Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial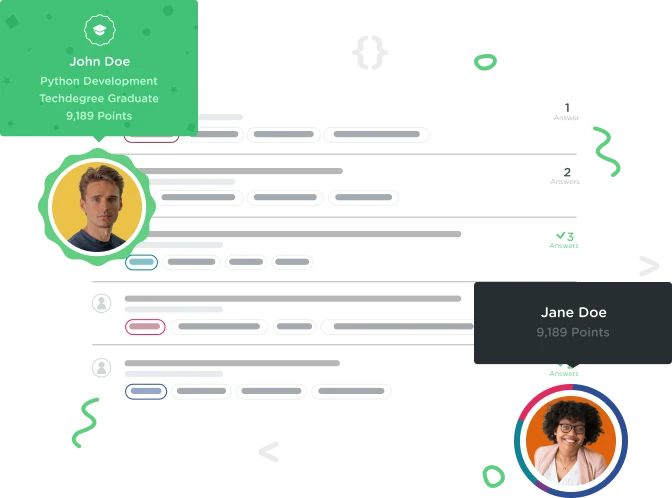

rasul
577 PointsBummer: java.util.NoSuchElementException: No line found (Look around Scanner.java line 1540)
I have a task about checking last name by its first letter, if it's between A-M returns line #1 , but if it's between N-Z returns line #2, so is there any way to check my cod is correct? however it's showing: Bummer: java.util.NoSuchElementException: No line found (Look around Scanner.java line 1540)
import java.util.Scanner;
public class ConferenceRegistrationAssistant {
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char alpha;
Scanner scanner = new Scanner(System.in);
System.out.print("Enter last name: ");
String lastNameInput = scanner.nextLine();
char firstletter = lastNameInput.charAt(0);
for(alpha = 'A'; alpha <= 'N'; alpha++) {
if (alpha == firstletter) {
lineNumber = 1;
} else { lineNumber = 2; }
}
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
2 Answers

rasul
577 PointsOh! I'm just realizing it was so simple, thanks a lot!

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsHello rasul
Great question, I looked at your code and I see you are using a Scanner Object. This should not be the case since you are not taking output from the user. The for-loop is also not needed since you are not looping - if-stament will do just fine.
So here is how I did it. Borrowing from your code, I declared a char alpha and used charAt(0) to get the first letter from the String lastName . I then used an if-statement logic to figure out if the char alpha is less or equal to M , if true the line number is 1 and if false the line number is 2. You really don't have to worry about using 'N' since 'M' is the letter in the middle; so it is either the char alpha be less or be more than M. See my code below:
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
char alpha = lastName.charAt(0);
if (alpha <= 'M') {
lineNumber = 1;
}
else{
lineNumber = 2;
}
return lineNumber;
}
Happy coding bro