Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial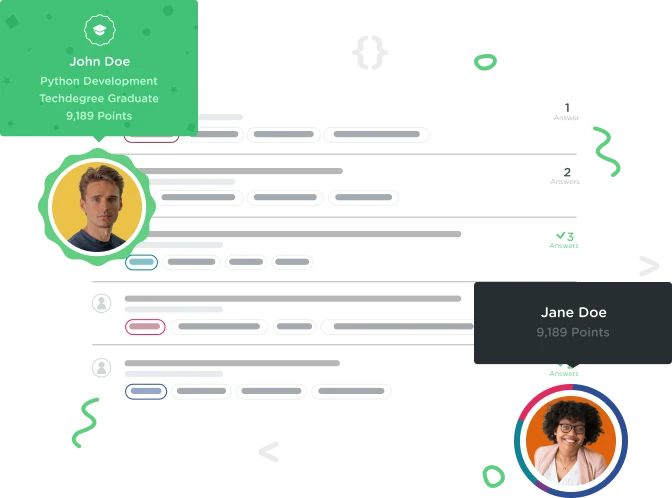
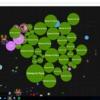
Noah Kilker
1,224 PointsBummer! Try again!
I have run multiple tests on the code I have wrote for this assignment, and have done all of what the question seems to ask. I think that I am misreading the question, as all I can get from the built in error message is "Bummer! Try again". I think i'm doing everything the question asks, correct me if i'm wrong. But seeing as i cant find anything wrong with my code, and the only error message it gives doesn't tell me whats wrong, i have no where else to go but the 'Get Help' button.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(mystring):
lowered = mystring.lower()
mystringParts = lowered.split(" ")
thisdict = {}
for s in mystringParts:
try:
thisdict.update({s : (thisdict[s] + 1)})
except KeyError:
thisdict.update({s : 1})
del thisdict[""]
return thisdict
1 Answer
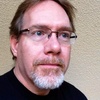
Chris Freeman
Treehouse Moderator 68,423 PointsYou are very close!
-
split on WHITESPACE instead of a literal space. Whitespace is the default with no argument.
-
remove
del
statement
Post back if you need more help. Good luck!!!
Noah Kilker
1,224 PointsNoah Kilker
1,224 PointsThanks for the help, I really appreciate it, this question really had me stumped. I did what you told me to do, and I think I understand why it works (less code, easier to read/write/understand), but I don't entirely understand why the old version didn't work, because as far as I can tell, the output is the same. There is obviously some difference, but I cant find one. I'd appreciate if you could explain the difference in the output, or the reasoning. If for no other reason than if I need to do something like this in the future and want to have all of the information.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherNoah Kilker my guess here is that you've tried it, but not with a data set that would give you a different result. The challenge says to split on all white space. But you were splitting on spaces. My guess is that you weren't trying with a data set that included tabs or new line characters. In that case, it wouldn't split on the tabs and new lines with your previous code. But by changing the
split()
to have no arguments, it now takes into account all whitespace. Not just spacesChris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsGiven the two test cases below, the difference is clearer. Note that deleting the empty key "" raises an error of that empty string key is not found.
Produces: