Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial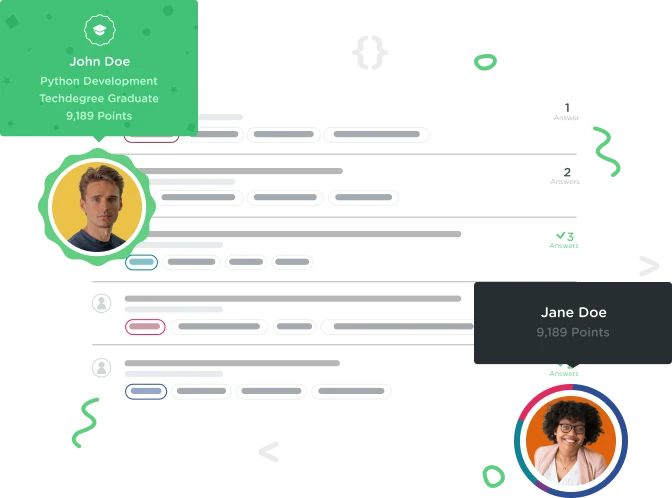
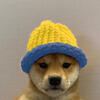
himar
7,420 PointsButton in android won't work
Im making the "Build a simple android app" project, Im in the "Coding Fun Facts" stage.
The problem is that when I run the app, the button "Show another fun fact" won't work, its supposed to give me a random fact. If you need me to give you any of my code, just tell me and I will.
Thanks.
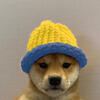
himar
7,420 PointsThe title is "Introduction to arrays". Here is the code:
package com.himarlabs.funfacts;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// Declare our View variables and assign the View from the layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
// The button was clicked, so update the fact label with a new fact
String fact = "";
// Randomly select a fact
Random randomGenerator = new Random(); // Construct a new Random number generator
int randomNumber = randomGenerator.nextInt(3);
/* Convert randomNumber to a text fact
* 0 = Ants stretch when they wake up in the morning.
* 1 = Ostriches can run faster than horses.
* 2 = Olympic gold medals are actually made mostly of silver.
*/
// if randomNumber equals 0 then
if (randomNumber == 0) {
// set fact equal to ants fact
fact = "Ants stretch when they wake up in the morning.";
}
else if (randomNumber == 1) {
fact = "Ostriches can run faster than horses.";
}
else if (randomNumber == 2) {
fact = "Olympic gold medals are actually made mostly of silver.";
}
else {
fact = "Sorry, there was an error!";
}
}
};
// if randomNumber equals 1 then
// set fact equal to ostriches fact
// if randomNumber equal 2 then
// set fact equal to olympic fact
// Update the label with our dynamic fact
showFactButton.setOnClickListener(listener);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.fun_facts, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
2 Answers
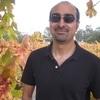
Kourosh Raeen
23,733 PointsThe problem is that you are not updating the factLabel TextView. You need to do that after the if statement where you assign a fact to the fact variable based on the value of the randomNumber:
// if randomNumber equals 0 then
if (randomNumber == 0) {
// set fact equal to ants fact
fact = "Ants stretch when they wake up in the morning.";
}
else if (randomNumber == 1) {
fact = "Ostriches can run faster than horses.";
}
else if (randomNumber == 2) {
fact = "Olympic gold medals are actually made mostly of silver.";
}
else {
fact = "Sorry, there was an error!";
}
// Update the label with our dynamic fact
factLabel.setText(fact);
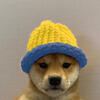
himar
7,420 PointsThank you so much! It works!
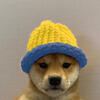
himar
7,420 PointsAnd here is the .xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
tools:context=".FunFactsActivity"
android:background="#ff51b46d">
<TextView
android:text="Did you know?"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/TextView"
android:textSize="24sp"
android:textColor="#80ffffff" />
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Ants stretch when they wake up in the morning."
android:id="@+id/factTextView"
android:layout_centerVertical="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:textSize="24sp"
android:textColor="@android:color/white" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Show another fun fact"
android:id="@+id/showFactButton"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:background="#ffffffff" />
</RelativeLayout>
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsWhat is the title of the video you're on? Also, can you post your code?