Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial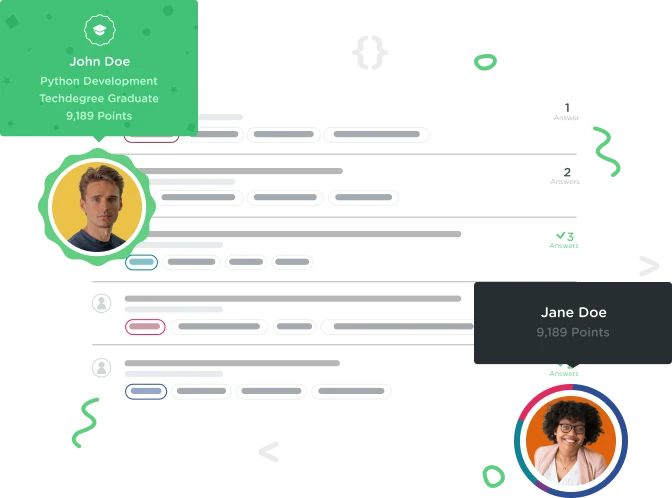

Gokul Nishanth
Courses Plus Student 581 PointsBummer Try again!
Great! Let's make one more scoring method! Create a score_yatzy method. If there are five dice with the same value, return 50. Otherwise, return 0.
from hands import YatzyHand as yh
class YatzyScoresheet:
def score_ones(self, hand):
return sum(hand.ones)
def _score_set(self, hand, set_size):
scores = [0]
for worth, count in hand._sets.items():
if count == set_size:
scores.append(worth*set_size)
return max(scores)
def score_one_pair(self, hand):
return self._score_set(hand, 2)
def score_chance(self, hand):
return sum(hand)
def score_yatzy(self):
if len(yh._by_value(1)) == 5:
return 50
if len(yh._by_value(2)) == 5:
return 50
if len(yh._by_value(3)) == 5:
return 50
if len(yh._by_value(4)) == 5:
return 50
if len(yh._by_value(5)) == 5:
return 50
if len(yh._by_value(6)) == 5:
return 50
else:
return 0
4 Answers
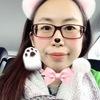
renhe
10,282 PointsHere's another way to approach it:
def score_yatzy(self, hand):
if len(set(hand)) == 1:
return 50
else:
return 0
If all five dice have the same value, then the length of the set should be one as there is only a single unique value.
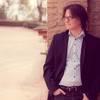
Michal Janek
Front End Web Development Techdegree Graduate 30,654 Pointsdef score_yatzy(self,hand):
if self._score_set(hand, 5):
return 50
else:
return 0
Meaning: If the function self._score_set(hand,5) returns True or any sort of Truthy data it means there are 5 of the same in the hand - therefore the if conditions returns 50. Otherwise it returns 0.

toirleachomalley
11,979 PointsI just did a fairly lazy compare between each item in the hand list.
if hand[0] == hand [1] and hand[1] == hand[2] and hand[2] == hand[3] and hand[3] == hand[4]: return 50 else: return 0
That seemed to do the trick. Not very elegant and I'm sure you could enumerate through the list and then return zero the first time you get an unequal item (not equal to the last item you checked), otherwise return 50. It would be much more flexible for longer lists but I'll leave that up to you.

J llama
12,631 Pointsdef score(hand): if hand[1:] == hand[:-1]: print(50) else: print(0)
score([1,1,1,1,1]) #to test
................................... simplified for you

Christian Scherer
2,989 PointsWould like to know this one as well. Do we really need to import sth from Yatzyhand?
rhupp
11,019 Pointsrhupp
11,019 PointsVery clever, @renhe. It looks like the solution was mainly a matter of logic. Thanks for this; I was definitely overthinking things. :)
Marcus Schumacher
16,616 PointsMarcus Schumacher
16,616 PointsWhy isn't my code here working?