Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial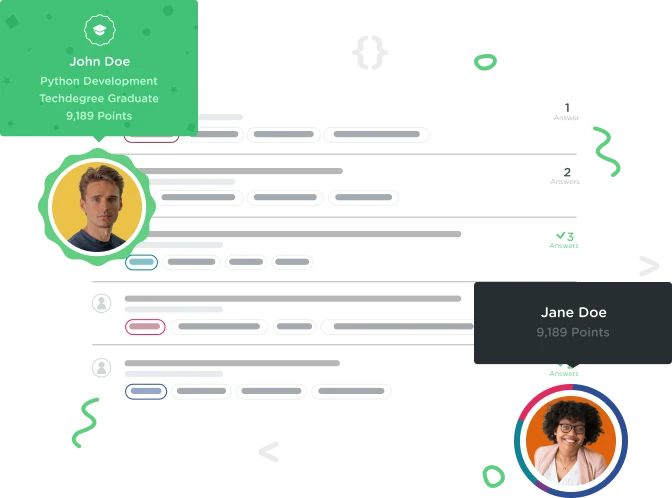
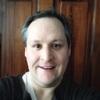
givens
7,484 PointsBummer Try Again, but it works in Spyder
I tested the morse.py code in Spyder. The correct output was when I typed:
s = S()
t = str(s)
v = Letter.from_string(t)
I tried this for combinations of dashes and dots. I pasted this code to Treehouse. I "rechecked work." I get Bummer Try Again! Not sure why when it works outside Treehouse.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls, morse_str):
morse_list = morse_str.split('-')
morse_list2 = []
for item in morse_list:
if item=="dot":
morse_list2.append('.')
else:
morse_list2.append('-')
return morse_list2
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
2 Answers
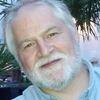
Jeff Muday
Treehouse Moderator 28,717 PointsYou got it correct, but with a little caveat... one of the confusing things about this challenge is that they are using underscores on the class method. So there are two little changes to make it pass the grader:
- replace the '-' with an underscore when you append to morse_list2
- since this is a class method, we need to use cls(morse_list2) as the return value.
@classmethod
def from_string(cls, morse_str):
morse_list = morse_str.split('-')
morse_list2 = []
for item in morse_list:
if item=="dot":
morse_list2.append('.')
else:
morse_list2.append('_') # 1. change to underscore
return cls(morse_list2) # 2. change to return as cls (class) method.
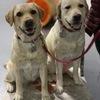
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi B G,
You have a couple of issues. First, you are using -
in your class method but the instructions tell you to use underscores:
takes a string like "dash-dot" and creates an instance with the correct pattern (['_', '.']).
Second, you are returning a list (return morse_list2
), but you are supposed to create a new instance of the class:
takes a string like "dash-dot" and creates an instance with the correct pattern (['_', '.']).
Hope that points you in the right direction
Cheers
Alex
Jeff Muday
Treehouse Moderator 28,717 PointsJeff Muday
Treehouse Moderator 28,717 Pointssorry... I was working on the answer and Alex Koumparos beat me to the punch! Enjoy Python!