Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial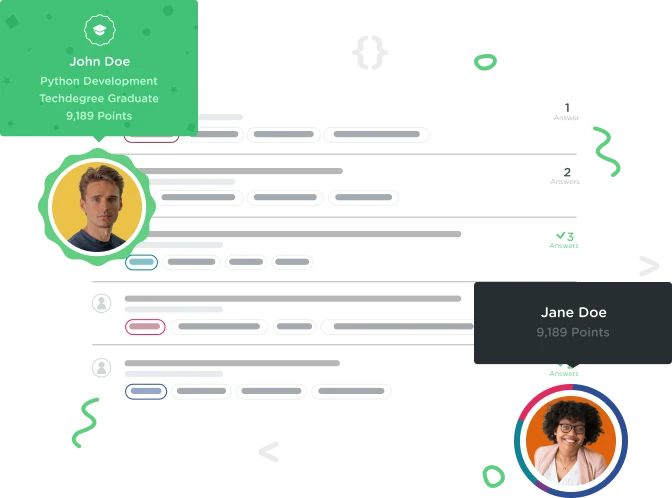
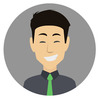
Dan Arata
9,253 PointsButtons readd duplicate songs to playlist.
I can see that my code is working. The only problem is that when I hit play, next, or stop, I'm getting an added list to my playlist.
I'm sure that I have the code down, I've rewatched the video and started from scratch twice yet I'm still producing the same result.
I'm pretty sure the problem is coming from the refresh on 'playlist.renderInElement(playlistElement);' but I'm not sure why
Here is my code:
app.js
var playlist = new Playlist();
var constantHeadache = new Song("Constant Headache", "Joyce Manor", "2:59");
var christmasCard = new Song("Christmas Card", "Joyce Manor", "3:23");
playlist.add(constantHeadache);
playlist.add(christmasCard);
var playlistElement = document.getElementById("playlist");
playlist.renderInElement(playlistElement);
var playButton = document.getElementById("play");
playButton.onclick = function(){
playlist.play();
playlist.renderInElement(playlistElement);
}
var nextButton = document.getElementById("next");
nextButton.onclick = function(){
playlist.next();
playlist.renderInElement(playlistElement);
}
var stopButton = document.getElementById("stop");
stopButton.onclick = function(){
playlist.stop();
playlist.renderInElement(playlistElement);
}
song.js
function Song(title, artist, duration) {
this.title = title;
this.artist = artist;
this.duration = duration;
this.isPlaying = false;
}
Song.prototype.play = function() {
this.isPlaying = true;
};
Song.prototype.stop = function() {
this.isPlaying = false;
};
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isPlaying){
htmlString += ' class="current"';
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - ' ;
htmlString += this.artist;
htmlString += '<span class="duration">';
htmlString += this.duration;
htmlString += '</span></li>';
return htmlString;
};
playlist.js
function Playlist() {
this.songs = [];
this.nowPlayingIndex = 0;
}
Playlist.prototype.add = function(songs) {
this.songs.push(songs);
};
Playlist.prototype.play = function() {
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.play();
};
Playlist.prototype.stop = function(){
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.stop();
};
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if(this.nowPlayingIndex === this.songs.length){
this.nowPlayingIndex = 0;
}
this.play()
};
Playlist.prototype.renderInElement = function(list) {
list.innterHTML = "";
for(var i = 0; i < this.songs.length; i++){
list.innerHTML += this.songs[i].toHTML();
}
};
1 Answer
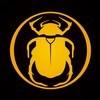
rydavim
18,814 PointsLooks like there's just a minor typo in your renderInElement function that made it so your innerHTML wasn't being reset, so it was adding to it every time a button called the rendering function.
Fixed the typo and it worked great for me, nice job!
list.innterHTML = ""; // Make sure this reads 'inner' in your function and you should be good to go!
Dan Arata
9,253 PointsDan Arata
9,253 PointsSilly mistake on my part! But thank you very much!
rydavim
18,814 Pointsrydavim
18,814 PointsPersonally, I find it much easier to find typos in other people's code than in my own. When it's mine, my brain just reads what I meant to type instead. Happy coding!