Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial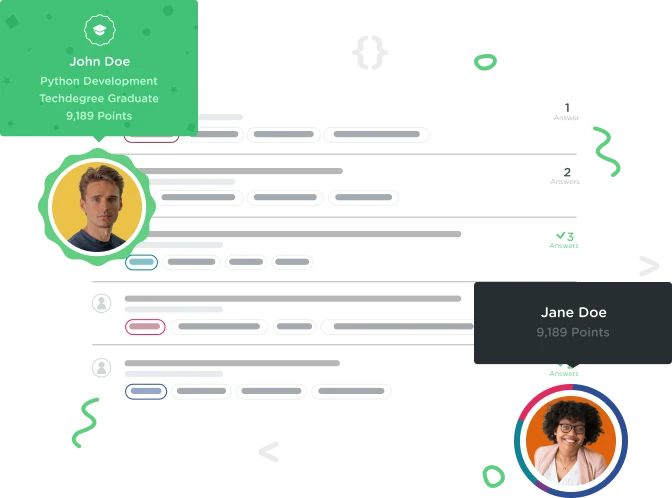
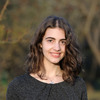
Larisa Popescu
20,242 PointsButtons don't work. I get an error saying that "Actions must be plain objects. Use custom middleware for async actions".
JavaScript > Building Applications with React and Redux > Putting it all Together > Updating the Player, Counter and AddPlayerForm Components
Larisa Popescu
20,242 PointsLarisa Popescu
20,242 PointsThe problem is that every time I press the + or - button from the app, I get this error: " createStore.js:165 Uncaught Error: Actions must be plain objects. Use custom middleware for async actions. at Object.dispatch [as updatePlayerScore] (createStore.js:165) at onClick (Counter.js?0f76:14) at HTMLUnknownElement.boundFunc (ReactErrorUtils.js:63) at Object.ReactErrorUtils.invokeGuardedCallback (ReactErrorUtils.js:69) at executeDispatch (EventPluginUtils.js:83) at Object.executeDispatchesInOrder (EventPluginUtils.js:106) at executeDispatchesAndRelease (EventPluginHub.js:41) at executeDispatchesAndReleaseTopLevel (EventPluginHub.js:52) at Array.forEach (<anonymous>) at forEachAccumulated (forEachAccumulated.js:22) "
This also happens when I want to remove a Player. But the add player button works just fine. I reviewed every video but I couldn't get this error.
Scoreboard.js
actiontypes/player.js
actions/player.js
reducers/player.js
Player.js
Counter.js
AddPlayerForm.js
index.js