Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial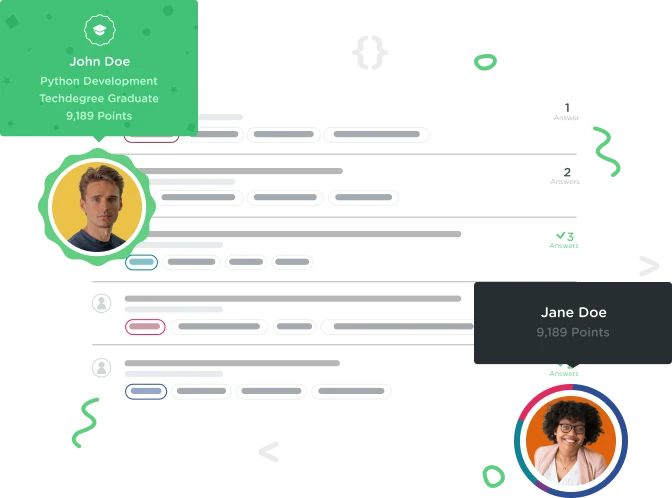
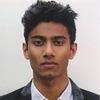
Samuel Kleos
Front End Web Development Techdegree Student 13,728 PointsBut with .then() you can't pass values as arguments into callbacks like you can with nested callbacks?
See the following code where I've written nested callbacks with continuation-passing style as an alternative to the chained mathPromise.then() methods in the instruction.
function calculate(x, callback) {
callback(x);
}
function add(x, y, callback) {
callback(x + y)
}
function finalValue(nextValue) {
console.log("The final value is ${nextValue}")
}
// Using nested callbacks to pass n
calculate(5, (n) => { // calculate passes callback with n param executing add()
// n param becomes input x and gets added 10
add(n, 10, (n) => { // add passes callback with n param executing subtract()
subtract(n, 2, (n) => {
multiply(n, 5, (n) => {
finalValue(n) ;
});
});
});
});
1 Answer
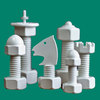
Steven Parker
231,275 PointsNote that your example is not an alternative to the lesson code as it contains no promises or async operations.
But you can pass values using promises and then by currying the callbacks to include the extra arguments:
const add = (x, y) => x + y;
const subtract = (x, y) => y - x;
const multiply = (x, y) => x * y;
const finalValue = nextValue => console.log(`The final value is ${nextValue}`);
const value = 5;
const mathPromise = new Promise( resolve => { resolve(value); } );
mathPromise
.then(add.bind(this, 10))
.then(subtract.bind(this, 2))
.then(multiply.bind(this, 5))
.then(finalValue);
// The final value is 65
Samuel Kleos
Front End Web Development Techdegree Student 13,728 PointsSamuel Kleos
Front End Web Development Techdegree Student 13,728 PointsFascinating!