Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial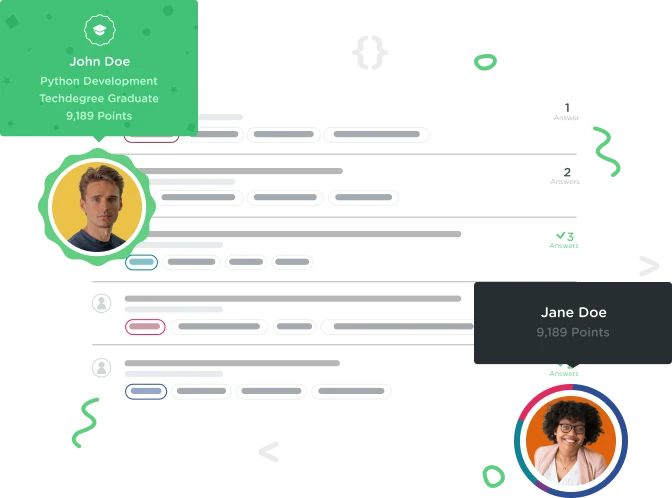

Shivam Barthwal
2,365 PointsBummer@Code ChallengeTask 2: Try returning the result of the expression
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
private String match;
private String unMatch;
public ScrabblePlayer() {
tiles = "";
match = "";
unMatch = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
// TODO: Add the tile to tiles
tiles += tile;
}
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
boolean isTile = tiles.indexOf(tile) != -1;
if (isTile) {
match += tile;
} else { unMatch += tile;
}
return isTile;
}
}
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
private String match;
private String unMatch;
public ScrabblePlayer() {
tiles = "";
match = "";
unMatch = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
// TODO: Add the tile to tiles
tiles += tile;
}
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
boolean isTile = tiles.indexOf(tile) != -1;
if (isTile) {
match += tile;
} else { unMatch += tile;
}
return isTile;
}
}
1 Answer

Yanuar Prakoso
15,196 PointsHi Shivam
The challenge just want you NOT to use the if statement. Instead you just have to directly use return statement to return value true or false. Keep in mind the .indexOf(character char) method on a String will automatically returning a boolean result. Therefore you do not need to declaring boolean on it. Here is how I complete the challenge:
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
return (tiles.indexOf(tile) > -1);
}
as you have known if tiles.indexOf(tile) returns -1 meaning the tile is not passed which will resulting false boolean result. How you gain true boolean result? Of course by stating tiles.indexOf(tile) > -1 meaning if the tile is there it will returns true. Or you can also use tiles.indexOf(tile) != -1 I just lazy enough to type ! and = because it was located too far apart. Sorry about that....
I hope this will help you a little.
Shivam Barthwal
2,365 PointsShivam Barthwal
2,365 PointsHi Yanuar,
Little late in responding. I already figured out of my own. Anyways, really appreciate your response:)