Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial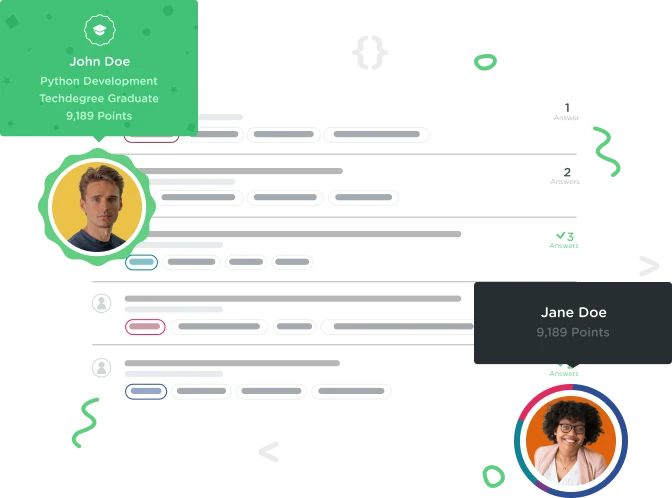

liamthornback2
9,618 Points"Bummer: We tried your spec with a version of subtraction.js that DOESN'T work correctly, expecting the test to fail..."
Not sure why my test would pass when it shouldn't. My code here looks almost exactly like the code I wrote for the project...
const expect = require('chai').expect
describe('subtraction', function() {
const subtraction = require('../WHEREVER')
it('only works with numbers', function() {
const handler = () => subtraction(null, {});
expect(handler).to.throw(Error);
expect(handler).to.throw('subtraction only works with numbers!');
});
});
function subtraction (number1, number2) {
if (typeof number1 !== 'number' || typeof number2 !== 'number') {
throw Error('subtraction only works with numbers!')
}
return number1 - number2
}
UPDATE:
So apparently I did two things wrong:
1.) I didn't export the subtraction function. It never occured to me to do so because I wasn't being tested on exporting functions. Seems pretty stupid but okay.
2.) I used an arrow function. I don't see what the problem is with writing my handler as an arrow function. Does anyone have any idea why treehouse would want the handler function to be written in the regular syntax?
2 Answers

Mike Francois
11,438 Pointsvar expect = require('chai').expect
describe('subtraction', function () {
var subtraction = require('../WHEREVER')
it('only works with numbers', function () {
var handler = function (){subtraction('string1', 'string2');};
expect(handler).to.throw(Error);
})
})
This worked for me, hope it helps.
I think the arrow function should be written as:
const handler = () => { subtraction(null, {}); };
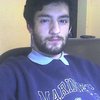
Dario Bahena
10,697 PointsIf you read the documentation, mocha does not like arrow functions. Although I am not quite sure why it would not work within its own context... It might be due to how 'this' is treated in arrow function expressions and regular function expressions. Regardless, I don't see a reason why this shouldn't work.
const handler = () => subtraction('s','a');
liamthornback2
9,618 Pointsliamthornback2
9,618 PointsI tried writing my arrow function like that before and it didn't work.
Thanks for the reply though.