have the same name in the child class:
def noise(self):
return self.sound.upper()

MUZ140515 Herbert Chirwa
2,589 Pointsthank you so much it is now very clear

MUZ140515 Herbert Chirwa
2,589 Pointsthank you so much it is now very clear

MUZ140515 Herbert Chirwa
2,589 Pointsthank you so much it is now very clear
MUZ140515 Herbert Chirwa
2,589 PointsMUZ140515 Herbert Chirwa
2,589 PointsAndrei thank the code passed perfectly fine but l still don't understand why I have to use the name noise not def Sheep_noise(self)
Andrei Fecioru
15,059 PointsAndrei Fecioru
15,059 PointsThis relates to the way class inheritance works. Let's say you have a
Base
class with a method calledboo
and you extend that class into a new class calledDerived
. The inheritance link established between the two classes automatically grants theDerived
class access to theboo
method implemented in theBase
class. So when you callboo
on theDerived
object you will actually invoke theboo
method implemented by theBase
class. This is why it is called inheritance in the first place: The child class gets stuff for free from its parent.However, if you want the
Derived
class to do it's own thing whenboo()
is invoked on it, you need a mechanism to override (and renounce) the implementation provided by the parent class via inheritance. This process is called method overriding. The child class overrides the implementation provided by the parent.The majority of Object Orientation languages (not just Python) implement this feature by allowing the child class to define a method with the exact same name as the method you want to override in the parent class. In other words, we do not define a method called
Derived_boo
like you suggested; you just define a method calledboo
. This will instruct the Python interpreter to discard the implementation of theboo
method provided by theBase
class and use the one provided by theDerived
class instead.Hope this helps.