Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial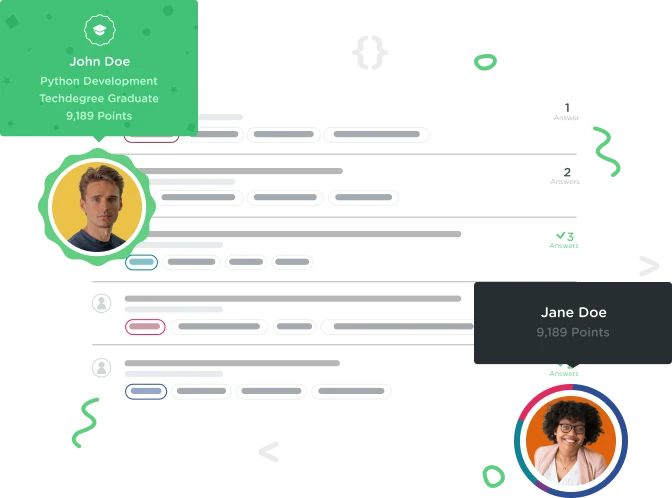
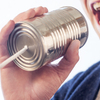
Brian Workman
10,519 PointsButterknife
I am having trouble with ButterKnife. I found that butterknife doesn't use inject anymore so I refactored my code to use @Bind but still cannot seem to find where the issue is. I want to butterknifes github and dug through the docs and tried to find if I was missing something but still nothing.
import android.content.Context;
import android.graphics.drawable.Drawable;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.Bundle;
import android.support.v4.content.ContextCompat;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import com.google.android.gms.common.api.GoogleApiClient;
import com.squareup.okhttp.Call;
import com.squareup.okhttp.Callback;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
import butterknife.Bind;
import butterknife.ButterKnife;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
public class MainActivity extends AppCompatActivity {
private static final String TAG = MainActivity.class.getSimpleName();
private GoogleApiClient mGoogleApiClient;
private CurrentWeather mCurrentWeather;
@Bind(R.id.timeLabel) TextView mTimeLabel;
@Bind(R.id.temperatureLabel) TextView mTemperatureLabel;
@Bind(R.id.humidityLabel) TextView mHumidityValue;
@Bind(R.id.precipLabel) TextView mPrecipValue;
@Bind(R.id.summaryLabel) TextView mSummaryLabel;
@Bind(R.id.iconImageView) ImageView mIconImageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.bind(this);
String apiKey = "584ab0a45b0194bc8e253ed0006aadff";
double latitude = 40.231327;
double longitude = -82.449925;
String forecastUrl = "https://api.forecast.io/forecast/"+apiKey+"/"+latitude+","+longitude;
if(isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Request request, IOException e) {
}
@Override
public void onResponse(Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alertUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "Exception caought: ", e);
}
catch (JSONException e) {
Log.e(TAG, "Exception caought: ", e);
}
}
});
Log.d(TAG, "MAIN UI code is running!");
} else {
Toast.makeText(this, getString(R.string.network_unavaliable),
Toast.LENGTH_LONG).show();
}
}
private void updateDisplay() {
mTemperatureLabel.setText(mCurrentWeather.getTemerature()+"");
mTimeLabel.setText("At "+mCurrentWeather.getFormattedTime()+" it will be");
mHumidityValue.setText(mCurrentWeather.getHumidity() + "");
mPrecipValue.setText(mCurrentWeather.getPercipChance() + "%");
mSummaryLabel.setText(mCurrentWeather.getPercipChance());
Drawable drawable = ContextCompat.getDrawable(this, mCurrentWeather.getIconId());
mIconImageView.setImageDrawable(drawable);
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "FROM JSON: " + timezone);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPercipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemerature(currently.getDouble("temperature"));
currentWeather.setTimeZone(timezone);
Log.i(TAG, currentWeather.getFormattedTime());
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if(networkInfo != null && networkInfo.isConnected()) {
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
3 Answers

Seth Kroger
56,413 PointsWhen you created the MainActivity did you use the Blank Activity (which is anything but blank these days) or Empty Activity? Blank splits the layout into two files with second <include>-ed in the first and ButterKnife has an issue with that. https://github.com/JakeWharton/butterknife/issues/393
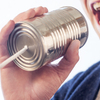
Brian Workman
10,519 PointsI think I found the problem.
In my updateDisplay() I forgot to include the empty string to convert the value returned from getPercipChance(). Changed the line to mSummaryLabel.setText(mCurrentWeather.getPercipChance()+""); and it seems to be working now. I am not sure why I can't see the errors like the video shows or else I could have found this a lot easier. The only error I get is the one I pasted above. I tried looking around in the different panes but can't seem to find it.
This is really fun stuff and I feel like I understand it but have no clue where to start on my own project. This is probably typical learning java, at least I hope so. :) Thanks for your help.
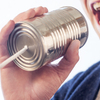
Brian Workman
10,519 PointsWell I think I was wrong above, I do have two layouts, and when I copied one and pasted into the other that must have fixed it. I didn't figure it out until I went on to the next lesson and changed something in the view and it happened again. Can I delete one of the views or how do you stop this from happening? Why did it happen?
Brian Workman
10,519 PointsBrian Workman
10,519 PointsErrors:
11-14 14:05:12.376 9026-9026/example.com.stormy E/AndroidRuntime: Caused by: java.lang.RuntimeException: Unable to bind views for example.com.stormy.MainActivity 11-14 14:05:12.376 9026-9026/example.com.stormy E/AndroidRuntime: at example.com.stormy.MainActivity.onCreate(MainActivity.java:53) 11-14 14:05:12.376 9026-9026/example.com.stormy E/AndroidRuntime: at example.com.stormy.MainActivity$$ViewBinder.bind(MainActivity$$ViewBinder.java:20) 11-14 14:05:12.376 9026-9026/example.com.stormy E/AndroidRuntime: at example.com.stormy.MainActivity$$ViewBinder.bind(MainActivity$$ViewBinder.java:8) 11-14 14:05:12.376 9026-9026/example.com.stormy E/AndroidRuntime: at example.com.stormy.MainActivity.onCreate(MainActivity.java:53)