Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial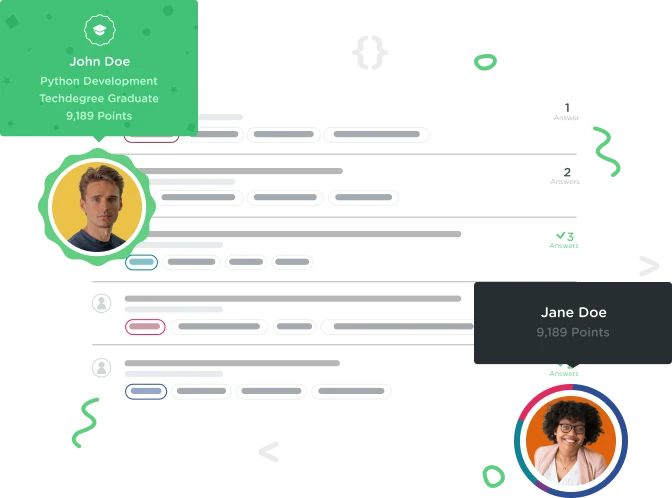

Morgan Strnad
14,292 PointsBummer! No `add` method detected that accepts one parameter of type `Contact` and that returns a `String`
What am I getting wrong on this? My objective is: Annotate the controller's add method so that it captures a POST request to "/contacts", and includes a Contact parameter whose value comes from a form submission. Then, before the return statement, use the autowired ContactService field to save the Contact parameter value.
package com.teamtreehouse.contactmgr.controller;
import com.teamtreehouse.contactmgr.service.ContactService;
import com.teamtreehouse.contactmgr.model.Contact;
import org.springframework.stereotype.Controller;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class ContactController {
@Autowired
private ContactService contactService;
@RequestMapping(value = "/contacts", method = RequestMethod.POST)
public String add() {
contactService.save(contact);
model.addAttribute(contact);
return "redirect:/contacts/" + contact.getId();
}
}
1 Answer
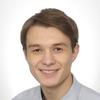
Sergey Podgornyy
20,660 PointsYou forgot to pass parameter to function
@RequestMapping(value = "/contacts", method = RequestMethod.POST)
public String add(Contact contact) {
// TODO: Save the Contact object
contactService.save(contact);
model.addAttribute(contact);
return "redirect:/contacts/" + contact.getId();
}
Chris Jones
Java Web Development Techdegree Graduate 23,933 PointsChris Jones
Java Web Development Techdegree Graduate 23,933 PointsI'm sorry, I don't understand why your method above works. Can you help me understand?
The challenge says "Annotate the controller's add method so that it captures a POST request to '/contacts', and includes a Contact parameter whose value comes from a form submission". How does your method capture the Contact object that comes from the form submission? And where does the Model object come from? I don't see it instantiated in the method or passed into the method's parameter.
Overall, could you just explain how your method works?
Here is what I was trying: