Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial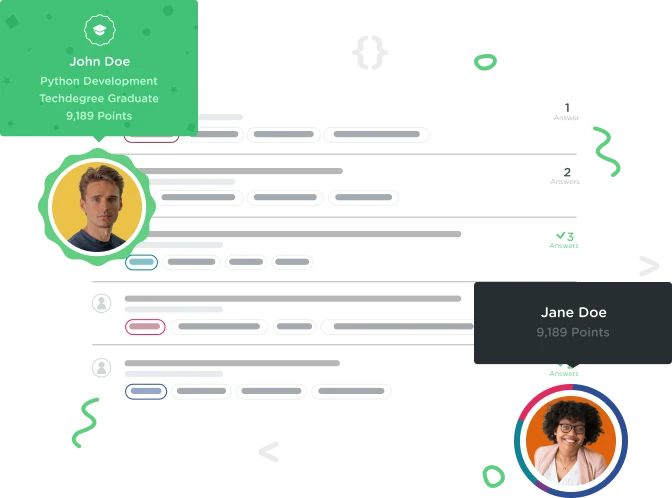

james white
78,399 Points"Bummer! Oh no we got a failure."
I was fiddling around and I got the "failure" Bummer! that I pasted in as the title of this thread
It's a type of Bummer I've never encountered before...but there where no compiler errors noted, though
Code should be attached below.
package com.teamtreehouse.vending;
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
public class CreditorTest {
private Creditor creditor;
@Before
public void setUp() throws Exception {
creditor = new Creditor();
}
@Test
public void addingFundsIncrementsAvailableFunds() throws Exception {
creditor.addFunds(25);
creditor.addFunds(25);
assertEquals(50, creditor.getAvailableFunds());
}
@Test
public void refundingReturnsAllAvailableFunds() throws Exception {
creditor.addFunds(10);
int refund = creditor.refund();
assertEquals(10, refund);
}
@Test
public void refundingResetsAvailableFundsToZero() throws Exception {
creditor.addFunds(10);
creditor.refund();
assertEquals(0, creditor.getAvailableFunds());
}
@Test (expected = NotEnoughFundsException.class)
public void NotEnoughFundsException() throws Exception {
}
}
The Preview for output.html:
FAILURES
--------
NotEnoughFundsException: Expected exception: com.teamtreehouse.vending.NotEnoughFundsException
Note: I've watched the Happy Path and Beyond" video as well as the "Exceptions" video
(around time mark 6:00 of the Exceptions video seemed almost applicable...somewhat...)
but I guess the "failure" is understanding what exactly the challenge is looking for...
3 Answers

james white
78,399 PointsTo anyone else looking at this forum thread...
Out of desperation I tried just adding the following code:
@Test(expected = NotEnoughFundsException.class)
public void deductingMoreMoneyThanAvailableNotAllowed() throws Exception {
creditor.deduct(100);
}
..and it passes the challenge --but don't ask me why though..
(Is '100' more relevant than '99' or '101' ...or even a value '1' somehow?)
Note: This code snippet comes directly from the bottom of the "CreditorTest.java" file
inside '/tests/com/treehouse/vending/' of the "s3v5" folder (after unzipping the zip download associated with the course).
Sorry Ken, but your answer pretty much failed to point me in the right direction.
I ended up figuring it out from pure brute force trial and error.
I can only hope that if more unit testing courses eventually come down the pike
(pass through Trello and the Roadmap)
that a much more significant effort is made to make the challenge's answer analyzer a little more specific in it's debug "hints".

Akshay Ramdasi
14,065 PointsThis is the answer for this exercise: I hope this helps- You can pass any number (I have passed 300), but remember it should be greater than amount you have previously added by using creditor.addFunds() method I have passed this method 2 times like this
creditor.addFunds(25);
creditor.addFunds(25);
So now the funds now is 25+25 = 50 (that is why I have passed 300 [300> 50] ) in order to pass the test.
@Test (expected = NotEnoughFundsException.class)
public void NotEnoughFundsException() throws Exception {
creditor.deduct(300);
}
```

Ken Alger
Treehouse TeacherJames;
Your syntax setup looks great, you just need to do something inside NotEnoughFundsException()
. The directions indicate that we should be looking at the deduct
method over in the Creditor
class, right? Perhaps we should be checking to see what happens if we deduct
more funds than we have available? That sounds like a reasonable thing to test, yes?
Since each test starts out with a zero balance, i.e. we start the test assuming we have not added any funds, if we do a deduct
in any amount we should get a balance less than zero, or for the sake of this course's topic, the vending machine would wind up owing us money. That would be great but I don't think Tex would like it much and we probably won't be doing much more work for him or his company.
Hope that points you in the right direction.
Happy coding,
Ken

james white
78,399 PointsHi Ken, I'm afraid your hopes are dashed.
It definitely did not point me (fully) in the right direction.
I know that there has to be something inside NotEnoughFundsException(), but I'm totally unclear what that something might be...
Looking at the zip download for the course (and specifically the code in tests/com/treehouse/vending/ of the "s3v5" folder),
I see inside "CreditorTest.java" lines like:
creditor.refund();
creditor.addFunds(10);
...where 'creditor' was assigned in the @Before section:
private Creditor creditor;
@Before
public void setUp() throws Exception {
creditor = new Creditor();
}
Checking out "AlphaNumericChooserTest.java" I see lines like:
chooser.locationFromInput("WRONG");
chooser.locationFromInput("B52");
private AlphaNumericChooser chooser;
...where 'chooser' was assign in the @Before section:
@Before
public void setUp() throws Exception {
chooser = new AlphaNumericChooser(26, 10);
}
So what I'm guessing is that the use of this something.something pattern is what is needed.
Even though we are both from Oregon, we are different in that I am very "pattern-driven".
I need to see the pattern before I can relate it to any kind of understanding of what's going on..
So I noticed (specifically) in the challenge the following code:
private Creditor creditor;
@Before
public void setUp() throws Exception {
creditor = new Creditor();
}
...which implies that what might be needed is "creditor.something".
Maybe it should be "creditor.deduct();" --based on what you were trying to explain..?
But when I try that:
@Test (expected = NotEnoughFundsException.class)
public void NotEnoughFundsException() throws Exception {
creditor.deduct();
}
It gives me a compiler error:
./com/teamtreehouse/vending/CreditorTest.java:46: error: method deduct in class Creditor cannot be applied to given types;
creditor.deduct();
^
required: int
found: no arguments
reason: actual and formal argument lists differ in length
1 error
...that implies some number ('int') is needed, but what argument is it looking for?
I even tried crazy code like:
@Test (expected = NotEnoughFundsException.class)
public void NotEnoughFundsException() throws Exception {
int deduct = creditor.deduct();
assertEquals(deduct, creditor.getAvailableFunds());
}
...or code like (to avoid the int compile error):
@Test (expected = NotEnoughFundsException.class)
public void NotEnoughFundsException() throws Exception {
int refund = creditor.refund();
assertEquals(refund, creditor.getAvailableFunds());
}
But in the end I either end up fighting compile errors or Bummer! we got a problem errors.
The treehouse answer analyzer is just being too non-specific about what is needed...(what it wants that would even get me in range of the type of code that's expected).

Ken Alger
Treehouse TeacherJames,
Sorry you didn't find my answer helpful. In looking at the method signature for deduct
in Creditor.java
it states that it takes an argument of int money
. Given that I felt, and still feel, that my answer would lead people to the same conclusion you game to through "brute force trial and error."
Happy coding,
Ken