Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial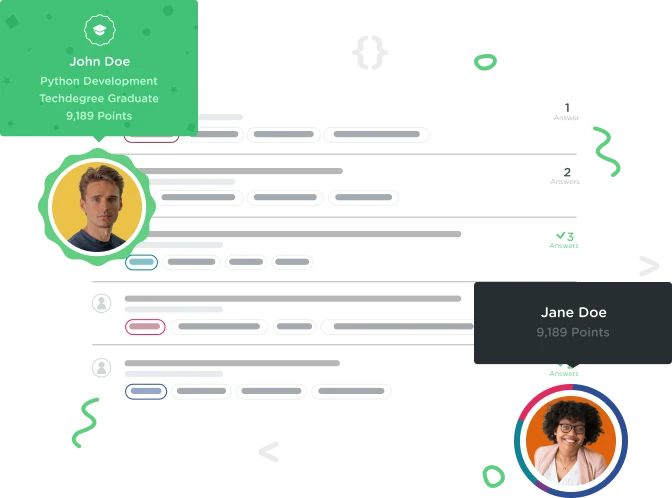
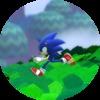
ilya A
3,802 PointsBummer: The `document.write()` method was called 27 times. It should be called 26 times.
I think that this code will pass if I simply change "26" to "25," but I would like to know the correct way of passing this challenge.
var count = 0;
while (count <= 26) {
document.write("loop has run" + count + "times")
count += 1;
}
3 Answers

Ryan Lovett
5,100 PointsTry thinking of it like this, the script is running while count <= 26. In that case, when the count is set to 0 and even though 0 represents nothing, it still meets the conditions of the while loop, which is why the script still runs for 0. So then, in that case the script is actually running 27 times if you include the time it runs for 0 plus the 26 you defined as a condition.
Hope that clears it up a bit, it is tricky to think about. :)

Ryan Lovett
5,100 PointsChanging to 25 is actually the correct way, you're on the right track! Think about what is happening here, you are setting the count to 0 and running until count <= (less the OR equal to) 26, so really you are telling it to run 27 times when you include the 0.
So if you want it to run 26 times, setting it to 25 will do just that since you are starting from 0 and 0 is included as one of those times. If you were starting from 1 then the current way you have it would work. Don't worry, this tends to stump a lot of people.
Hope this helps!
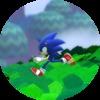
ilya A
3,802 PointsThat was helpful, thank you. But I find it strange how zero counts as 1
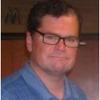
Mark Wilkowske
Courses Plus Student 18,131 PointsWhen count first enters the while loop it is 0 and less than 26 so it prints out.
In JavaScript, think of variables as occupying a space in browser memory.
var count = 0; this literally means: we are defining a space in memory called 'count' and at this time zero is in 'count'. This is not saying 'nothing' is in count. During the loop count changes until a limit is reached when the loop stops.
(count < 26) passes the challenge.
(count <= 25) - passes the challenge as well but there's an important reason not to go that route - I need to brush up more.
You can console.log both versions and see but first add a ; to the end of your document.write line.
ilya A
3,802 Pointsilya A
3,802 PointsThat makes perfect sense! Thank you. Now I am curious... Would it be possible to somehow use the number 26, and have it only run 26 times? (perhaps use a different equation?)
Ryan Lovett
5,100 PointsRyan Lovett
5,100 PointsNo problem, i'm glad that made more sense! To use the number 26, you would just have to set the count variable to 1 instead of 0 when you define it. Then it is running from 1 to 26 instead of 0 to 26, hope that helps!