Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial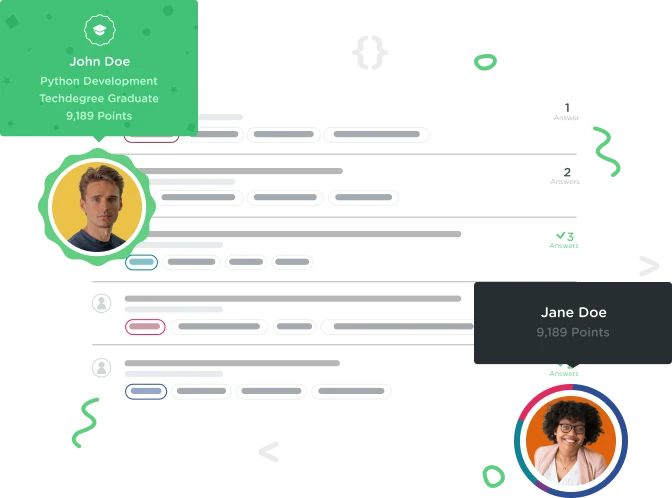
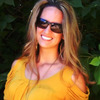
Lindsey Whitney
9,699 PointsButtons don't work
Everything seems to be displaying correctly, but the buttons aren't working. I've re-watched the video to try to catch my error, but can't find it. On a related note, it would be great to have access to the completed files done by the instructor. It would be easier to find your mistakes, and would make great reference material.
Thanks, Lindsey
2 Answers

alexlitel
25,059 PointsLindsey Whitney There appears to be a few errors in your toHTML
prototype. You forgot to include the start of an li
tag at the beginning of your function and close it in quotes. Additionally, you accidentally capitalized the "l" in playing
in this.isPlaying
and forgot the semicolon after htmlString += ' - '
.
This is what the code should look like.
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isPlaying) {
htmlString += 'class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - ';
htmlString += this.artist;
htmlString += '<span class="duration">';
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
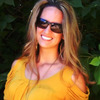
Lindsey Whitney
9,699 PointsThank you!!! I actually did have the start of the li tag in my workspace — but it didn't paste for some reason (or maybe I managed to accidentally delete a few characters). But fixing the last two things you mentioned fixed it! Amazing how you can proof read and still miss things like that :p
Lindsey Whitney
9,699 PointsLindsey Whitney
9,699 PointsI thought it would pull in my code automatically, but I guess I have to paste it all in? Here goes...